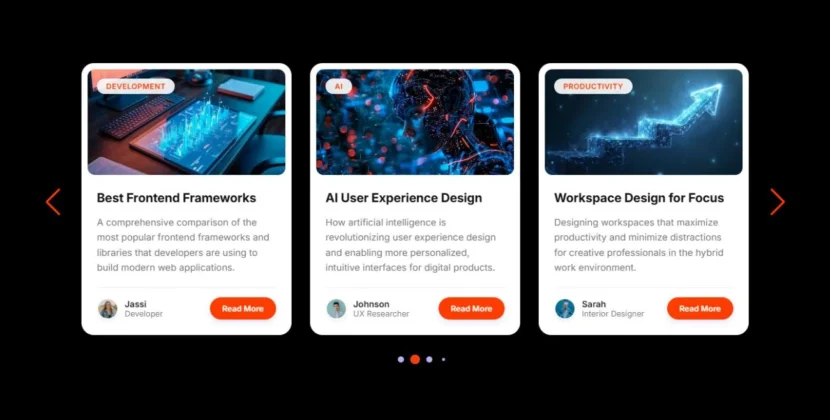
Creating an Animation Button in HTML, CSS, and JavaScript is an exciting way to enhance user interaction on your website. In this tutorial, you’ll learn how to build a button that animates with a click, providing an engaging experience for your visitors.
What is an Animation Button in HTML?
An Animation Button in HTML is a dynamic button that triggers visual effects when clicked. These animations can vary in style, such as changing colors, expanding in size, or producing ripple effects, enriching the user experience on web applications and sites.
Why Use Button Click Animation Button?
Button click animations serve several purposes:
- Engagement: Animated buttons attract user attention and encourage interaction.
- Feedback: They provide immediate visual feedback, confirming to users that their action has been recognized.
- Aesthetics: These animations can enhance the overall design of your website, making it look modern and appealing.
Steps for Creating an Animation Button
Let’s break down the process of creating an Animation Button in HTML into simple steps.
1. Project File Structure
To create this Animation Button, you’ll need to set up a project with two main files:
- index.html: This file will contain the HTML structure for your button.
- style.css: This file will hold all the CSS styles needed for the buttons and animations.
2. Designing the Button Click Animation
Now that your files are set up, it’s time to design your button.
HTML Structure: Open your index.html file and add the following code:
<!DOCTYPE html> <!-- Coding By abhikesh- abhikesh.com --> <html lang="en"> <head> <meta charset="UTF-8" /> <meta http-equiv="X-UA-Compatible" content="IE=edge" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Button Click Animation</title> <link rel="stylesheet" href="style.css" /> </head> <body> <button>Click Me</button> <script> const button = document.querySelector(".button"); button.addEventListener("click", (e) => { e.preventDefault; button.classList.add("animate"); setTimeout(() => { button.classList.remove("animate"); }, 600); }); </script> </body> </html>
CSS Styling: In your style.css file, include the following code to style your button and add the animation:
/* Import Google font - Poppins */ @import url("https://fonts.googleapis.com/css2?family=Poppins:wght@200;300;400;500;600;700&display=swap"); * { margin: 0; padding: 0; box-sizing: border-box; font-family: "Poppins", sans-serif; } body { display: flex; height: 100vh; display: flex; align-items: center; justify-content: center; background-color: #f0faff; } .button { position: relative; padding: 10px 22px; border-radius: 6px; border: none; color: #fff; cursor: pointer; background-color: #7d2ae8; transition: all 0.2s ease; } .button:active { transform: scale(0.96); } .button:before, .button:after { position: absolute; content: ""; width: 150%; left: 50%; height: 100%; transform: translateX(-50%); z-index: -1000; background-repeat: no-repeat; } .button.animate::before { top: -70%; background-image: radial-gradient(circle, #7d2ae8 20%, transparent 20%), radial-gradient(circle, transparent 20%, #7d2ae8 20%, transparent 30%), radial-gradient(circle, #7d2ae8 20%, transparent 20%), radial-gradient(circle, #7d2ae8 20%, transparent 20%), radial-gradient(circle, transparent 10%, #7d2ae8 15%, transparent 20%), radial-gradient(circle, #7d2ae8 20%, transparent 20%), radial-gradient(circle, #7d2ae8 20%, transparent 20%), radial-gradient(circle, #7d2ae8 20%, transparent 20%), radial-gradient(circle, #7d2ae8 20%, transparent 20%); background-size: 10% 10%, 20% 20%, 15% 15%, 20% 20%, 18% 18%, 10% 10%, 15% 15%, 10% 10%, 18% 18%; animation: greentopBubbles ease-in-out 0.6s forwards infinite; } @keyframes greentopBubbles { 0% { background-position: 5% 90%, 10% 90%, 10% 90%, 15% 90%, 25% 90%, 25% 90%, 40% 90%, 55% 90%, 70% 90%; } 50% { background-position: 0% 80%, 0% 20%, 10% 40%, 20% 0%, 30% 30%, 22% 50%, 50% 50%, 65% 20%, 90% 30%; } 100% { background-position: 0% 70%, 0% 10%, 10% 30%, 20% -10%, 30% 20%, 22% 40%, 50% 40%, 65% 10%, 90% 20%; background-size: 0% 0%, 0% 0%, 0% 0%, 0% 0%, 0% 0%, 0% 0%; } } .button.animate::after { bottom: -70%; background-image: radial-gradient(circle, #7d2ae8 20%, transparent 20%), radial-gradient(circle, #7d2ae8 20%, transparent 20%), radial-gradient(circle, transparent 10%, #7d2ae8 15%, transparent 20%), radial-gradient(circle, #7d2ae8 20%, transparent 20%), radial-gradient(circle, #7d2ae8 20%, transparent 20%), radial-gradient(circle, #7d2ae8 20%, transparent 20%), radial-gradient(circle, #7d2ae8 20%, transparent 20%); background-size: 15% 15%, 20% 20%, 18% 18%, 20% 20%, 15% 15%, 20% 20%, 18% 18%; animation: greenbottomBubbles ease-in-out 0.6s forwards infinite; } @keyframes greenbottomBubbles { 0% { background-position: 10% -10%, 30% 10%, 55% -10%, 70% -10%, 85% -10%, 70% -10%, 70% 0%; } 50% { background-position: 0% 80%, 20% 80%, 45% 60%, 60% 100%, 75% 70%, 95% 60%, 105% 0%; } 100% { background-position: 0% 90%, 20% 90%, 45% 70%, 60% 110%, 75% 80%, 95% 70%, 110% 10%; background-size: 0% 0%, 0% 0%, 0% 0%, 0% 0%, 0% 0%, 0% 0%; } }
Read Also
- Glassmorphism Login Form in HTML and CSS
Explore the stylish world of glassmorphism as you create a modern login form using HTML and CSS. This guide breaks down the design process step by step. - Toggle Button using HTML, CSS, and JavaScript
Discover how to enhance user interaction by creating a sleek toggle button with HTML, CSS, and JavaScript. This tutorial covers everything from structure to styling. - Responsive Cards in HTML and CSS
Learn how to design eye-catching responsive cards that adapt seamlessly to any device. This guide offers practical tips for achieving stunning layouts. - Build a Google Gemini Chatbot Using HTML, CSS, and JS
Dive into chatbot development by creating a Google Gemini chatbot with HTML, CSS, and JavaScript. This tutorial will help you understand the basics of interactive forms.
Conclusion
Following these steps, you have successfully created an HTML animation Button that reacts when clicked, providing a delightful user experience. Feel free to customize the button’s style and animations to match your website’s theme.
Feel free to share this tutorial with others if you found it helpful! Your support enables us to continue creating valuable content for the developer community. Thank you for reading!