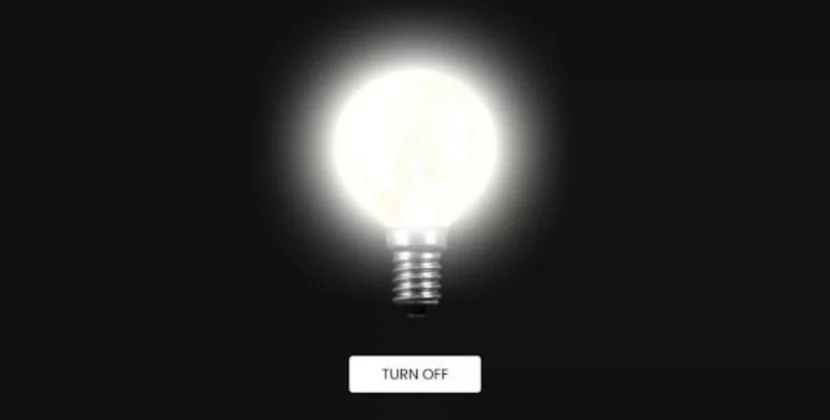
Are you ready to create a Flipping Card UI Design in HTML & CSS? This tutorial will guide you to build a sleek, modern flipping card animation using only HTML and CSS. Whether you’re a beginner or an experienced developer looking to add flair to your web designs, mastering this effect will enhance your skillset and make your projects stand out.
By the end of this guide, you’ll not only understand how to create a Flipping Card UI Design, but you’ll also be able to download the source code and customize it for your use. Let’s get started!
Introduction to Flipping Card UI Design
A Flipping Card UI Design adds interactive and visually appealing elements to your website. It’s a design feature where the card “flips” when hovered over, revealing more information on the other side. This technique is widely used in modern web design, especially for displaying product information, portfolio items, or cards containing key details concisely.
By learning to create a Flipping Card UI Design using HTML & CSS, you can add unique interactivity to your web projects without relying on JavaScript, ensuring faster load times and smoother performance.
Why Use HTML & CSS for Flipping Card UI?
Using HTML & CSS for your Flipping Card UI offers several benefits:
- Ease of Use: HTML and CSS are straightforward, making it easy to create interactive designs without complex code.
- No JavaScript Required: You can achieve smooth animations using just CSS, which keeps your design lightweight and SEO-friendly.
- Responsive Design: CSS allows you to make the design responsive, ensuring that your flipping cards work perfectly on mobile devices and different screen sizes.
- Improved Load Speed: By sticking to HTML & CSS, you minimize the need for heavy libraries, which leads to faster load times—a key factor for SEO.
Incorporating Flipping Card UI Design not only improves the user experience but also enhances the visual appeal of your site, keeping visitors engaged.
How to Create a Flipping Card UI Design: Step-by-Step Guide
1. Setting Up HTML Structure
The foundation of your Flipping Card UI Design lies in the HTML structure. Start by setting up the basic layout for the card and its content. Here’s how you can create the skeleton of your flipping card.
<!DOCTYPE html><html lang="en"><head><meta charset="UTF-8" /><meta name="viewport" content="width=device-width, initial-scale=1.0" /><title>Flipping Card UI Design</title><link rel="stylesheet" href="style.css" /></head><body><section><div class="container"><div class="card front-face"><header><span class="logo"><img src="images/logo.png" alt="" /><h5>Master Card</h5></span><img src="images/chip.png" alt="" class="chip" /></header><div class="card-details"><div class="name-number"><h6>Card Number</h6><h5 class="number">5050 4040 3030 2020</h5><h5 class="name">Abhikesh Kumar</h5></div><div class="valid-date"><h6>Valid Thru</h6><h5>00/30</h5></div></div></div><div class="card back-face"><h6>For customer service call +977 1111 3433 or email at</h6><span class="magnetic-strip"></span><div class="signature"><i>001</i></div><h5>Lorem ipsum dolor sit amet, consectetur adipisicing elit. Officiamaiores sed doloremque nesciunt neque beatae voluptatibus doloribus.Libero et quis magni magnam nihil temporibus? Facere consecteturdolore reiciendis et veniam.</h5></div></div></section></body></html>
In this HTML code:
- Card-container: Contains the card and manages the overall layout.
- Front and back classes represent the two sides of the flipping card.
Make sure to structure your HTML with semantic tags for better SEO and accessibility. Using elements like <header>, <section>, and <article> can improve how search engines interpret your content.
2. Styling with CSS
Now that the structure is in place, let’s style the Flipping Card with CSS. The CSS will control the size, color, and the actual flipping animation.
Create a style.css file and add the following code:
/* Import Google Font - Poppins */@import url("https://fonts.googleapis.com/css2?family=Poppins:wght@300;400;500;600&display=swap");* {margin: 0;padding: 0;box-sizing: border-box;font-family: "Poppins", sans-serif;}section {position: relative;min-height: 100vh;width: 100%;background: #121321;display: flex;align-items: center;justify-content: center;color: #fff;perspective: 1000px;}section::before {content: "";position: absolute;height: 240px;width: 240px;border-radius: 50%;transform: translate(-150px, -100px);background: linear-gradient(90deg, #9c27b0, #f3f5f5);}section::after {content: "";position: absolute;height: 240px;width: 240px;border-radius: 50%;transform: translate(150px, 100px);background: linear-gradient(90deg, #9c27b0, #f3f5f5);}.container {position: relative;height: 225px;width: 375px;z-index: 100;transition: 0.6s;transform-style: preserve-3d;}.container:hover {transform: rotateY(180deg);}.container .card {position: absolute;height: 100%;width: 100%;padding: 25px;border-radius: 25px;backdrop-filter: blur(25px);background: rgba(255, 255, 255, 0.1);box-shadow: 0 25px 45px rgba(0, 0, 0, 0.25);border: 1px solid rgba(255, 255, 255, 0.1);backface-visibility: hidden; /* Ensure backface-visibility is set */transform-style: preserve-3d;}.front-face {backface-visibility: hidden;}.front-face header,.front-face .logo {display: flex;align-items: center;}.front-face header {justify-content: space-between;}.front-face .logo img {width: 48px;margin-right: 10px;}h5 {font-size: 16px;font-weight: 400;}.front-face .chip {width: 50px;}.front-face .card-details {display: flex;margin-top: 40px;align-items: flex-end;justify-content: space-between;}h6 {font-size: 10px;font-weight: 400;}h5.number {font-size: 18px;letter-spacing: 1px;}h5.name {margin-top: 20px;}.card.back-face {border: none;padding: 15px 25px 25px 25px;transform: rotateY(180deg);backface-visibility: hidden; /* Ensure backface-visibility is set */}.card.back-face h6 {font-size: 8px;}.card.back-face .magnetic-strip {position: absolute;top: 40px;left: 0;height: 45px;width: 100%;background: #000;}.card.back-face .signature {display: flex;justify-content: flex-end;align-items: center;margin-top: 80px;height: 40px;width: 85%;border-radius: 6px;background: repeating-linear-gradient(#fff,#fff 3px,#efefef 0px,#efefef 9px);}.signature i {color: #000;font-size: 12px;padding: 4px 6px;border-radius: 4px;background-color: #fff;margin-right: -30px;z-index: -1;}.card.back-face h5 {font-size: 8px;margin-top: 15px;}
In this CSS code:
- Perspective adds the 3D effect needed for the card flip.
- Transform and transition properties enable the smooth flip animation.
- Backface visibility hides the content of the back until the flip occurs.
This CSS will make your Flipping Card UI Design functional, responsive, and engaging for users.
Best Practices for Flipping Card UI
When creating a Flipping Card UI, follow these best practices to ensure it’s optimized for both SEO and user experience:
- Responsive Design: Make sure your card is mobile-friendly by using media queries to adjust the layout on smaller screens. Here’s an example of a responsive media query:
- Optimize for Speed: Keep the CSS simple and clean to reduce the load time of your page. Quick loading speeds play a crucial role in improving your website’s SEO performance.
- Alt Text for Accessibility: Include alt text for any images or visual elements within your card. This ensures that users with disabilities, and search engines, can interpret the content correctly.
- Semantic HTML: Use proper heading tags (<h2>, <h3>, etc.) and organize your content logically. This improves SEO by helping search engines understand the structure of your webpage.
- Keyword Optimization: Use trending keywords related to UI Design, HTML, CSS animations, and responsive web design to attract organic traffic.
@media (max-width: 600px) { .card.back-face h5 { font-size: 6px; margin-top: 15px; } }
Following these practices will make your Flipping Card UI Design not only look great but also perform well on search engines and across all devices.
Read Also
- Glassmorphism Login Form in HTML and CSS
Explore the stylish world of glassmorphism as you create a modern login form using HTML and CSS. This guide breaks down the design process step by step. - Toggle Button using HTML, CSS, and JavaScript
Discover how to enhance user interaction by creating a sleek toggle button with HTML, CSS, and JavaScript. This tutorial covers everything from structure to styling. - Responsive Cards in HTML and CSS
Learn how to design eye-catching responsive cards that adapt seamlessly to any device. This guide offers practical tips for achieving stunning layouts. - Build a Google Gemini Chatbot Using HTML, CSS, and JS
Dive into chatbot development by creating a Google Gemini chatbot with HTML, CSS, and JavaScript. This tutorial will help you understand the basics of interactive forms.
Download the Flipping Card Source Code
Ready to experiment with the code? You can download the full source code for the Flipping Card UI Design by clicking the button below. This gives you a head start to customize and use it in your projects.