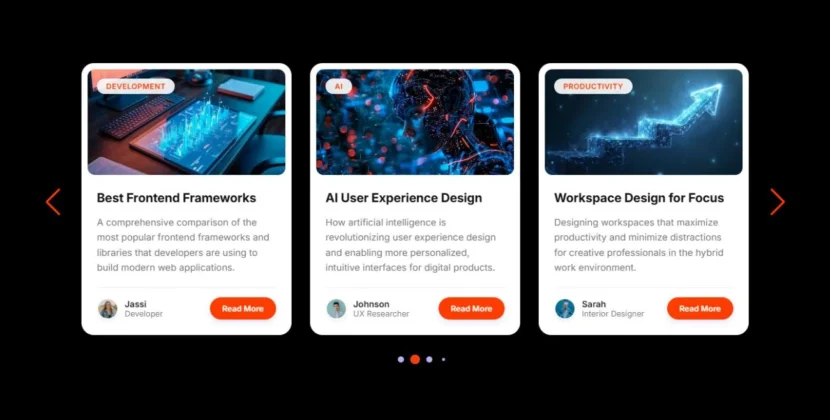
Hello friend! I hope your project is going smoothly, and I can’t wait to connect and create wonderful memories together soon! In this blog post, you will learn how to create a responsive Testimonial Slider using HTML, CSS, and SwiperJS/JavaScript. This tutorial will empower you to showcase customer reviews effectively.
What is a Testimonial Slider?
A Testimonial Slider displays reviews of products and services. For example, testimonials on a coffee shop website may include customer opinions about the coffee offered. This dynamic element enhances user engagement and builds trust with potential customers.
Project Overview
In this project, our Testimonial Slider will feature:
- An image of the reviewer
- Review text
- A quote icon
- The reviewer’s name and details
- Navigation buttons for sliding through testimonials
How Does It Work?
When interacting with the Testimonial Slider, users can click on the navigation buttons to slide through testimonials. Additionally, users can drag the slider to navigate between quotes easily. The design is completely responsive, effortlessly adjusting to various screen sizes.
Step-by-Step Guide to Create a Testimonial Slider
To create your Testimonial Slider, you must develop three files: an HTML file, a CSS file, and a JavaScript file. Here’s a quick summary of how to begin:
1. Create the HTML Structure
Add the following HTML code to your file to set up the basic structure of the Testimonial Slider:
<!DOCTYPE html><!-- Coding by abhikesh | www.abhikesh.com--><html lang="en"><head> <meta charset="UTF-8" /> <meta http-equiv="X-UA-Compatible" content="IE=edge" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Responsive Testimonial Slider</title> <!-- Swiper CSS --> <link rel="stylesheet" href="css/swiper-bundle.min.css" /> <!-- CSS --> <link rel="stylesheet" href="css/style.css" /> <!-- Boxicons CSS --> <link href="https://unpkg.com/boxicons@2.1.2/css/boxicons.min.css" rel="stylesheet" /></head><body> <section class="container">  <div class="testimonial mySwiper">   <div class="testi-content swiper-wrapper">    <div class="slide swiper-slide">     <img src="images/img1.jpg" alt="" class="image" />     <p>      Lorem ipsum dolor sit amet, consectetur adipisicing elit. Aperiam,      saepe provident dolorem a quaerat quo error facere nihil deleniti      eligendi ipsum adipisci, fugit, architecto amet asperiores      doloremque deserunt eum nemo.     </p>     <i class="bx bxs-quote-alt-left quote-icon"></i>     <div class="details">      <span class="name">Marnie Lotter</span>      <span class="job">Web Developer</span>     </div>    </div>    <div class="slide swiper-slide">     <img src="images/img2.jpg" alt="" class="image" />     <p>      Lorem ipsum dolor sit amet, consectetur adipisicing elit. Aperiam,      saepe provident dolorem a quaerat quo error facere nihil deleniti      eligendi ipsum adipisci, fugit, architecto amet asperiores      doloremque deserunt eum nemo.     </p>     <i class="bx bxs-quote-alt-left quote-icon"></i>     <div class="details">      <span class="name">Marnie Lotter</span>      <span class="job">Web Developer</span>     </div>    </div>    <div class="slide swiper-slide">     <img src="images/img3.jpg" alt="" class="image" />     <p>      Lorem ipsum dolor sit amet, consectetur adipisicing elit. Aperiam,      saepe provident dolorem a quaerat quo error facere nihil deleniti      eligendi ipsum adipisci, fugit, architecto amet asperiores      doloremque deserunt eum nemo.     </p>     <i class="bx bxs-quote-alt-left quote-icon"></i>     <div class="details">      <span class="name">Marnie Lotter</span>      <span class="job">Web Developer</span>     </div>    </div>   </div>   <div class="swiper-button-next nav-btn"></div>   <div class="swiper-button-prev nav-btn"></div>   <div class="swiper-pagination"></div>  </div> </section> <!-- Swiper JS --> <script src="js/swiper-bundle.min.js"></script> <!-- JavaScript --> <script src="js/script.js"></script></body></html>
