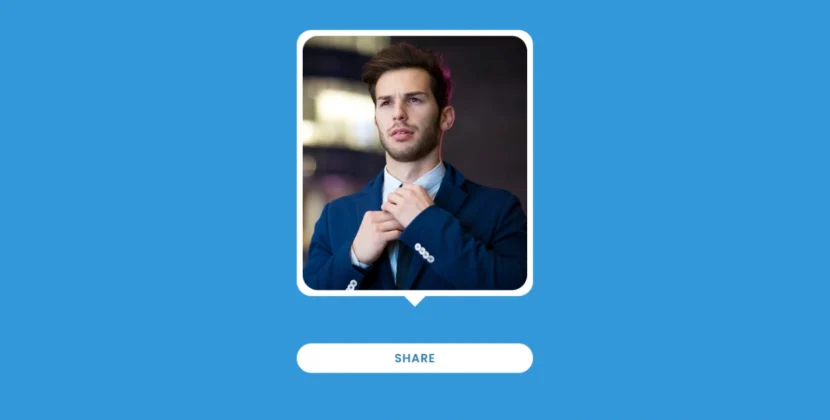
Hello friends, and welcome! Today, you will learn how to hide and show passwords using HTML, CSS, and JavaScript. I have developed various Login Forms with their animations and features; many of you have asked me for input field label animations and toggle password visibility features – here they are!
You can reveal or conceal your password’s letters by pressing the toggle button. This feature has been included as an extra security measure, as many users wish to keep their passwords private.
Look at the image below; it clearly demonstrates how to hide and reveal a password. In one input field, password letters remain concealed, while in another, we can clearly see them.
I created this project with code. By following along, you’ll learn how to code this project yourself!
Make an Array to Hide/Show Password in HTML CSS & JavaScript
Before jumping into source code development, it is vital to comprehend some critical points from this video tutorial.
Watching this video, it is clear that initially, there was only a grey border around our password field with an eye icon. But as soon as I focused on that password field, the text moved up, the border changed from grey to blue, and the eye icon and border colour altered accordingly – this animation was produced entirely using HTML CSS! To achieve such results.
I hope that creating the Show and Hide Password project is straightforward for you. If you need assistance building it, all necessary source codes are listed below.
Access Contact Form Validate Password on Login/Signup Form [Source Code]
After you have created two files—HTML and CSS—copy and paste their respective source codes from these two documents into this Login & Registration Form. By clicking download, you can get all its source code.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <!----==== CSS ====--> <link rel="stylesheet" href="style.css"> <!----==== Icounscout Link ====--> <link rel="stylesheet" href="https://unicons.iconscout.com/release/v4.0.0/css/line.css"> <title>Password Show & Hide</title> </head> <body> <div> <div> <input type="password" spellcheck="false" required> <label for="">Password</label> <i></i> </div> </div> <script> const toggle = document.querySelector(".toggle"), input = document.querySelector("input"); toggle.addEventListener("click", () =>{ if(input.type ==="password"){ input.type = "text"; toggle.classList.replace("uil-eye-slash", "uil-eye"); }else{ input.type = "password"; } }) </script> </body> </html>
/* Google Font Import - Poppins */ @import url('https://fonts.googleapis.com/css2?family=Poppins:wght@300;400;500;600;700&display=swap'); *{ margin: 0; padding: 0; box-sizing: border-box; font-family: 'Poppins', sans-serif; } body{ height: 100vh; display: flex; align-items: center; justify-content: center; background: #4070f4; } .container{ position: relative; max-width: 340px; width: 100%; padding: 20px; border-radius: 6px; background-color: #fff; } .container .input-box{ position: relative; height: 50px; } .input-box input{ position: absolute; height: 100%; width: 100%; outline: none; border: 2px solid #ccc; border-radius: 6px; padding: 0 35px 0 15px; transition: all 0.2s linear; } input:is(:focus, :valid){ border-color: #4070f4; } .input-box :is(label, i){ position: absolute; top: 50%; transform: translateY(-50%); color: #999; transition: all 0.2s linear; } .input-box label{ left: 15px; pointer-events: none; font-size: 16px; font-weight: 400; } input:is(:focus, :valid) ~ label{ color: #4070f4; top: 0; font-size: 12px; font-weight: 500; background-color: #fff; } .input-box i{ right: 15px; cursor: pointer; font-size: 20px; } input:is(:focus, :valid) ~ i{ color: #4070f4; }
View a live demo by clicking “view live.” If you experience difficulty creating this Password Show-and-Hide Project or its code does not function as expected, feel free to download a copy of its source code from here free of charge.
Finally, a tutorial that makes form validation easy to understand! Your explanations were thorough yet easy to follow. I’ll definitely be referring back to this post in the future.
I’ve been looking for a tutorial like this for ages! Your step-by-step approach and code examples made it easy to grasp the concepts. Can’t wait to implement form validation in my projects.
This tutorial saved me so much time and frustration. I struggled with form validation until I found this post. Now I feel like a pro! Thank you for the clear instructions.
Great post! I’ve always wondered how to implement the show/hide password feature, and your tutorial explained it perfectly. The combination of HTML, CSS, and JavaScript was simple yet effective. This is definitely something I’ll be using in my projects. Thanks for the clear instructions!
Fantastic guide! The tutorial on showing and hiding passwords using HTML, CSS, and JavaScript was very clear and practical. The JavaScript function and CSS styling tips were easy to understand and implement. This feature is a great addition to enhance user experience and security. Thanks for the excellent instructions!
Great tutorial! The guide on implementing the show/hide password feature with HTML, CSS, and JavaScript was clear and straightforward. I found the JavaScript implementation and CSS styling tips particularly helpful. This feature enhances user experience significantly. Thanks for the easy-to-follow instructions and practical examples!
Great tutorial! The feature to show/hide passwords is such a useful enhancement for user experience. Your guide made it easy to implement with HTML, CSS, and JavaScript. The explanations were straightforward, and the examples were practical. Thanks for sharing this—it’s going to be very useful for my web development work!
Awesome guide! The show/hide password feature is a fantastic addition to any form, and your tutorial made it straightforward to implement using HTML, CSS, and JavaScript. The explanations were clear and practical, making it easy to follow. Thanks for sharing these valuable insights—I’ll definitely use this in my projects!
Excellent guide! The feature to show/hide passwords is so useful for enhancing user experience and security. Your tutorial made it easy to implement this functionality using HTML, CSS, and JavaScript. The explanations were clear and concise. Thanks for the valuable tips—I’m excited to apply this to my own projects!
Excellent tutorial! The show/hide password feature is a great addition for improving user security and convenience. Your guide made it easy to implement with HTML, CSS, and JavaScript. The explanations were clear and the examples were practical. This will definitely enhance my forms. Thanks for the great insights!
Fantastic tutorial! The show/hide password feature is such a useful addition, and your guide made it simple to implement with HTML, CSS, and JavaScript. The instructions were clear and easy to follow. This will definitely enhance the user experience on my forms. Thanks for the great insights and practical examples!
Excellent tutorial! The show/hide password feature is such a great addition for improving user experience. Your guide made it easy to implement this functionality using HTML, CSS, and JavaScript. The instructions were clear and straightforward. Thanks for the valuable tips—I’m excited to incorporate this into my own projects!
Great guide! The show/hide password feature is so useful for enhancing security and user experience. Your tutorial made it really simple to implement with HTML, CSS, and JavaScript. The explanations were clear and practical. Thanks for sharing this valuable tip—I’m excited to use it in my own forms!
Awesome tutorial! The show/hide password feature is such a great user experience enhancement, and your guide made it very easy to implement. The explanations for HTML, CSS, and JavaScript were clear and straightforward. I’m looking forward to applying this to my own forms. Thanks for the excellent guide!
Excellent guide! The show/hide password feature is such a practical addition, and your tutorial made it simple to implement. The instructions for HTML, CSS, and JavaScript were clear and well-organized. This will definitely improve the functionality of my forms. Thanks for the great tips!
Fantastic tutorial! The show/hide password feature is a great addition for improving user experience, and your guide made it very straightforward to implement. The explanations for HTML, CSS, and JavaScript were clear and concise. I’m excited to use this on my own projects. Thanks for the helpful guide!
Great tutorial! The show/hide password feature is so useful for enhancing user experience. Your guide on implementing this with HTML, CSS, and JavaScript was very clear and easy to follow. I’m excited to integrate this into my forms. Thanks for the practical and well-explained instructions!
Excellent tutorial! The show/hide password feature is a great addition for improving user experience, and your guide made it simple to implement. The explanations for HTML, CSS, and JavaScript were clear and concise. This will definitely enhance my forms. Thanks for the detailed and practical instructions!
Wonderful tutorial! The show/hide password feature is a must-have for modern forms, and your guide made it straightforward to implement. The explanations for HTML, CSS, and JavaScript were very clear and easy to follow. I’m excited to apply this to my own projects. Thanks for the great tips!
Great tutorial! The ability to show and hide passwords is such a useful feature for forms. Your guide made it easy to implement this with HTML, CSS, and JavaScript. I particularly appreciated the clear explanations and practical examples. This will definitely enhance my web forms. Thanks for the detailed instructions!
Excellent guide! The show/hide password functionality is a crucial feature for enhancing user experience. Your step-by-step instructions for implementing this with HTML, CSS, and JavaScript were very clear and easy to understand. I’m looking forward to applying this to my own projects. Thanks for the insightful tutorial!
Excellent tutorial! The show/hide password feature is such a useful addition to forms. Your detailed explanation of how to implement this with HTML, CSS, and JavaScript was very clear and easy to follow. This will definitely enhance the functionality of my forms. Thanks for the great guide!
Great tutorial! The feature to show and hide passwords is such a practical addition to any form. Your clear and detailed instructions for HTML, CSS, and JavaScript made it easy to implement. I’m excited to use this on my site. Thanks for the helpful guide!
Awesome tutorial! The show/hide password feature is a great addition to improve user experience, and your guide made it simple to implement. The explanations for HTML, CSS, and JavaScript were very clear. I’m excited to integrate this into my forms. Thanks for the excellent instructions!
Great guide! The show/hide password feature is such a useful addition to forms. Your detailed explanations of the HTML, CSS, and JavaScript made the implementation straightforward. I found the step-by-step approach very helpful. Thanks for sharing this valuable tutorial!
Fantastic tutorial! The feature to show and hide passwords is a great enhancement for user experience, and your guide made it easy to implement. The explanations for HTML, CSS, and JavaScript were clear and detailed. I’m excited to use this in my own projects. Thanks for the great tips!
Fantastic tutorial! The show/hide password feature is such a useful addition to forms, and your guide made it easy to implement. The explanations for HTML, CSS, and JavaScript were clear and straightforward. This will definitely make my forms more user-friendly. Thanks for sharing!
Excellent guide! The feature to show and hide passwords adds a great user experience touch. Your instructions on HTML, CSS, and JavaScript were very clear and practical. This will definitely enhance my forms. Thanks for the detailed and easy-to-follow tutorial!
Awesome tutorial! The feature to show and hide passwords is such a practical enhancement for forms. Your step-by-step explanation of HTML, CSS, and JavaScript was very clear and easy to follow. This will definitely improve the user experience on my site. Thanks for the excellent guide!
Great guide! The show/hide password feature is a fantastic addition to any form, and your instructions made it really easy to implement. I appreciated the clear explanations for HTML, CSS, and JavaScript. This will definitely enhance the usability of my website. Thanks for the helpful tutorial!
Excellent tutorial! The feature to show and hide passwords is such a valuable addition to forms. Your clear instructions on HTML, CSS, and JavaScript made the implementation straightforward. I’m excited to use this in my own projects. Thanks for the great guide!
This is an excellent guide! Implementing the show/hide password feature is such a smart way to enhance user experience. Your step-by-step instructions for HTML, CSS, and JavaScript were easy to follow and very practical. Thanks for sharing this useful tip!
Great tutorial! The show/hide password feature is a fantastic addition to any form, and your guide made it easy to implement. The explanations for HTML, CSS, and JavaScript were very clear and practical. I’m excited to add this feature to my own projects. Thanks for the helpful insights!
Amazing tutorial! The feature to show and hide passwords is such a practical addition to any login form. Your guide was very clear and detailed, especially the JavaScript part. This will definitely improve the usability of my forms. Thanks for the insightful post!
This guide is excellent! The ability to show and hide passwords enhances user experience significantly. Your explanations of the HTML, CSS, and JavaScript code were very clear and easy to understand. I’m looking forward to implementing this feature on my website. Thanks for sharing such a useful tutorial!
Fantastic guide! The show/hide password functionality is such a valuable feature for user interfaces. Your detailed explanation of the HTML, CSS, and JavaScript involved was really helpful. I’m excited to add this feature to my own projects. Thanks for the clear and practical instructions!
This is an excellent tutorial! The feature to show and hide passwords adds a great user experience touch to forms. Your explanations of the HTML, CSS, and JavaScript were clear and concise. I found the implementation details very useful. Thanks for making this so accessible!
Great post! The show/hide password feature is a must-have for modern web forms, and your guide made it easy to implement. The explanations for HTML structure, CSS styling, and JavaScript functionality were clear and practical. This will definitely enhance the user experience on my site. Thanks for sharing!
This is a super helpful guide! The feature to show/hide the password is such a practical addition to forms. Your explanation of the HTML structure, CSS styling, and JavaScript functionality was spot-on. I can’t wait to implement this on my own projects. Thanks for sharing!
Excellent tutorial on adding a show/hide password feature! The HTML, CSS, and JavaScript code examples were very clear and easy to implement. This feature is a great addition to any login form, and your step-by-step guide made it simple to integrate. Thanks for the great tips!
Great post! The show/hide password feature is something I’ve wanted to add to my forms for a while. Your explanation of the HTML, CSS, and JavaScript code was clear and easy to follow. This will definitely enhance the user experience on my website. Thanks for the practical tips!