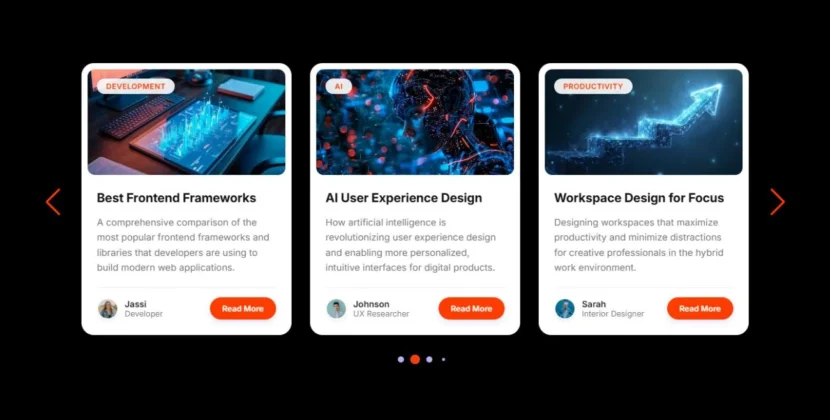
Are you looking for a simple yet stylish animated profile card design using only HTML and CSS? You’re in the right place! In this blog post, we’ll walk through creating an animated profile card that showcases a person’s profile details and some sleek animation effects. This design is perfect for portfolio websites, personal branding pages, or anywhere you want to present an individual’s identity confidently.
Whether you’re a beginner or an experienced developer, this tutorial will help you build a functional and aesthetically pleasing profile card. And the best part? No JavaScript is needed—everything is achieved with pure HTML and CSS!
What is a Profile Card?
A profile card is a visual representation of a person’s basic information. It typically includes the individual’s name, job title, social media links, and sometimes a photo. This compact way to present essential details about someone is commonly used on personal websites, business platforms, or portfolio pages.
Why Use HTML & CSS for Profile Cards?
Using only HTML and CSS for your profile card design ensures the code remains lightweight, fast to load, and easy to manage. No heavy JavaScript libraries are required, making your website more accessible and SEO-friendly.
With just HTML for structure and CSS for styling, you can create a clean and professional-looking profile card that looks great and performs well across all devices.
Features of Our Animated Profile Card
This animated profile card design includes the following features:
- A visually appealing layout with a picture, name, job title, and social media icons.
- A button that toggles the profile card’s visibility with sliding animations.
- Smooth animations for social media icons when hovered.
- Flexible layout optimized for all screen sizes.
Now, let’s explore the steps to bring this design to life!
Step-by-Step Guide to Building the Animated Profile Card
Step 1: Building the HTML Structure
The straightforward HTML structure includes elements like the profile image, name, job title, social media icons, and a button. Here’s how you can structure it:
<!DOCTYPE html><!-- Website - www.abhikesh.com --><html lang="en" dir="ltr"><head>Â <meta charset="UTF-8" />Â <title>Animated Profile Card HTML CSS | CodingNepal</title>Â <link rel="stylesheet" href="style.css" />Â <script src="https://kit.fontawesome.com/a076d05399.js"></script></head><body>Â <div class="main-box">Â Â <div class="content">Â Â Â <input type="checkbox" id="check" />Â Â Â <label class="box" for="check">Â Â Â Â <div class="share">SHARE</div>Â Â Â Â <div class="cancel">CANCEL</div>Â Â Â </label>Â Â Â <div class="image-box">Â Â Â Â <img src="james.jpeg" alt="" />Â Â Â Â <div class="about">Â Â Â Â Â <div class="details">Â Â Â Â Â Â <div class="name">Abhikesh</div>Â Â Â Â Â Â <div class="job">Devoloper | Designer</div>Â Â Â Â Â Â <div class="icon">Â Â Â Â Â Â Â <a href="#"><i class="fab fa-facebook-f"></i></a>Â Â Â Â Â Â Â <a href="#"><i class="fab fa-twitter"></i></a>Â Â Â Â Â Â Â <a href="#"><i class="fab fa-instagram"></i></a>Â Â Â Â Â Â Â <a href="#"><i class="fab fa-youtube"></i></a>Â Â Â Â Â Â </div>Â Â Â Â Â </div>Â Â Â Â </div>Â Â Â </div>Â Â </div>Â </div></body></html>
Step 2: Styling the Profile Card with CSS
Next, we’ll use CSS to style the card. We’ll apply Flexbox to centre the content and give the profile card a sleek look with modern design elements.
@import url("https://fonts.googleapis.com/css2?family=Poppins:wght@200;300;400;500;600;700&display=swap");* {Â margin: 0;Â padding: 0;Â box-sizing: border-box;Â font-family: "Poppins", sans-serif;}body {Â background: #3498db;}.main-box {Â position: absolute;Â left: 50%;Â top: 50%;Â transform: translate(-50%, -50%);Â width: 400px;Â height: 550px;Â text-align: center;}.main-box .content {Â position: relative;Â height: 100%;Â width: 100%;}.content .box {Â position: absolute;Â height: 50px;Â width: 100%;Â left: 0;Â bottom: 0;Â border-radius: 25px;Â cursor: pointer;}.share,.cancel {Â position: absolute;Â height: 100%;Â width: 100%;Â left: 0;Â top: 0;Â font-size: 20px;Â font-weight: 600;Â color: #2980b9;Â line-height: 50px;Â background: #fff;Â letter-spacing: 1px;Â border-radius: 25px;Â opacity: 0;Â transition: all 0.3s ease;}.content .box .share {Â opacity: 1;}#check:checked ~ .box .share {Â opacity: 0;}#check:checked ~ .box .cancel {Â opacity: 1;}.content .image-box {Â position: absolute;Â height: 450px;Â width: 100%;Â bottom: 130px;Â left: 50%;Â transform: translateX(-50%);Â background: #fff;Â padding: 10px;Â border-radius: 25px;Â transition: all 0.4s ease;}#check:checked ~ .image-box {Â bottom: 70px;}#check {Â display: none;}.image-box::before {Â position: absolute;Â content: "";Â bottom: -12px;Â left: 50%;Â transform: translateX(-50%) rotate(45deg);Â height: 30px;Â width: 30px;Â background: #fff;Â z-index: -1;}.image-box img {Â height: 100%;Â width: 100%;Â object-fit: cover;Â border-radius: 26px;}.image-box .about {Â position: absolute;Â background: rgba(0, 0, 0, 0.5);Â height: 100%;Â width: 100%;Â left: 0;Â top: 0;Â border-radius: 25px;Â padding: 20px;Â text-align: center;Â opacity: 0;Â transition: all 0.3s ease;}#check:checked ~ .image-box .about {Â opacity: 1;}.about .details {Â position: absolute;Â width: 100%;Â left: 0;Â bottom: 35px;}.details .name,.job {Â font-size: 18px;Â font-weight: 500;Â color: #fff;}
Step 3: Adding Animations
CSS animations bring life to your profile card. We’ll add simple sliding and hover effects for the social media icons and use a button to control the visibility of the profile card.
.details .icon i {Â font-size: 20px;Â color: #fff;Â height: 45px;Â width: 45px;Â line-height: 43px;Â border-radius: 50%;Â border: 2px solid #fff;Â margin: 14px 5px;Â transition: all 0.3s ease;}.details .icon i:hover {Â transform: scale(0.95);}
Step 4: Making the Profile Card Responsive
You’ll want to add media queries to ensure your profile card looks great on all devices. This will allow the card to resize and adjust its layout based on the screen size.
@media screen and (max-width: 768px) {.image-box .about {Â position: absolute;Â background: rgba(0, 0, 0, 0.5);Â height: 100%;Â width: 100%;Â left: 0;Â top: 0;Â border-radius: 25px;Â padding: 20px;Â text-align: center;Â opacity: 0;Â transition: all 0.3s ease;}}Want to Check Username Availability on Social Media?If you’re looking to check username availability on various social media platforms, visit NameChkr to find out!
Read Also
- Glassmorphism Login Form in HTML and CSS
Explore the stylish world of glassmorphism as you create a modern login form using HTML and CSS. This guide breaks down the design process step by step.- Toggle Button using HTML, CSS, and JavaScript
Discover how to enhance user interaction by creating a sleek toggle button with HTML, CSS, and JavaScript. This tutorial covers everything from structure to styling.- Responsive Cards in HTML and CSS
Learn how to design eye-catching responsive cards that adapt seamlessly to any device. This guide offers practical tips for achieving stunning layouts.- Build a Google Gemini Chatbot Using HTML, CSS, and JS
Dive into chatbot development by creating a Google Gemini chatbot with HTML, CSS, and JavaScript. This tutorial will help you understand the basics of interactive forms.Conclusion
Building an animated profile card using only HTML and CSS is a great way to add interactive, visually appealing elements to your website. By keeping it simple, lightweight, and responsive, you can ensure that your profile card performs well on all devices.
Whether using this card for your portfolio, business site, or personal blog, you now have a versatile and engaging way to display your profile information.
Download the Source Code
If you want to dive deeper or face any issues while creating the animated profile card, please download the complete source code below.