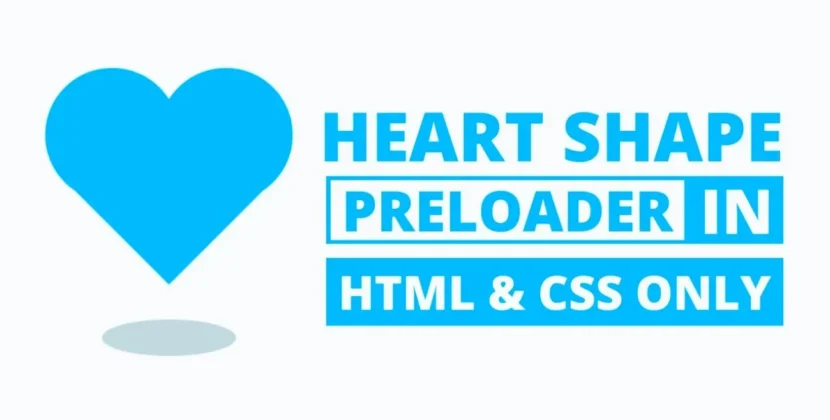
Sliders are a fantastic way to present content on websites, especially if you’re aiming for a clean and interactive design. Whether you’re showcasing products, portfolios, or images, a responsive card slider can really enhance your website’s user experience.
In this guide, I’ll show you how to create a responsive card slider using HTML, CSS, and JavaScript, and to make it even more appealing, we’ll incorporate the glassmorphism effect. Using SwiperJS, a popular JavaScript library for sliders, we can make the slider touch-friendly and fully responsive for both mobile and desktop.
By the end of this tutorial, you’ll have a fully functional and responsive image slider that you can add to your own website.
Why Use SwiperJS for a Responsive Slider?
SwiperJS is one of the best libraries available for creating interactive and mobile-friendly sliders. It’s easy to set up, highly customizable, and supports modern features like touch gestures, lazy loading, and autoplay.
If you prefer to understand the mechanics behind building sliders from scratch, you can also try creating a slider using vanilla JavaScript. It will help deepen your JavaScript skills, giving you full control over the slider’s behavior.
Now, let’s dive into building our responsive card slider!
Step-by-Step Guide to Creating a Responsive Card Slider with HTML, CSS & JavaScript
Step-by-Step Guide to Creating a Responsive Card Slider with HTML, CSS & JavaScript
1. Set Up Your Project Folder
To begin, create a folder for your project. You can name it something like card-slider. Inside the folder, create the following essential files:
- index.html for the HTML markup
- style.css for the CSS styles
- script.js for the JavaScript code
2. Prepare the Images
For the slider, you’ll need images. Place your images in a folder within the project directory called images. You can use any set of images you like, but it’s important to optimize them for faster loading on both mobile and desktop.
3. Write the HTML Code
In your index.html file, you’ll set up the structure of your card slider. Be sure to include the SwiperJS CDN links to enable all the functionality that the library offers.
In style.css, you’ll define how your slider looks. We’re using a modern glassmorphism effect, which will give your slider a frosted glass-like appearance. Feel free to play with different color schemes, shadows, and backgrounds to personalize the look.
In script.js, you’ll initialize SwiperJS and add the interactivity. This is where you can configure various features like autoplay, pagination, and touch gestures to make the slider functional across devices.
Read Also
- Glassmorphism Login Form in HTML and CSS
Explore the stylish world of glassmorphism as you create a modern login form using HTML and CSS. This guide breaks down the design process step by step. - Toggle Button using HTML, CSS, and JavaScript
Discover how to enhance user interaction by creating a sleek toggle button with HTML, CSS, and JavaScript. This tutorial covers everything from structure to styling. - Responsive Cards in HTML and CSS
Learn how to design eye-catching responsive cards that adapt seamlessly to any device. This guide offers practical tips for achieving stunning layouts. - Build a Google Gemini Chatbot Using HTML, CSS, and JS
Dive into chatbot development by creating a Google Gemini chatbot with HTML, CSS, and JavaScript. This tutorial will help you understand the basics of interactive forms.
Conclusion: Building a Responsive Slider for Modern Websites
By following this tutorial, you’ve successfully created a responsive card slider using HTML, CSS, and JavaScript (SwiperJS). This slider not only enhances your website’s visual appeal but also improves the user experience, especially for mobile users.
Feel free to customize the slider by experimenting with different settings in SwiperJS, such as pagination, navigation, and transitions. For more advanced customization, refer to the SwiperJS documentation to unlock its full potential and personalize your slider.
Additionally, if you prefer a ready-made version, you can download the project files by clicking the button below. This will allow you to explore the code in more detail or implement it directly into your own projects.