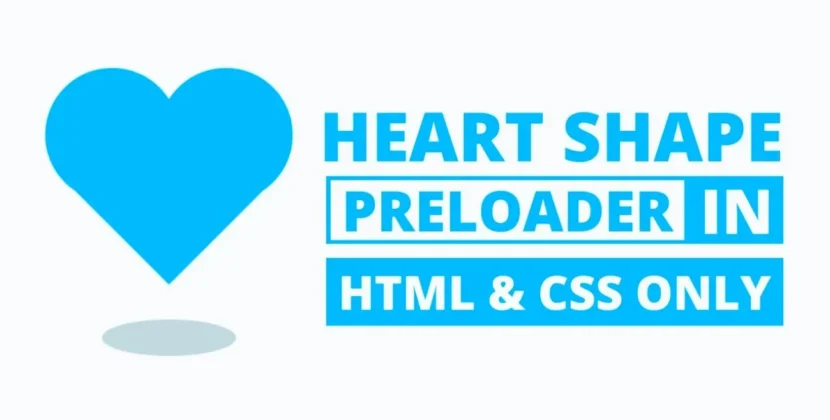
In today’s web development world, enhancing user experience with subtle animations can make a big difference. One popular interactive effect is the Button Ripple Animation, which provides immediate feedback to users when they click on a button. This tutorial will walk you through creating a smooth ripple animation using HTML, CSS, and JavaScript. Whether new to web development or looking to polish your existing skills, this tutorial will help you build a polished, professional-looking button animation.
Why Button Ripple Animation?
Button ripple animations create a visual confirmation that a button has been clicked. This effect starts from the point where the user clicks and expands outward like a ripple, making the interface feel more responsive and interactive. It’s a common technique used in material design and across modern websites and applications.
In this post, we’ll:
- Explore the steps to create a Button Ripple Animation from scratch.
- Walk through the HTML, CSS, and JavaScript code to build this animation.
- Provide the full source code at the end for easy download.
Let’s dive into how you can create this eye-catching effect.
Understanding the Button Ripple Animation
When a button is clicked, a ripple effect appears starting from the point of the click and expands outward. This effect is created using a combination of CSS animations and JavaScript for interaction. In essence, a hidden element (a circle or “ripple”) is dynamically added to the button when it’s clicked, and its size and position are adjusted based on where the user clicked.
Key Benefits of Adding Ripple Animations:
- User Feedback: Provides visual feedback, improving user experience.
- Modern Look: Adds a contemporary, sleek feel to your buttons.
- Enhanced Interaction: This makes your interface feel more responsive and interactive.
Step-by-Step: Building the Button Ripple Animation
Follow the steps below to build your button ripple effect:
1. Setting Up the Project Files
First, create a folder for your project, and inside the folder, create two files:
- index.html: The main HTML file.
- style.css: The stylesheet for styling the button and animation.
2. Writing the HTML Code
In the index.html file, create the basic structure of the webpage and the button that will display the ripple effect. Here’s the HTML code:
<!DOCTYPE html><!-- Coding By Abhikesh - abhikesh.com --><html lang="en" dir="ltr"> <head>  <meta charset="UTF-8">  <title> Button Ripple Effect | CodingLab </title>  <link rel="stylesheet" href="style.css">  </head><body> <div class="buttons">  <a class="button"href="#">Button</a>  <a class="button two" href="#">Button</a> </div> <script> // Ripple Effect JavaScript Code let buttons = document.querySelectorAll(".button"); for (var i = 0; i < buttons.length; i++) {  buttons[i].addEventListener("click", (e)=>{   e.preventDefault(); // preventing form submitting   let overlay = document.createElement('span'); //creating a tag(span)   overlay.classList.add("overlay"); //adding a class inside the span   e.target.appendChild(overlay); //adding overlay tag inside the anchor tag at in HTML   let xValue = e.clientX - e.target.offsetLeft; //by this we get perfect value where we will click   let yValue = e.clientY - e.target.offsetTop; //by this we get perfect value where we will click    overlay.style.left = xValue + "px"; //changing the position of the overlay according to our clicks on the button    overlay.style.top = yValue + "px"; //changing the position of the overlay according to our clicks on the button }); } </script></body></html>
In this HTML structure, we have a simple button that will trigger the ripple animation upon being clicked. We’re also using a bit of JavaScript to calculate the position of the click.
