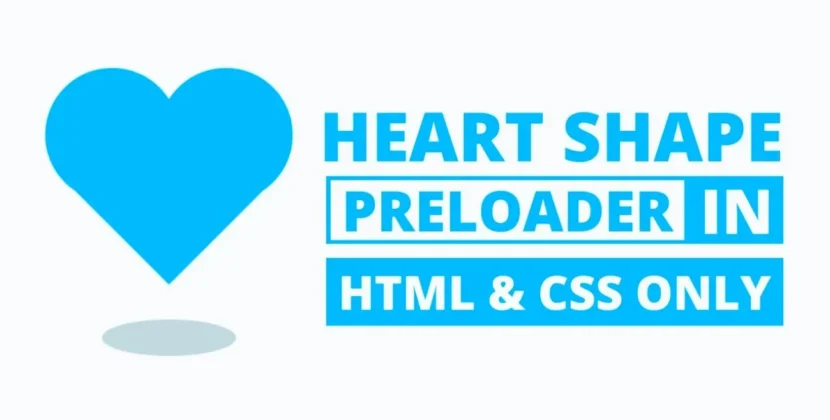
Hey there! Welcome to our blog! Today, we’re diving into an exciting project that’s not only fun but also visually stunning: creating a sleek Glassmorphism Calculator using just HTML and CSS. If you’re looking to spruce up your web design skills, you’re in the right place!
Understanding Glassmorphism Calculator
Before we jump into the fun stuff, let’s chat a bit about glassmorphism calculator. This design trend is all about that frosted glass effect that feels so modern and fresh. Imagine creating an interface that not only works well but also looks great—it’s like the icing on the cake for your web projects!
Setting Up the Project
So, let’s get started! First things first, we’ll need to set up our project. Don’t worry; it’s super easy!
- Create a new folder for your project.
- Inside that folder, make two files: index.html and style.css.
Features of Our Glassmorphism Calculator
Now, let’s talk about what our Glassmorphism Calculator will do. Here are some cool features we’ll implement:
- A visually appealing interface that draws users in with its glassy effect.
- Basic arithmetic operations: addition, subtraction, multiplication, and division—everything you need for those everyday calculations.
- Responsive design that looks fabulous on any device, so your calculator is ready to go wherever you are.
Building the HTML Structure
Let’s begin by crafting the HTML structure for our calculator. Below is the code that forms the basic layout:
<!DOCTYPE html><html lang="en" dir="ltr">Â <!-- Website - www.abhikesh.com -->Â <head>Â Â <meta charset="UTF-8" />Â Â <title>Glassmorphism Calculator HTML CSS JavaScript | CodingNepal</title>Â Â <link rel="stylesheet" href="style.css" />Â </head>Â <body>Â Â <div class="bubbles">Â Â Â <span class="one"></span>Â Â Â <span class="two"></span>Â Â Â <span class="three"></span>Â Â Â <span class="four"></span>Â Â Â <span class="five"></span>Â Â Â <span class="six"></span>Â Â Â <span class="seven"></span>Â Â Â <span class="seven"></span>Â Â </div>Â Â <div class="bubbles">Â Â Â <span class="one"></span>Â Â Â <span class="two"></span>Â Â Â <span class="three"></span>Â Â Â <span class="four"></span>Â Â Â <span class="five"></span>Â Â Â <span class="six"></span>Â Â Â <span class="seven"></span>Â Â Â <span class="seven"></span>Â Â </div>Â Â <div class="bubbles">Â Â Â <span class="one"></span>Â Â Â <span class="two"></span>Â Â Â <span class="three"></span>Â Â Â <span class="four"></span>Â Â Â <span class="five"></span>Â Â Â <span class="six"></span>Â Â Â <span class="seven"></span>Â Â Â <span class="seven"></span>Â Â </div>Â Â <div class="bubbles">Â Â Â <span class="one"></span>Â Â Â <span class="two"></span>Â Â Â <span class="three"></span>Â Â Â <span class="four"></span>Â Â Â <span class="five"></span>Â Â Â <span class="six"></span>Â Â Â <span class="seven"></span>Â Â Â <span class="seven"></span>Â Â </div>Â Â <div class="container">Â Â Â <form action="#" name="forms">Â Â Â Â <input type="text" name="answer" />Â Â Â Â <div class="buttons">Â Â Â Â Â <input type="button" value="AC" onclick="forms.answer.value = ''" />Â Â Â Â Â <input type="button" value="DEL" onclick="forms.answer.value = forms.answer.value.substr(0 , forms.answer.value.length -1)" />Â Â Â Â Â <input type="button" value="%" onclick="forms.answer.value += '%'" />Â Â Â Â Â <input type="button" value="/" onclick="forms.answer.value += '/'" />Â Â Â Â </div>Â Â Â Â <div class="buttons">Â Â Â Â Â <input type="button" value="7" onclick="forms.answer.value += '7'" />Â Â Â Â Â <input type="button" value="8" onclick="forms.answer.value += '8'" />Â Â Â Â Â <input type="button" value="9" onclick="forms.answer.value += '9'" />Â Â Â Â Â <input type="button" value="*" onclick="forms.answer.value += '*'" />Â Â Â Â </div>Â Â Â Â <div class="buttons">Â Â Â Â Â <input type="button" value="4" onclick="forms.answer.value += '4'" />Â Â Â Â Â <input type="button" value="5" onclick="forms.answer.value += '5'" />Â Â Â Â Â <input type="button" value="6" onclick="forms.answer.value += '6'" />Â Â Â Â Â <input type="button" value="-" onclick="forms.answer.value += '-'" />Â Â Â Â </div>Â Â Â Â <div class="buttons">Â Â Â Â Â <input type="button" value="1" onclick="forms.answer.value += '1'" />Â Â Â Â Â <input type="button" value="2" onclick="forms.answer.value += '2'" />Â Â Â Â Â <input type="button" value="3" onclick="forms.answer.value += '3'" />Â Â Â Â Â <input type="button" value="+" onclick="forms.answer.value += '+'" />Â Â Â Â </div>Â Â Â Â <div class="buttons">Â Â Â Â Â <input type="button" value="0" onclick="forms.answer.value += '0'" />Â Â Â Â Â <input type="button" value="00" onclick="forms.answer.value += '00'" />Â Â Â Â Â <input type="button" value="." onclick="forms.answer.value += '.'" />Â Â Â Â Â <input type="button" value="=" onclick="forms.answer.value = eval(forms.answer.value)" />Â Â Â Â </div>Â Â Â </form>Â Â </div>Â </body></html>
Explanation of the HTML Code
- The <div class=”bubbles”> contains several <span> elements that create animated bubbles, giving a dynamic feel to the background.
- The <div class=”container”> holds the calculator layout, including an input field for the calculations and various buttons for user interaction.
Styling with CSS
Now, let’s style our calculator to give it that beautiful glass morphism effect. Here’s how the CSS looks:
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@200;300;400;500;600;700&display=swap');*{Â margin: 0;Â padding: 0;Â box-sizing: border-box;Â font-family: 'Poppins',sans-serif;}body{Â height: 100vh;Â width: 100%;Â overflow: hidden;Â display: flex;Â justify-content: center;Â align-items: center;Â background: linear-gradient(#2196f3 , #e91e63);}.bubbles{Â position: absolute;Â bottom: -120px;Â display: flex;Â flex-wrap: wrap;Â margin-top: 70px;Â width: 100%;Â justify-content: space-around;}.bubbles span{Â height: 60px;Â width: 60px;Â background: rgba(255, 255, 255, 0.1);Â animation: move 10s linear infinite;Â position: relative;Â overflow: hidden;}@keyframes move {Â 100%{Â Â transform: translateY(-100vh);Â }}.bubbles span.one{Â animation-delay: 2.2s;Â transform: scale(2.15)}.bubbles span.two{Â animation-delay: 3.5s;Â transform: scale(1.55);}.bubbles span.three{Â animation-delay: 0.2s;Â transform: scale(0.35);}.bubbles span.four{Â animation-delay: 6s;Â transform: scale(2.15);}.bubbles span.five{Â animation-delay: 7s;Â transform: scale(0.5);}.bubbles span.six{Â animation-delay: 4s;Â transform: scale(2.5);}.bubbles span.seven{Â animation-delay: 3;Â transform: scale(1.5);}.bubbles span:before{Â content: '';Â position: absolute;Â left: 0;Â top: 0;Â height: 60px;Â width: 40%;Â transform: skew(45deg) translateX(150px);Â background: rgba(255, 255, 255, 0.15);Â animation: mirror 3s linear infinite;}@keyframes mirror {Â 100%{Â Â transform: translateX(-450px);Â }}.bubbles span.one:before{Â animation-delay: 1.5s;}.bubbles span.two:before{Â animation-delay: 3.5s;}.bubbles span.three:before{Â animation-delay: 2.5s;}.bubbles span.four:before{Â animation-delay: 7.5s;}.bubbles span.five:before{Â animation-delay: 4.5s;}.bubbles span.six:before{Â animation-delay: 0.5s;}.bubbles span.seven:before{Â animation-delay: 6s;}.container{Â z-index: 12;Â width: 420px;Â padding: 15px;Â border-radius: 12px;Â background: rgba(255, 255, 255, 0.1);Â box-shadow: 0 20px 50px rgba(0, 0, 0, 0.15);Â border-top: 1px solid rgba(255, 255, 255, 0.5);Â border-left: 1px solid rgba(255, 255, 255, 0.5);}.container input[type="text"]{Â width: 100%;Â height: 100px;Â margin: 0 3px;Â outline: none;Â border: none;Â color: #fff;Â font-size: 30px;Â text-align: right;Â padding-right: 10px;Â background: transparent;}.container input[type="button"]{Â height: 75px;Â color: #fff;Â width: calc(100% / 4 - 5px);Â background: transparent;Â border-radius: 12px;Â margin-top: 15px;Â outline: none;Â border: none;Â font-size: 25px;Â cursor: pointer;Â transition: all 0.3s ease;}.container input[type="button"]:hover{Â background: rgba(255, 255, 255, 0.1);}
Explanation of the CSS Code
The CSS file starts with an import statement to include the Poppins font from Google Fonts.
- The body element is styled with a vibrant gradient background, ensuring a visually appealing backdrop for the calculator.
- The .container class applies the glass morphism effect with a transparent background and rounded edges, along with subtle shadows for depth.
Read Also
- Glassmorphism Login Form in HTML and CSS
Explore the stylish world of glassmorphism as you create a modern login form using HTML and CSS. This guide breaks down the design process step by step. - Toggle Button using HTML, CSS, and JavaScript
Discover how to enhance user interaction by creating a sleek toggle button with HTML, CSS, and JavaScript. This tutorial covers everything from structure to styling. - Responsive Cards in HTML and CSS
Learn how to design eye-catching responsive cards that adapt seamlessly to any device. This guide offers practical tips for achieving stunning layouts. - Build a Google Gemini Chatbot Using HTML, CSS, and JS
Dive into chatbot development by creating a Google Gemini chatbot with HTML, CSS, and JavaScript. This tutorial will help you understand the basics of interactive forms.
Conclusion
Congratulations! You’ve just created a beautiful Glassmorphism Calculator using only HTML and CSS. This project showcases not only your coding skills but also your ability to implement modern design trends effectively.
Feel free to experiment with the code, tweak the styles, and make it your own. The beauty of web development lies in the endless possibilities for customization!
Download Source Code
If you want to get your hands on the full source code for this glass morphism calculator, simply click the button below: