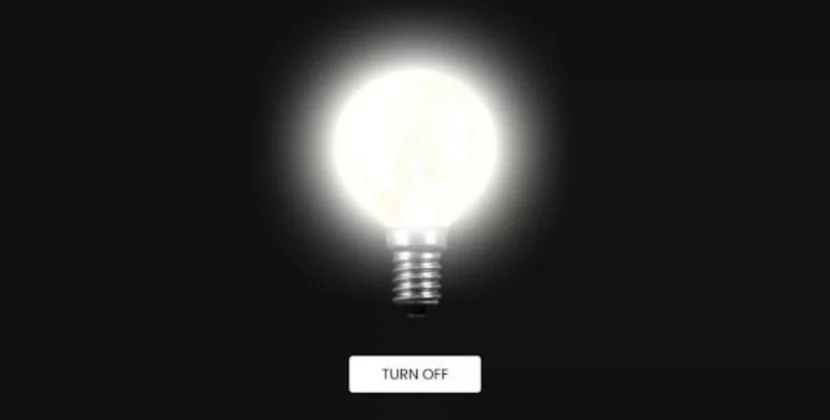
In today’s digital world, creating a responsive website in HTML and CSS is an essential skill for web designers and developers. Whether showcasing your portfolio, hosting a blog, or simply experimenting with web design, having a responsive site ensures your content looks great on any device.
This guide is perfect for beginners who want to build their first responsive website in HTML and CSS. We’ll walk you through creating an engaging homepage featuring an interactive navigation bar and styling elements that make your website visually appealing and user-friendly.
Why Build a Responsive Website in HTML and CSS?
Responsive websites adjust seamlessly across different screen sizes and devices, making them accessible to a broader audience. By using only HTML and CSS, you can create a fully functional and aesthetically pleasing website without relying on complex coding frameworks.
Step-by-Step Guide to Creating a Responsive Website in HTML and CSS
Follow these simple steps to build your very own responsive website:
Step 1: Set Up Your Project
- Create a folder for your project and name it something relevant.
- Inside this folder, create two files: index.html (for the HTML structure) and style.css (for the CSS styling).
Step 2: Create the HTML Structure
In your index.html file, add the following basic HTML markup. This includes semantic tags like <header>, <nav>, <h1>, <h2>, and <ul> for your navigation:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Responsive Website Homepage HTML and CSS</title> <link rel="stylesheet" href="style.css" /> </head> <body> <header class="header"> <nav class="navbar"> <h2 class="logo"><a href="#">abhikesh</a></h2> <input type="checkbox" id="menu-toggle" /> <label for="menu-toggle" id="hamburger-btn"> <svg xmlns="http://www.w3.org/2000/svg" height="24" viewBox="0 0 24 24" width="24"> <path d="M3 12h18M3 6h18M3 18h18" stroke="currentColor" stroke-width="2" stroke-linecap="round"/> </svg> </label> <ul class="links"> <li><a href="#">Home</a></li> <li><a href="#">About Us</a></li> <li><a href="#">Services</a></li> <li><a href="#">Portfolio</a></li> <li><a href="#">Contact Us</a></li> </ul> <div class="buttons"> <a href="#" class="signin">Sign In</a> <a href="#" class="signup">Sign Up</a> </div> </nav> </header> <section class="hero-section"> <div class="hero"> <h2>Mobile App Development</h2> <p> Join us in the exciting world of programming and turn your ideas into reality. Unlock the world of endless possibilities - learn to code and shape the digital future with us. </p> <div class="buttons"> <a href="#" class="join">Join Now</a> <a href="#" class="learn">Learn More</a> </div> </div> <div class="img"> <img src="https://abhikesh.com/blogs/wp-content/uploads/2023/10/hero-bg.png" alt="hero image" /> </div> </section> </body> </html>
Step 3: Apply CSS for Responsiveness
In your style.css file, you will add CSS code to style your website and ensure it’s responsive:
/* Importing Google font - Open Sans */ @import url("https://fonts.googleapis.com/css2?family=Open+Sans:wght@300;400;500;600;700&display=swap"); * { margin: 0; padding: 0; box-sizing: border-box; font-family: "Open Sans", sans-serif; } body { height: 100vh; width: 100%; background: linear-gradient(to bottom, #175d69 23%, #330c43 95%); } .header { position: fixed; top: 0; left: 0; width: 100%; } .navbar { display: flex; align-items: center; justify-content: space-between; max-width: 1200px; margin: 0 auto; padding: 20px 15px; } .navbar .logo a { font-size: 1.8rem; text-decoration: none; color: #fff; } .navbar .links { display: flex; align-items: center; list-style: none; gap: 35px; } .navbar .links a { font-weight: 500; text-decoration: none; color: #fff; padding: 10px 0; transition: 0.2s ease; } .navbar .links a:hover { color: #47b2e4; } .navbar .buttons a { text-decoration: none; color: #fff; font-size: 1rem; padding: 15px 0; transition: 0.2s ease; } .navbar .buttons a:not(:last-child) { margin-right: 30px; } .navbar .buttons .signin:hover { color: #47b2e4; } .navbar .buttons .signup { border: 1px solid #fff; padding: 10px 20px; border-radius: 0.375rem; text-align: center; transition: 0.2s ease; } .navbar .buttons .signup:hover { background-color: #47b2e4; color: #fff; } .hero-section { display: flex; justify-content: space-evenly; align-items: center; height: 95vh; padding: 0 15px; max-width: 1200px; margin: 0 auto; } .hero-section .hero { max-width: 50%; color: #fff; } .hero h2 { font-size: 2.5rem; margin-bottom: 20px; } .hero p { font-size: 1.2rem; margin-bottom: 20px; color: #c9c7c7; } .hero-section .img img { width: 517px; } .hero-section .buttons { margin-top: 40px; } .hero-section .buttons a { text-decoration: none; color: #fff; padding: 12px 24px; border-radius: 0.375rem; font-weight: 600; transition: 0.2s ease; display: inline-block; } .hero-section .buttons a:not(:last-child) { margin-right: 15px; } .buttons .join { background-color: #47b2e4; } .hero-section .buttons .learn { border: 1px solid #fff; border-radius: 0.375rem; } .hero-section .buttons a:hover { background-color: #47b2e4; } /* Hamburger menu styles */ #menu-toggle { display: none; } #hamburger-btn { font-size: 1.8rem; color: #fff; cursor: pointer; display: none; order: 1; } @media screen and (max-width: 1023px) { .navbar .logo a { font-size: 1.5rem; } .links { position: fixed; left: -100%; top: 75px; width: 100%; height: 100vh; padding-top: 50px; background: #175d69; flex-direction: column; transition: 0.3s ease; } .navbar #menu-toggle:checked ~ .links { left: 0; } .navbar #hamburger-btn { display: block; } .header .buttons { display: none; } .hero-section .hero { max-width: 100%; text-align: center; } .hero-section img { display: none; } }
Understanding the Code
- HTML Structure: This creates the website’s basic layout, including the header, navigation bar, and main content area.
- CSS Styling: We used a media query to make the navigation bar stack vertically on smaller screens, ensuring the website is fully responsive.
Benefits of Building a Responsive Website in HTML and CSS
- Improved User Experience: Users can view your website seamlessly across different devices, improving their overall experience.
- Easy Maintenance: Using pure HTML and CSS reduces the complexity of your site, making it easier to maintain and update.
- SEO-Friendly: A well-designed responsive website looks great and performs well in search engines, attracting more visitors.
Read Also
- Glassmorphism Login Form in HTML and CSS
Explore the stylish world of glassmorphism as you create a modern login form using HTML and CSS. This guide breaks down the design process step by step. - Toggle Button using HTML, CSS, and JavaScript
Discover how to enhance user interaction by creating a sleek toggle button with HTML, CSS, and JavaScript. This tutorial covers everything from structure to styling. - Responsive Cards in HTML and CSS
Learn how to design eye-catching responsive cards that adapt seamlessly to any device. This guide offers practical tips for achieving stunning layouts. - Build a Google Gemini Chatbot Using HTML, CSS, and JS
Dive into chatbot development by creating a Google Gemini chatbot with HTML, CSS, and JavaScript. This tutorial will help you understand the basics of interactive forms.
Final words and conclusion.
Building a responsive website in HTML and CSS is an exciting and productive project, especially for beginners. This guide teaches you the critical steps to creating a beautiful and adaptable homepage that works across all devices.
Don’t stop here! Continue experimenting by recreating other modern designs or adding new features to your website. The possibilities are endless with just HTML and CSS.
Download the Source Code
Click the button below to quickly download all the source code files for this project and start building your responsive website!
Comments
Unlock the Show and Hide Password Feature in HTML, CSS
Responsive Login & Registration Form in HTML & CSS | Free Code
Ultimate Overview of Creating a Stunning Login and Registration Form
Create a Powerful Functional Contact Form in PHP – Easy Tutorial
Create a Powerful Functional Contact Form in PHP – Easy Tutorial