3. Writing the CSS Code
Now, move on to the style.css file to style the button and create the ripple animation. Below is the CSS code to make the button look good and animate the ripple:
/* Google Font Link */ @import url('https://fonts.googleapis.com/css2?family=Poppins:wght@200;300;400;500;600;700&display=swap'); *{ margin: 0; padding: 0; box-sizing: border-box; font-family: "Poppins" , sans-serif; } body{ height: 100vh; display: flex; align-items: center; justify-content: center; background: #350048; } .buttons .button{ position: relative; display: inline-block; color: #fff; padding: 12px 38px; background: linear-gradient(90deg, #6616d0, #ac34e7); border-radius: 45px; margin: 10px; font-size: 30px; font-weight: 400; text-decoration: none; box-shadow: 3px 5px rgba(0, 0, 0, 0.1); border-top: 1px solid rgba(0,0,0,0.1); overflow: hidden; } .buttons .button.two{ background: linear-gradient(90deg, #025ce3, #4e94fd); } .buttons .button .overlay{ position: absolute; background: #fff; top: 0; left: 0; transform: translate(-50%,-50%); border-radius: 50%; animation: blink 0.5s linear; } @keyframes blink { 0%{ height: 0px; width: 0px; opacity: 0.3; } 100%{ height: 400px; width: 400px; opacity: 0; } }
In this CSS code:
- .ripple-button: This class styles the button with padding, and a gradient background, and removes any borders.
- .ripple: This class defines the animated ripple effect that appears when the button is clicked. The ripple expands using a scaling animation (transform: scale()).
- @keyframes ripple-effect: This keyframe defines the animation for the ripple, which scales it up and fades it
JavaScript Explanation
In the script block within the HTML, we add a click event listener to each button. When the button is clicked, a new span element (representing the ripple) is created and positioned at the exact point where the user clicked. The ripple class is applied to animate the effect, and after the animation is complete (600ms), the ripple element is removed from the DOM to prevent clutter.
Additional Tips and Customizations
- Customizing Colors: You can easily adjust the gradient background of the button or the color of the ripple effect to match your website’s theme.
- Animation Duration: If you want the ripple to last longer, simply increase the animation duration in the CSS.
- Button Sizes: The button can be resized or given different styles like rounded corners or shadows to suit your design needs.
Read Also
- Glassmorphism Login Form in HTML and CSS
Explore the stylish world of glassmorphism as you create a modern login form using HTML and CSS. This guide breaks down the design process step by step. - Toggle Button using HTML, CSS, and JavaScript
Discover how to enhance user interaction by creating a sleek toggle button with HTML, CSS, and JavaScript. This tutorial covers everything from structure to styling. - Responsive Cards in HTML and CSS
Learn how to design eye-catching responsive cards that adapt seamlessly to any device. This guide offers practical tips for achieving stunning layouts. - Build a Google Gemini Chatbot Using HTML, CSS, and JS
Dive into chatbot development by creating a Google Gemini chatbot with HTML, CSS, and JavaScript. This tutorial will help you understand the basics of interactive forms.
Conclusion
Creating a Button Ripple Animation using HTML, CSS, and JavaScript is a fun and straightforward way to enhance the interactivity of your website. This animation is widely used across modern websites and apps, adding a professional touch to any interface.
By following this tutorial, you’ll not only create a beautiful ripple animation but also deepen your understanding of CSS animations and JavaScript DOM manipulation. The flexibility of this technique allows you to easily customize it for different styles and effects.
Download the Source Code
If you want to experiment further or need a quick start, you can download the complete source code for this button ripple animation. Click the button below to download all the files: