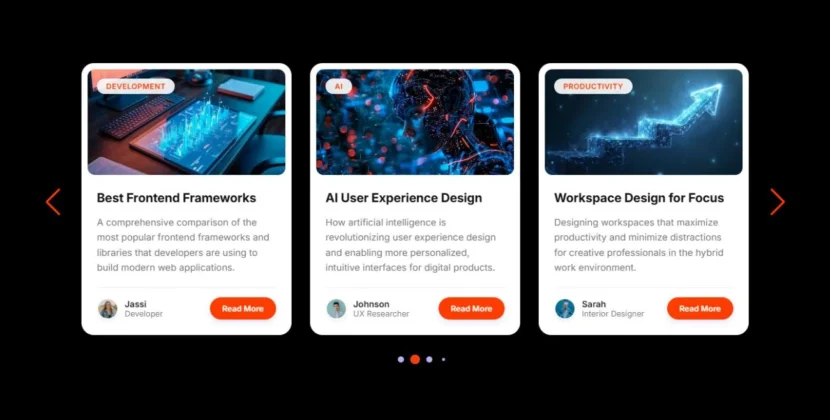
In today’s digital world, reacting to content with likes, hearts, and emojis has become a big part of social media. Whether you’re scrolling through Instagram or watching TikTok, the double-click heart animation is a familiar way to show love for content. This fun feature makes it easy and interesting for users to express their appreciation.
In this tutorial, we’ll show you how to create a stunning Double Click Heart Animation using HTML, CSS, and JavaScript. By the end, you’ll have a working heart animation that pops up when users double-click an image on your webpage. This feature is great for blogs, portfolios, or any web project that wants to encourage more interaction.
Why Add a Heart Animation to Your Website?
Incorporating animations like the heart icon is an excellent way to enhance your website’s interactivity. This feature not only captivates visitors visually but also encourages them to stay on your site longer, providing a fun way to interact with content.
Whether you’re developing a social platform, a personal blog, or an eCommerce site, adding interactive elements can significantly improve the overall user experience. This double-click animation is a straightforward yet effective approach to achieving that.
Steps to Create the Double Click Heart Animation
Let’s dive into creating the heart animation from the ground up. You should have a fundamental grasp of HTML, CSS, and JavaScript. Follow the steps below to ensure the animation functions as intended.
1. Set Up Your Project Folder
To start, create a dedicated folder for your project.
- index.html: This file will hold the structure of your HTML code.
- style.css: This file will include the styling for your project.
- script.js: This file will handle the animation logic using JavaScript.
2. Writing the HTML Code
The first step is to create the structure of your webpage. Here’s the HTML code that you’ll need to paste into your index.html file:
<!DOCTYPE html><!-- Coding by Abhikesh || www.abhikesh.com --><html lang="en"><head><meta charset="UTF-8" /><meta http-equiv="X-UA-Compatible" content="IE=edge" /><meta name="viewport" content="width=device-width, initial-scale=1.0" /><title>Double Click For Heart</title><link rel="stylesheet" href="style.css" /><link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.3.0/css/all.min.css" /><script src="script.js" defer></script></head><body><div class="container"><i class="fa-solid fa-heart heart"></i></div></body></html>
This basic HTML structure includes an image container and a placeholder for the animated heart. You can replace your-image.jpg with any image you’d like to use for the animation.
3. Styling the Animation in CSS
Now, let’s add some style to the animation. Here’s the code you need to paste into your style.css file:
/* Import Google font - Poppins */@import url("https://fonts.googleapis.com/css2?family=Poppins:wght@200;300;400;500;600;700&display=swap");* {margin: 0;padding: 0;box-sizing: border-box;font-family: "Poppins", sans-serif;}body {height: 100vh;display: flex;align-items: center;justify-content: center;background: #f6f7fb;}.container {position: relative;height: 420px;width: 320px;background-image: url("img.jpg");background-size: cover;background-position: center;border-radius: 12px;cursor: pointer;box-shadow: 0 15px 25px rgba(0, 0, 0, 0.1);}.heart {position: absolute;color: red;font-size: 40px;opacity: 0;transform: translate(-50%, -50%);}.heart.active {animation: animate 0.8s linear forwards;}@keyframes animate {30% {font-size: 80px;opacity: 1;}50% {opacity: 1;font-size: 60px;}70% {font-size: 70px;}80% {font-size: 60px;opacity: 1;}90% {font-size: 60px;opacity: 1;}}
This CSS code styles the image container, makes the heart icon invisible by default, and defines a scaling animation that will be triggered when the heart is clicked.
4. Adding Functionality with JavaScript
Now, let’s handle the double-click functionality using JavaScript. Here’s the code you need to paste into your script.js file:
// Select the container and heart elements from the DOMconst container = document.querySelector(".container"),heart = document.querySelector(".heart");// Add a double-click event listener to the containercontainer.addEventListener("dblclick", (e) => {// Calculate the x and y position of the double-click eventlet xValue = e.clientX - e.target.offsetLeft,yValue = e.clientY - e.target.offsetTop;// Set the position of the heart element using the x and y valuesheart.style.left = `${xValue}px`;heart.style.top = `${yValue}px`;// Add the active class to the heart element to animate itheart.classList.add("active");// Remove the active class after 1 secondsetTimeout(() => {heart.classList.remove("active");}, 1000);});
This JavaScript code listens for a double-click event on the image container. Once a double-click is detected, the heart icon becomes visible and scales up with a heartbeat animation.
5. Testing Your Animation
Once you’ve written the code, open the index.html file in your browser to see the animation in action. When you double-click the image, the heart animation should appear and then disappear after a short duration.
If the animation doesn’t work as expected, make sure that:
- The heart icon is correctly linked in the CSS (background: url(‘heart-icon. png’)).
- JavaScript is correctly linked in the HTML.
- Benefits of Adding Animations Like Double Click Heart
Interactive elements like this heart animation improve user experience by making your website more engaging. It also increases time spent on your site, which can boost your SEO rankings and help you better connect with your audience.
Animations like these can also encourage users to interact with your content more frequently, leading to higher click-through rates and, potentially, conversions.
Read Also
- Glassmorphism Login Form in HTML and CSS
Explore the stylish world of glassmorphism as you create a modern login form using HTML and CSS. This guide breaks down the design process step by step. - Toggle Button using HTML, CSS, and JavaScript
Discover how to enhance user interaction by creating a sleek toggle button with HTML, CSS, and JavaScript. This tutorial covers everything from structure to styling. - Responsive Cards in HTML and CSS
Learn how to design eye-catching responsive cards that adapt seamlessly to any device. This guide offers practical tips for achieving stunning layouts. - Build a Google Gemini Chatbot Using HTML, CSS, and JS
Dive into chatbot development by creating a Google Gemini chatbot with HTML, CSS, and JavaScript. This tutorial will help you understand the basics of interactive forms.
Final Thoughts
Creating a Double Click Heart Animation in HTML, CSS, and JavaScript is not only a great way to make your website more interactive but also a simple and fun project for web developers of all skill levels. By following the steps outlined above, you can easily integrate this feature into your website.
If you run into any issues or simply want to skip the manual steps, feel free to download the source code by clicking the button below.
Download Source Code
This project is easy to implement and can be further customized to match your website’s design. We hope this tutorial was useful for you—happy coding!