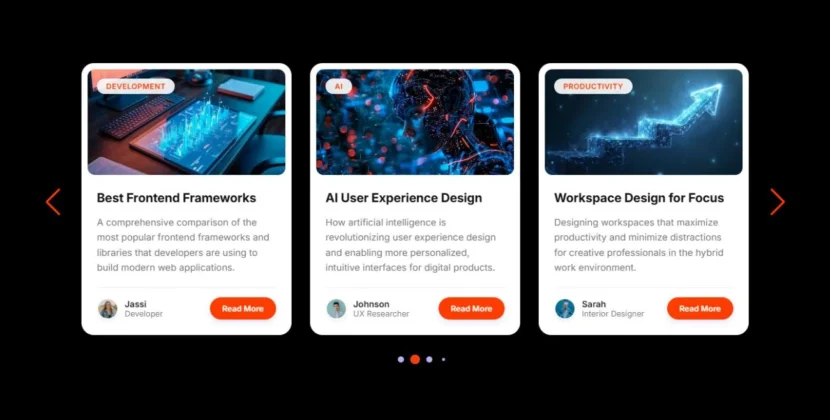
Creating a Digital Clock with Glowing Effect Using HTML, CSS, and JavaScript is a fantastic project for web developers of all levels. This blog will guide you through building a sleek digital clock that displays real-time with glowing gradient effects, combining aesthetics and functionality. Whether you’re enhancing your portfolio or adding interactive elements to your website, this project is a must-try.
Introduction to the Digital Clock with Glowing Effect
A Digital Clock shows time in numeric digits rather than using an analog clock’s rotating hands. In this tutorial, we’ll create a vibrant digital clock that fetches real-time data from your computer using JavaScript’s new Date() method and displays it with a colorful glowing background effect.
This project is perfect for beginners because it uses straightforward HTML, CSS, and JavaScript code. The glowing effect adds a visually appealing touch, making it ideal for modern web pages and personal projects.
Features of the Digital Clock with Glowing Effect
Here’s what makes this digital clock stand out:
- Real-Time Display: The clock fetches and displays the current time dynamically.
- Colorful Glowing Background: Adds an eye-catching gradient glow to the clock’s text and background.
- Simple Implementation: The code is concise and beginner-friendly, perfect for those just starting with web development.
- Customizable Design: Easily modify the gradient colors and font styles to suit your preferences or brand.
- No Server Dependency: This clock takes real-time data directly from your device, making it fast and efficient.
Benefits of Creating a Digital Clock with Glowing Effect
- Enhance Your Web Development Skills: This project strengthens your fundamentals of HTML, CSS, and JavaScript.
- Portfolio-Worthy Design: Showcase your creative skills with an attractive and functional digital clock.
- Improved User Engagement: Interactive elements like clocks can enhance the user experience on your website.
- Customizable: Tailor the glowing effect and design to match any theme or purpose.
How to Build the Digital Clock with Glowing Effect
To build the Digital Clock with a Glowing Effect, you need an HTML file and a CSS file. Follow the steps below:
HTML File
Start by creating the structure of the clock. Here’s the code for the HTML file:
<!DOCTYPE html><!-- Created By abhikesh.com --><html lang="en" dir="ltr"><head>Â Â <meta charset="utf-8">Â Â <title>Digital Clock with Glowing Effect | Abhikesh Kumar</title></head><body>Â Â <div class="wrapper">Â Â Â Â <div class="display">Â Â Â Â Â Â <div id="time"></div>Â Â Â Â </div>Â Â Â Â <span></span>Â Â Â Â <span></span>Â Â </div></body></html>
CSS File
The CSS file is where the magic happens, bringing the glowing effect to life and styling the digital clock beautifully.
Create a file named style.css and add the following code to transform your clock into a glowing masterpiece.
  <style>    * {      margin: 0;      padding: 0;      font-family: 'Poppins', sans-serif;    }    html,    body {      display: grid;      height: 100%;      place-items: center;      background: #000;    }    .wrapper {      height: 100px;      width: 360px;      position: relative;      background: linear-gradient(135deg, #14ffe9, #ffeb3b, #ff00e0);      border-radius: 10px;      cursor: default;      animation: animate 1.5s linear infinite;    }    .wrapper .display,    .wrapper span {      position: absolute;      top: 50%;      left: 50%;      transform: translate(-50%, -50%);    }    .wrapper .display {      z-index: 999;      height: 85px;      width: 345px;      background: #1b1b1b;      border-radius: 6px;      text-align: center;    }    .display #time {      line-height: 85px;      color: #fff;      font-size: 50px;      font-weight: 600;      letter-spacing: 1px;      background: linear-gradient(135deg, #14ffe9, #ffeb3b, #ff00e0);      -webkit-background-clip: text;      -webkit-text-fill-color: transparent;      animation: animate 1.5s linear infinite;    }    @keyframes animate {      100% {        filter: hue-rotate(360deg);      }    }    .wrapper span {      height: 100%;      width: 100%;      border-radius: 10px;      background: inherit;    }    .wrapper span:first-child {      filter: blur(7px);    }    .wrapper span:last-child {      filter: blur(20px);    }  </style>
JavaScript File
Finally, JavaScript is used to fetch real-time data and display it on the clock. Create a file named script.js and add this code:
<script>Â Â Â Â setInterval(() => {Â Â Â Â Â Â const time = document.querySelector(".display #time");Â Â Â Â Â Â let date = new Date();Â Â Â Â Â Â let hours = date.getHours();Â Â Â Â Â Â let minutes = date.getMinutes();Â Â Â Â Â Â let seconds = date.getSeconds();Â Â Â Â Â Â let day_night = "AM";Â Â Â Â Â Â if (hours > 12) {Â Â Â Â Â Â Â Â day_night = "PM";Â Â Â Â Â Â Â Â hours = hours - 12;Â Â Â Â Â Â }Â Â Â Â Â Â if (seconds < 10) {Â Â Â Â Â Â Â Â seconds = "0" + seconds;Â Â Â Â Â Â }Â Â Â Â Â Â if (minutes < 10) {Â Â Â Â Â Â Â Â minutes = "0" + minutes;Â Â Â Â Â Â }Â Â Â Â Â Â if (hours < 10) {Â Â Â Â Â Â Â Â hours = "0" + hours;Â Â Â Â Â Â }Â Â Â Â Â Â time.textContent = hours + ":" + minutes + ":" + seconds + " " + day_night;Â Â Â Â });Â Â </script>
Read Also
- Glassmorphism Login Form in HTML and CSS
Explore the stylish world of glassmorphism as you create a modern login form using HTML and CSS. This guide breaks down the design process step by step. - Toggle Button using HTML, CSS, and JavaScript
Discover how to enhance user interaction by creating a sleek toggle button with HTML, CSS, and JavaScript. This tutorial covers everything from structure to styling. - Responsive Cards in HTML and CSS
Learn how to design eye-catching responsive cards that adapt seamlessly to any device. This guide offers practical tips for achieving stunning layouts. - Build a Google Gemini Chatbot Using HTML, CSS, and JS
Dive into chatbot development by creating a Google Gemini chatbot with HTML, CSS, and JavaScript. This tutorial will help you understand the basics of interactive forms.
Conclusion and Source Code
With just a few lines of code, you can create a Digital Clock with a Glowing Effect that enhances your web design projects. This clock is visually appealing and easy to customize and implement on any webpage.
To download the complete source code, click the button below: