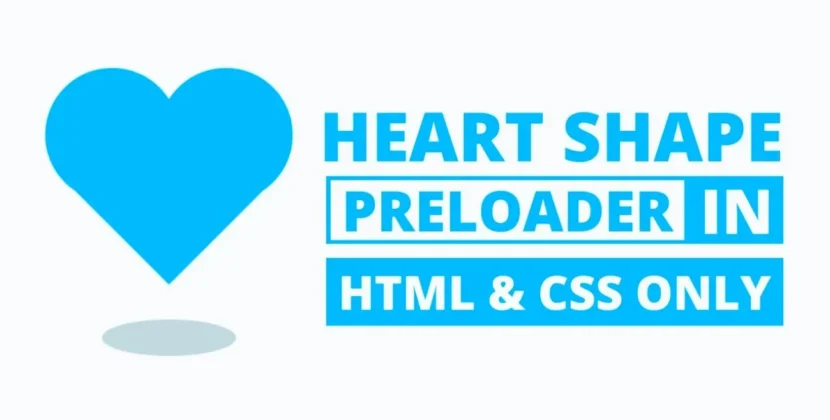
Are you ready to create your own responsive login and registration form in HTML and CSS? This detailed tutorial will show you how to create a responsive, user-friendly, and modern login and registration form. This form design can help you improve the user experience on your website, no matter if you’re a beginner in this field. Plus, you can also download the full source code to customize and use in your projects.
By the end of this guide, you’ll understand how to create a Responsive Login and Registration Form and optimize it for better performance and SEO. So, let’s get started!
What is a Responsive Login and Registration Form?
Websites that need user authentication must have a responsive login and registration form. While the registration form gathers user data for account creation, the login form enables users to enter their email address, phone number, or password to access the website. Combining both forms on a single page with a seamless design improves the user experience, especially on mobile devices.
The form is responsive, and it adjusts perfectly to a variety of screen sizes, ensuring that your website looks professional and operates efficiently on all devices.
Why Build a Responsive Login and Registration Form Using HTML & CSS?
Using HTML and CSS to create a responsive login and registration form has several benefits:
- Lightweight Design: HTML and CSS ensure your form loads quickly, which is critical for SEO and user retention.
- No JavaScript Required: This form uses only HTML and CSS, keeping your design simple and efficient while avoiding unnecessary JavaScript.
- Fully Responsive: CSS media queries ensure your form adapts seamlessly to various screen sizes, providing an optimal user experience.
- SEO-Friendly: By using semantic HTML and focusing on performance, your page will rank better in search engines.
Features of the Responsive Login and Registration Form
- Single-page Design: Both the login and registration forms are integrated on one page, and users can easily toggle between them with a smooth transition.
- CSS Flip Animation: The form smoothly flips between the login and registration sections, creating an engaging visual effect.
- No JavaScript: This design relies solely on CSS transitions, simplifying the development process.
- Responsive Layout: The form is designed to work perfectly across all devices, ensuring a great user experience.
- SEO Optimization: Semantic HTML tags and lightweight CSS ensure that your form loads quickly and ranks well in search engines.
Step-by-Step Guide: Building a Responsive Login and Registration Form
1. Setting Up the HTML Structure
Start by setting up the basic HTML structure. The HTML will contain two main sections: the login form and the registration form. The form toggles between login and registration using a checkbox and CSS transitions.
Here’s the basic HTML structure:
<!DOCTYPE html><!-- Created by abhikesh |www.abhikesh.com--><html lang="en" dir="ltr"><head><meta charset="UTF-8"><title> Login and Registration Form in HTML & CSS | abhikesh </title><link rel="stylesheet" href="style.css"><!-- Fontawesome CDN Link --><link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.3/css/all.min.css"><meta name="viewport" content="width=device-width, initial-scale=1.0"></head><body><div class="container"><input type="checkbox" id="flip"><div class="cover"><div class="front"><img src="images/frontImg.jpg" alt=""><div class="text"><span class="text-1">Every new friend is a <br> new adventure</span><span class="text-2">Let's get connected</span></div></div><div class="back"><img class="backImg" src="images/backImg.jpg" alt=""><div class="text"><span class="text-1">Complete miles of journey <br> with one step</span><span class="text-2">Let's get started</span></div></div></div><div class="forms"><div class="form-content"><div class="login-form"><div class="title">Login</div><form action="#"><div class="input-boxes"><div class="input-box"><i class="fas fa-envelope"></i><input type="text" placeholder="Enter your email" required></div><div class="input-box"><i class="fas fa-lock"></i><input type="password" placeholder="Enter your password" required></div><div class="text"><a href="#">Forgot password?</a></div><div class="button input-box"><input type="submit" value="Sumbit"></div><div class="text sign-up-text">Don't have an account? <label for="flip">Sigup now</label></div></div></form></div><div class="signup-form"><div class="title">Signup</div><form action="#"><div class="input-boxes"><div class="input-box"><i class="fas fa-user"></i><input type="text" placeholder="Enter your name" required></div><div class="input-box"><i class="fas fa-envelope"></i><input type="text" placeholder="Enter your email" required></div><div class="input-box"><i class="fas fa-lock"></i><input type="password" placeholder="Enter your password" required></div><div class="button input-box"><input type="submit" value="Sumbit"></div><div class="text sign-up-text">Already have an account? <label for="flip">Login now</label></div></div></form></div></div></div></div></body></html>2. Styling with CSS
Now that you have the structure, it’s time to style the form using CSS. The CSS will manage the layout, colors, and flip animation.
Here’s an example of the basic CSS for the form:
/* Google Font Link */@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@200;300;400;500;600;700&display=swap');* {margin: 0;padding: 0;box-sizing: border-box;font-family: "Poppins", sans-serif;}body {min-height: 100vh;display: flex;align-items: center;justify-content: center;background: #000;padding: 30px;}.container {position: relative;max-width: 850px;width: 100%;background: #fff;padding: 40px 30px;box-shadow: 0 5px 10px rgba(0, 0, 0, 0.2);perspective: 2700px;}.container .cover {position: absolute;top: 0;left: 50%;height: 100%;width: 50%;z-index: 98;transition: all 1s ease;transform-origin: left;transform-style: preserve-3d;backface-visibility: hidden;}.container #flip:checked ~ .cover {transform: rotateY(-180deg);}.container #flip:checked ~ .forms .login-form {pointer-events: none;}.container .cover .front,.container .cover .back {position: absolute;top: 0;left: 0;height: 100%;width: 100%;}.cover .back {transform: rotateY(180deg);}.container .cover img {position: absolute;height: 100%;width: 100%;object-fit: cover;z-index: 10;}.container .cover .text {position: absolute;z-index: 10;height: 100%;width: 100%;display: flex;flex-direction: column;align-items: center;justify-content: center;}.container .cover .text::before {content: '';position: absolute;height: 100%;width: 100%;opacity: 0.5;background: #FF014F;}.cover .text .text-1,.cover .text .text-2 {z-index: 20;font-size: 26px;font-weight: 600;color: #fff;text-align: center;}.cover .text .text-2 {font-size: 15px;font-weight: 500;}.container .forms {height: 100%;width: 100%;background: #fff;}.container .form-content {display: flex;align-items: center;justify-content: space-between;}.form-content .login-form,.form-content .signup-form {width: calc(100% / 2 - 25px);}.forms .form-content .title {position: relative;font-size: 24px;font-weight: 500;color: #333;}.forms .form-content .title:before {content: '';position: absolute;left: 0;bottom: 0;height: 3px;width: 25px;background: #FF014F;}.forms .signup-form .title:before {width: 20px;}.forms .form-content .input-boxes {margin-top: 30px;}.forms .form-content .input-box {display: flex;align-items: center;height: 50px;width: 100%;margin: 10px 0;position: relative;}.form-content .input-box input {height: 100%;width: 100%;outline: none;border: none;padding: 0 30px;font-size: 16px;font-weight: 500;border-bottom: 2px solid rgba(0, 0, 0, 0.2);transition: all 0.3s ease;}.form-content .input-box input:focus,.form-content .input-box input:valid {border-color: #FF014F;}.form-content .input-box i {position: absolute;color: #FF014F;font-size: 17px;}.forms .form-content .text {font-size: 14px;font-weight: 500;color: #333;}.forms .form-content .text a {text-decoration: none;}.forms .form-content .text a:hover {text-decoration: underline;}.forms .form-content .button {color: #fff;margin-top: 40px;}.forms .form-content .button input {color: #fff;background: #FF014F;border-radius: 6px;padding: 0;cursor: pointer;transition: all 0.4s ease;}.forms .form-content .button input:hover {background: #FF014F;}.forms .form-content label {color: #FF014F;cursor: pointer;}.forms .form-content label:hover {text-decoration: underline;}.forms .form-content .login-text,.forms .form-content .sign-up-text {text-align: center;margin-top: 25px;}.container #flip {display: none;}a{color: #FF014F;}@media (max-width: 730px) {.container .cover {display: none;}.form-content .login-form,.form-content .signup-form {width: 100%;}.form-content .signup-form {display: none;}.container #flip:checked ~ .forms .signup-form {display: block;}.container #flip:checked ~ .forms .login-form {display: none;}}3. Making the Form Responsive
To make sure the form works on all screen sizes, you’ll need to use CSS media queries. This ensures that the form adjusts its layout when viewed on smaller devices like phones and tablets.
Here’s an example:
@media (max-width: 730px) {.container .cover {display: none;}.form-content .login-form,.form-content .signup-form {width: 100%;}.form-content .signup-form {display: none;}.container #flip:checked ~ .forms .signup-form {display: block;}.container #flip:checked ~ .forms .login-form {display: none;}}This media query adjusts the width of the form for mobile devices and ensures the form is easy to interact with on smaller screens.
Best Practices for Optimizing Your Form for SEO
To ensure your Responsive Login and Registration Form performs well on search engines and offers a smooth user experience, follow these best practices:
- Fast Loading Speed: Keep your HTML and CSS code clean and efficient to minimize loading times, which is crucial for SEO.
- Keyword Optimization: Include relevant keywords like “Responsive Login and Registration Form,” “HTML and CSS,” and “UI Design” throughout your content for better search engine ranking.
- Semantic HTML: Use meaningful HTML tags like <h2>, <h3>, <form>, and <label> to structure your content in a way that is easy for search engines to understand.
- Alt Text for Images: If you include any images in your form, use alt text to describe them. This improves accessibility and helps search engines interpret the content.
- Mobile Responsiveness: Ensure your form is fully responsive by testing it on various devices and screen sizes. A mobile-friendly design improves SEO and user experience.
Want to Check Username Availability on Social Media?If you’re looking to check username availability on various social media platforms, visit NameChkr to find out!
Read Also
- Glassmorphism Login Form in HTML and CSS
Explore the stylish world of glassmorphism as you create a modern login form using HTML and CSS. This guide breaks down the design process step by step.- Toggle Button using HTML, CSS, and JavaScript
Discover how to enhance user interaction by creating a sleek toggle button with HTML, CSS, and JavaScript. This tutorial covers everything from structure to styling.- Responsive Cards in HTML and CSS
Learn how to design eye-catching responsive cards that adapt seamlessly to any device. This guide offers practical tips for achieving stunning layouts.- Build a Google Gemini Chatbot Using HTML, CSS, and JS
Dive into chatbot development by creating a Google Gemini chatbot with HTML, CSS, and JavaScript. This tutorial will help you understand the basics of interactive forms.Download the Source Code for Responsive Login and Registration Form
Ready to add this form to your website? You can download the complete source code for the Responsive Login and Registration Form by clicking the button below. Customize it for your project, and enjoy the simplicity of HTML and CSS to create a user-friendly interface.
This is exactly what I needed! The responsive design makes the login and registration forms look great on any device. Thank you for sharing the free code—it’s super helpful for beginners like me. Keep up the great work!
Great web site you have got here.. It’s difficult to find high-quality writing like yours these
days. I truly appreciate individuals like you! Taake care!!