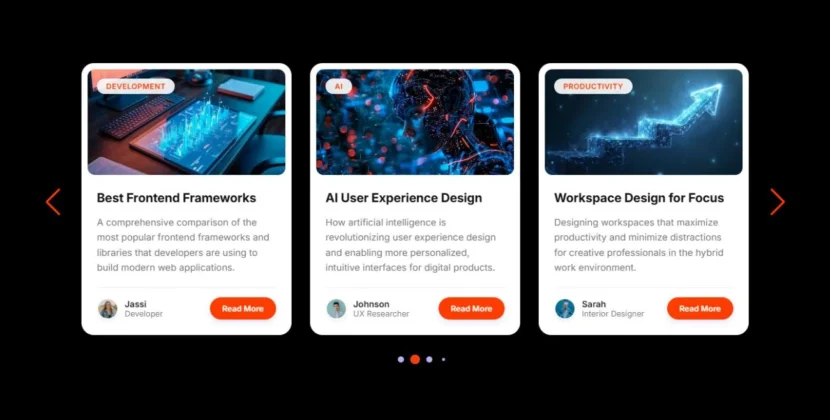
Z-Index Transitions: Enhance Your Images with CSS
In this tutorial, we’ll guide you through creating Z-Index transitions, an interactive effect on images using only CSS. This approach is perfect for web developers looking to enhance user engagement by adding dynamic visual effects to images. By utilizing CSS alone, you can create seamless transitions that add depth to your design and improve the user experience without the need for JavaScript.
Whether you’re a beginner or an experienced developer, you’ll find value in learning how to implement Z-Index transitions on your website. Not only do these effects provide a professional look, but they also keep your site lightweight and fast. In this step-by-step guide, you’ll discover just how easy it is to create this eye-catching effect using only HTML and CSS.
What is a Z-Index Transition?
Z-Index transitions are a CSS effect that allows specific elements, such as images or cards, to layer over one another in a smooth and visually appealing manner. This technique is widely used in image galleries, product cards, and various designs where visual hierarchy plays a key role in user interaction.
Why Master Z-Index Transitions?
Mastering Z-Index transitions offers several advantages:
- Enhances User Interaction: This technique adds a dynamic and engaging visual effect to your website.
- Lightweight Design: Since no JavaScript is needed, you can ensure faster load times.
- Improves SEO: A faster, cleaner website contributes to better rankings on search engines.
- Responsive and Accessible: When implemented correctly, these transitions work beautifully across all devices.
How to Create Z-Index Transitions on Images with CSS
Here’s a step-by-step guide to building this visually appealing feature using only HTML and CSS.
Set Up Your Basic HTML Structure
Start by creating the structure of your HTML, where each image or card will have its section. Below is an example of how to set up the basic HTML for this:
<!DOCTYPE html><!-- Website - www.abhikesh.com --><html lang="en" dir="ltr"><head><meta charset="UTF-8" /><title>Image Hover Animation HTML CSS | abhikesh</title><link rel="stylesheet" href="style.css" /></head><body><div class="container"><div class="icon-image"><div class="icon"><img src="images/img1.jpg" alt="" /></div><div class="hover-image one"><div class="img"><img src="images/img1.jpg" alt="" /></div><div class="content"><div class="details"><div class="name">Rahul</div><div class="job">Designer || Developer</div></div></div></div></div><div class="icon-image"><div class="icon"><img src="images/img2.jpg" alt="" /></div><div class="hover-image one"><div class="img"><img src="images/img2.jpg" alt="" /></div><div class="content"><div class="details"><div class="name">Rohit</div><div class="job">Blogger || Designer</div></div></div></div></div><div class="icon-image"><div class="icon"><img src="images/img3.jpg" alt="" /></div><div class="hover-image one"><div class="img"><img src="images/img3.jpg" alt="" /></div><div class="content"><div class="details"><div class="name">Arzu</div><div class="job">Designer || Developer</div></div></div></div></div><div class="icon-image"><div class="icon"><img src="images/img4.jpg" alt="" /></div><div class="hover-image one"><div class="img"><img src="images/img4.jpg" alt="" /></div><div class="content"><div class="details"><div class="name">Shivani</div><div class="job">Photographer || Youtuber</div></div></div></div></div><div class="icon-image"><div class="icon"><img src="images/img5.jpg" alt="" /></div><div class="hover-image one"><div class="img"><img src="images/img5.jpg" alt="" /></div><div class="content"><div class="details"><div class="name">Aryn</div><div class="job">Developer || Designer</div></div></div></div></div><div class="icon-image last"><div class="icon"><img src="images/img6.jpg" alt="" /></div><div class="hover-image one"><div class="img"><img src="images/img6.jpg" alt="" /></div><div class="content"><div class="details"><div class="name">Anil</div><div class="job">Blogger || Youtuber</div></div></div></div></div></div></body></html>
- Product Image: The image element that will display the product image.
- Card Info: This section will include product details such as the name, price, and an “Add to Cart” button.
Style with CSS for the Transition
Next, add CSS to style the product card and create smooth transitions when users hover over it. We will use the z-index property along with other CSS properties like transform and transition to create this effect.
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@200;300;400;500;600;700&display=swap');*{margin: 0;padding: 0;box-sizing: border-box;font-family: 'Poppins',sans-serif;}body{height: 100vh;width: 100%;display: flex;justify-content: center;align-items: center;background: #0396FF;}.container{height: 500px;display: flex;min-width: 400px;align-items: flex-end;justify-content: center;margin-top: -55px;}.icon-image{position: relative;height: 70px;width: 70px;margin: 0 5px;cursor: pointer;box-shadow: 0 5px 10px rgba(0, 0, 0, 0.25);border-radius: 50%;background: #fff;}.icon-image .icon img{position: absolute;height: 95%;width: 95%;left: 50%;top: 50%;transform: translate(-50%, -50%);object-fit: cover;border-radius: 50%;border: 3px solid #0396FF;}.icon-image::before{content: '';position: absolute;width: 50px;height: 50px;left: 50%;top: -50px;transform: translateX(-50%);}.icon-image .hover-image{position: absolute;height: 350px;width: 300px;bottom: 100px;left: 50%;z-index: 0;transform: translateX(-50%);border-radius: 25px;pointer-events: none;box-shadow: 0 5px 10px rgba(0, 0, 0, 0.15);transition: transform 0.5s ease, z-index 0s, left 0.5s ease ;transition-delay: 0s, 0.5s, 0.5s;}.icon-image:hover .hover-image{left: -200px;z-index: 12;transform: translateX(80px);transition: left 0.5s ease, z-index 0s, transform 0.5s ease;transition-delay: 0s, 0.5s, 0.5s;}.hover-image img{position: absolute;height: 100%;width: 100%;object-fit: cover;border: 3px solid #fff;border-radius: 25px;}.hover-image .content{position: absolute;width: 100%;bottom: -10px;padding: 0 10px;}.content::before{content: '';position: absolute;height: 20px;width: 20px;background: #fff;left: 50%;bottom: -7px;transform: rotate(45deg) translateX(-50%);z-index: -1;}.content .details{position: relative;background: #fff;padding: 10px;border-radius: 12px;text-align: center;opacity: 0;pointer-events: none;transform: translateY(10px);}.icon-image:hover .details{transition: all 0.5s ease;transition-delay: 0.9s;opacity: 1;transform: translateY(4px);pointer-events: auto;}.details::before{content: '';position: absolute;height: 20px;width: 20px;background: #fff;left: 50%;bottom: -15px;transform: rotate(45deg) translateX(-50%);z-index: -1;}.content .details .name{font-size: 20px;font-weight: 500;}.content .details .job{font-size: 17px;color: #0396FF;margin: -3px 0 5px 0;}.content .details a:hover{color: #0396FF;}.container .last .hover-image{transition: none;}.container .last:hover .hover-image{transition: 0;}.last:hover .details{transition-delay: 0s;}
This approach ensures the card adapts to mobile screens, maintaining a responsive and user-friendly design.
Best Practices for SEO and Performance
When building animated and interactive designs, it’s essential to consider SEO and performance optimization. Here are a few tips:
Semantic HTML: Use proper HTML tags like <h2>, <p>, and <button> to structure your content for search engines.
- Optimize Images: Compress images to reduce load times while maintaining high quality.
- Alt Text for Images: Use descriptive alt text to improve accessibility and SEO.
- Responsive Design: Ensure your designs are mobile-friendly by testing them across different screen sizes.
- Fast Load Times: Keep your CSS clean and lightweight to ensure faster loading times, which improves both user experience and SEO ranking.
Read Also
- Glassmorphism Login Form in HTML and CSS
Explore the stylish world of glassmorphism as you create a modern login form using HTML and CSS. This guide breaks down the design process step by step. - Toggle Button using HTML, CSS, and JavaScript
Discover how to enhance user interaction by creating a sleek toggle button with HTML, CSS, and JavaScript. This tutorial covers everything from structure to styling. - Responsive Cards in HTML and CSS
Learn how to design eye-catching responsive cards that adapt seamlessly to any device. This guide offers practical tips for achieving stunning layouts. - Build a Google Gemini Chatbot Using HTML, CSS, and JS
Dive into chatbot development by creating a Google Gemini chatbot with HTML, CSS, and JavaScript. This tutorial will help you understand the basics of interactive forms.
Conclusion
By following this guide, you’ve learned how to create stunning Z-Index transitions on images using only HTML and CSS. This powerful technique not only enhances your website’s visual appeal but also improves performance and SEO, all while maintaining a lightweight structure.
Ready to start building? You can download the complete source code below and try it out on your project.