2. Style the Slider with CSS
Next, include your CSS code to add styles and ensure that the Testimonial Slider looks visually appealing:
/* Google Fonts - Poppins */@import url("https://fonts.googleapis.com/css2?family=Poppins:wght@300;400;500;600&display=swap");* {Â margin: 0;Â padding: 0;Â box-sizing: border-box;Â font-family: "Poppins", sans-serif;}.container {Â height: 100vh;Â width: 100%;Â display: flex;Â align-items: center;Â justify-content: center;Â background-color: #e3f2fd;}.testimonial {Â position: relative;Â max-width: 900px;Â width: 100%;Â padding: 50px 0;Â overflow: hidden;}.testimonial .image {Â height: 170px;Â width: 170px;Â object-fit: cover;Â border-radius: 50%;}.testimonial .slide {Â display: flex;Â align-items: center;Â justify-content: center;Â flex-direction: column;Â row-gap: 30px;Â height: 100%;Â width: 100%;}.slide p {Â text-align: center;Â padding: 0 160px;Â font-size: 14px;Â font-weight: 400;Â color: #333;}.slide .quote-icon {Â font-size: 30px;Â color: #4070f4;}.slide .details {Â display: flex;Â flex-direction: column;Â align-items: center;}.details .name {Â font-size: 14px;Â font-weight: 600;Â color: #333;}.details .job {Â font-size: 12px;Â font-weight: 400;Â color: #333;}/* swiper button css */.nav-btn {Â height: 40px;Â width: 40px;Â border-radius: 50%;Â transform: translateY(30px);Â background-color: rgba(0, 0, 0, 0.1);Â transition: 0.2s;}.nav-btn:hover {Â background-color: rgba(0, 0, 0, 0.2);}.nav-btn::after,.nav-btn::before {Â font-size: 20px;Â color: #fff;}.swiper-pagination-bullet {Â background-color: rgba(0, 0, 0, 0.8);}.swiper-pagination-bullet-active {Â background-color: #4070f4;}@media screen and (max-width: 768px) {Â .slide p {Â Â padding: 0 20px;Â }Â .nav-btn {Â Â display: none;Â }download : swiper-bundle.min.css}
3. Implement Functionality with JavaScript
Finally, use JavaScript to add interactivity to your Testimonial Slider:
var swiper = new Swiper(".mySwiper", { slidesPerView: 1, grabCursor: true, loop: true, pagination: {  el: ".swiper-pagination",  clickable: true, }, navigation: {  nextEl: ".swiper-button-next",  prevEl: ".swiper-button-prev", },download : swiper-bundle.min.js});Demo of the Testimonial Slider
You can check out the demo of the Testimonial Slider to see how it functions. This hands-on experience will give you insight into the code and its behavior.
Want to Check Username Availability on Social Media?If you’re looking to check username availability on various social media platforms, visit NameChkr to find out!
Read Also
- Glassmorphism Login Form in HTML and CSS
Explore the stylish world of glassmorphism as you create a modern login form using HTML and CSS. This guide breaks down the design process step by step.- Toggle Button using HTML, CSS, and JavaScript
Discover how to enhance user interaction by creating a sleek toggle button with HTML, CSS, and JavaScript. This tutorial covers everything from structure to styling.- Responsive Cards in HTML and CSS
Learn how to design eye-catching responsive cards that adapt seamlessly to any device. This guide offers practical tips for achieving stunning layouts.- Build a Google Gemini Chatbot Using HTML, CSS, and JS
Dive into chatbot development by creating a Google Gemini chatbot with HTML, CSS, and JavaScript. This tutorial will help you understand the basics of interactive forms.Conclusion
Creating a Testimonial Slider is an engaging and practical project that enhances your web development skills. After following the steps outlined in this tutorial, you will have successfully built your own testimonial slider.
If you encounter difficulties during creation, use the button below to download the complete source code. This resource is available for free!