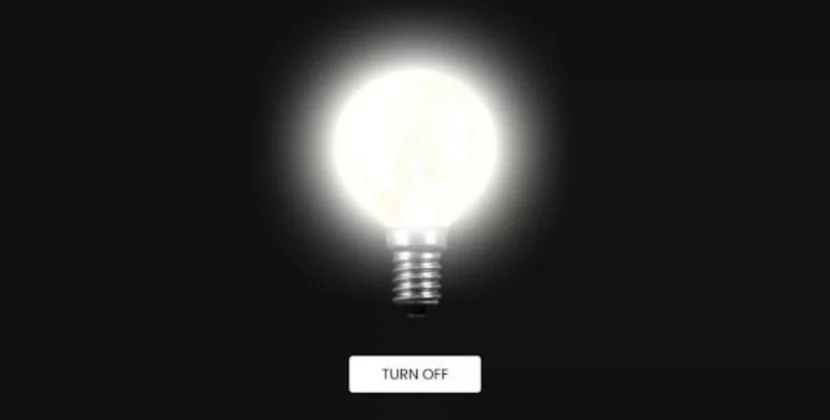
New to Web Development? Start by Building a Website with a Login and Registration Form!
If you’re just starting out in web development, creating a website with a Login and Registration Form is a perfect project to build your skills. This hands-on practice will help you master critical skills, like designing smooth navigation menus, developing homepage layouts, and writing functional form logic. It’s an ideal way to practice and learn while bringing your ideas to life!
In this guide, I will walk you through creating a responsive website that includes Login and Registration Form using HTML, CSS, and JavaScript. By completing this project, you’ll gain practical experience and learn essential web development concepts like DOM manipulation, event handling, and conditional statements.
Responsive Design with Login and Registration Form
The website’s homepage features a navigation bar and a login button. When clicked, the button reveals an attractive blurred background for the login form on the left side, with input fields on the right. If you wish to register instead, simply click the sign-up link, which takes you directly to the Registration Form.
Steps for Establishing a Website with Login and Registration Form
Follow these step-by-step instructions to create a responsive website featuring Login and Registration Form with HTML, CSS, and JavaScript:
- Create a Project Folder: Name it as you like and store the necessary files.
- Create index.html: This will be your main document.
- Create style.css: This file will contain your CSS code.
- Create script.js: This will house your JavaScript code.
- Add Images Folder: Include all the images needed for this project.
Coding the Login and Registration Form
Upload the following HTML code to your index.html file, which includes the header, nav, ul, form, and additional elements required for your project.
<!DOCTYPE html><!-- Coding By Abhikesh - www.abhikesh.com --><html lang="en"><head><meta charset="UTF-8"><meta name="viewport" content="width=device-width, initial-scale=1.0"><title>Website with Login & Signup Form | Abhikesh</title><!-- Google Fonts Link For Icons --><link rel="stylesheet"href="https://fonts.googleapis.com/css2?family=Material+Symbols+Rounded:opsz,wght,FILL,GRAD@48,400,0,0"><link rel="stylesheet" href="style.css"><script src="script.js" defer></script></head><body><header><nav class="navbar"><span class="hamburger-btn material-symbols-rounded">menu</span><a href="#" class="logo"><h2>Abhikesh</h2></a><ul class="links"><span class="close-btn material-symbols-rounded">close</span><li><a href="#">Home</a></li><li><a href="#">Portfolio</a></li><li><a href="#">Courses</a></li><li><a href="#">About us</a></li><li><a href="#">Contact us</a></li></ul><button class="login-btn">LOG IN</button></nav></header><div class="blur-bg-overlay"></div><div class="form-popup"><span class="close-btn material-symbols-rounded">close</span><div class="form-box login"><div class="form-details"><h2>Welcome Back</h2><p>Please log in using your personal information to stay connected with us.</p></div><div class="form-content"><h2>LOGIN</h2><form action="#"><div class="input-field"><input type="text" required><label>Email</label></div><div class="input-field"><input type="password" required><label>Password</label></div><a href="#" class="forgot-pass-link">Forgot password?</a><button type="submit">Log In</button></form><div class="bottom-link">Don't have an account?<a href="#" id="signup-link">Signup</a></div></div></div><div class="form-box signup"><div class="form-details"><h2>Create Account</h2><p>To become a part of our community, please sign up using your personal information.</p></div><div class="form-content"><h2>SIGNUP</h2><form action="#"><div class="input-field"><input type="text" required><label>Enter your email</label></div><div class="input-field"><input type="password" required><label>Create password</label></div><div class="policy-text"><input type="checkbox" id="policy"><label for="policy">I agree the<a href="#" class="option">Terms & Conditions</a></label></div><button type="submit">Sign Up</button></form><div class="bottom-link">Already have an account?<a href="#" id="login-link">Login</a></div></div></div></div></body></html>
Add CSS codes to your style.css document to visually style your website and forms. Experiment with various CSS properties like colors, fonts, and backgrounds to give your site a personalized touch.
/* Importing Google font - Open Sans */@import url("https://fonts.googleapis.com/css2?family=Open+Sans:wght@400;500;600;700&display=swap");* {margin: 0;padding: 0;box-sizing: border-box;font-family: "Open Sans", sans-serif;}body {height: 100vh;width: 100%;background: url("images/hero-bg.jpg") center/cover no-repeat;}header {position: fixed;width: 100%;top: 0;left: 0;z-index: 10;padding: 0 10px;}.navbar {display: flex;padding: 22px 0;align-items: center;max-width: 1200px;margin: 0 auto;justify-content: space-between;}.navbar .hamburger-btn {display: none;color: #fff;cursor: pointer;font-size: 1.5rem;}.navbar .logo {gap: 10px;display: flex;align-items: center;text-decoration: none;}.navbar .logo img {width: 40px;border-radius: 50%;}.navbar .logo h2 {color: #fff;font-weight: 600;font-size: 1.7rem;}.navbar .links {display: flex;gap: 35px;list-style: none;align-items: center;}.navbar .close-btn {position: absolute;right: 20px;top: 20px;display: none;color: #000;cursor: pointer;}.navbar .links a {color: #fff;font-size: 1.1rem;font-weight: 500;text-decoration: none;transition: 0.1s ease;}.navbar .links a:hover {color: #19e8ff;}.navbar .login-btn {border: none;outline: none;background: #fff;color: #275360;font-size: 1rem;font-weight: 600;padding: 10px 18px;border-radius: 3px;cursor: pointer;transition: 0.15s ease;}.navbar .login-btn:hover {background: #ddd;}.form-popup {position: fixed;top: 50%;left: 50%;z-index: 10;width: 100%;opacity: 0;pointer-events: none;max-width: 720px;background: #fff;border: 2px solid #fff;transform: translate(-50%, -70%);}.show-popup .form-popup {opacity: 1;pointer-events: auto;transform: translate(-50%, -50%);transition: transform 0.3s ease, opacity 0.1s;}.form-popup .close-btn {position: absolute;top: 12px;right: 12px;color: #878484;cursor: pointer;}.blur-bg-overlay {position: fixed;top: 0;left: 0;z-index: 10;height: 100%;width: 100%;opacity: 0;pointer-events: none;backdrop-filter: blur(5px);-webkit-backdrop-filter: blur(5px);transition: 0.1s ease;}.show-popup .blur-bg-overlay {opacity: 1;pointer-events: auto;}.form-popup .form-box {display: flex;}.form-box .form-details {width: 100%;color: #fff;max-width: 330px;text-align: center;display: flex;flex-direction: column;justify-content: center;align-items: center;}.login .form-details {padding: 0 40px;background: url("images/login-img.jpg");background-position: center;background-size: cover;}.signup .form-details {padding: 0 20px;background: url("images/signup-img.jpg");background-position: center;background-size: cover;}.form-box .form-content {width: 100%;padding: 35px;}.form-box h2 {text-align: center;margin-bottom: 29px;}form .input-field {position: relative;height: 50px;width: 100%;margin-top: 20px;}.input-field input {height: 100%;width: 100%;background: none;outline: none;font-size: 0.95rem;padding: 0 15px;border: 1px solid #717171;border-radius: 3px;}.input-field input:focus {border: 1px solid #00bcd4;}.input-field label {position: absolute;top: 50%;left: 15px;transform: translateY(-50%);color: #4a4646;pointer-events: none;transition: 0.2s ease;}.input-field input:is(:focus, :valid) {padding: 16px 15px 0;}.input-field input:is(:focus, :valid)~label {transform: translateY(-120%);color: #00bcd4;font-size: 0.75rem;}.form-box a {color: #00bcd4;text-decoration: none;}.form-box a:hover {text-decoration: underline;}form :where(.forgot-pass-link, .policy-text) {display: inline-flex;margin-top: 13px;font-size: 0.95rem;}form button {width: 100%;color: #fff;border: none;outline: none;padding: 14px 0;font-size: 1rem;font-weight: 500;border-radius: 3px;cursor: pointer;margin: 25px 0;background: #00bcd4;transition: 0.2s ease;}form button:hover {background: #0097a7;}.form-content .bottom-link {text-align: center;}.form-popup .signup,.form-popup.show-signup .login {display: none;}.form-popup.show-signup .signup {display: flex;}.signup .policy-text {display: flex;margin-top: 14px;align-items: center;}.signup .policy-text input {width: 14px;height: 14px;margin-right: 7px;}@media (max-width: 950px) {.navbar :is(.hamburger-btn, .close-btn) {display: block;}.navbar {padding: 15px 0;}.navbar .logo img {display: none;}.navbar .logo h2 {font-size: 1.4rem;}.navbar .links {position: fixed;top: 0;z-index: 10;left: -100%;display: block;height: 100vh;width: 100%;padding-top: 60px;text-align: center;background: #fff;transition: 0.2s ease;}.navbar .links.show-menu {left: 0;}.navbar .links a {display: inline-flex;margin: 20px 0;font-size: 1.2rem;color: #000;}.navbar .links a:hover {color: #00BCD4;}.navbar .login-btn {font-size: 0.9rem;padding: 7px 10px;}}@media (max-width: 760px) {.form-popup {width: 95%;}.form-box .form-details {display: none;}.form-box .form-content {padding: 30px 20px;}}
Once the styles are applied, load your website in the browser. While the forms may initially be hidden, they will become visible later through JavaScript.
Finally, add JavaScript code to your script.js file. This code includes click event listeners, allowing classes to be toggled on various HTML elements.
const navbarMenu = document.querySelector(".navbar .links");const hamburgerBtn = document.querySelector(".hamburger-btn");const hideMenuBtn = navbarMenu.querySelector(".close-btn");const showPopupBtn = document.querySelector(".login-btn");const formPopup = document.querySelector(".form-popup");const hidePopupBtn = formPopup.querySelector(".close-btn");const signupLoginLink = formPopup.querySelectorAll(".bottom-link a");// Show mobile menuhamburgerBtn.addEventListener("click", () => {navbarMenu.classList.toggle("show-menu");});// Hide mobile menuhideMenuBtn.addEventListener("click", () => hamburgerBtn.click());// Show login popupshowPopupBtn.addEventListener("click", () => {document.body.classList.toggle("show-popup");});// Hide login popuphidePopupBtn.addEventListener("click", () => showPopupBtn.click());// Show or hide signup formsignupLoginLink.forEach(link => {link.addEventListener("click", (e) => {e.preventDefault();formPopup.classList[link.id === 'signup-link' ? 'add' : 'remove']("show-signup");});});
Read Also
- Glassmorphism Login Form in HTML and CSS
Explore the stylish world of glassmorphism as you create a modern login form using HTML and CSS. This guide breaks down the design process step by step. - Toggle Button using HTML, CSS, and JavaScript
Discover how to enhance user interaction by creating a sleek toggle button with HTML, CSS, and JavaScript. This tutorial covers everything from structure to styling. - Responsive Cards in HTML and CSS
Learn how to design eye-catching responsive cards that adapt seamlessly to any device. This guide offers practical tips for achieving stunning layouts. - Build a Google Gemini Chatbot Using HTML, CSS, and JS
Dive into chatbot development by creating a Google Gemini chatbot with HTML, CSS, and JavaScript. This tutorial will help you understand the basics of interactive forms.
Conclusion
Creating a website with Login and Registration Form is an engaging and hands-on learning experience that explores various web development components. Following the steps outlined in this post, you should have successfully created your login and registration Form using HTML, CSS, and JavaScript.
Brilliant explanation! I’ve been searching for a comprehensive tutorial on form validation, and this is by far the best I’ve found. Thank you for sharing your expertise!
Great tutorial! I appreciate how you covered the basics as well as more advanced techniques. Form validation is such an important aspect of web design, and now I feel equipped to handle it effectively.
Wow, this tutorial is exactly what I needed. I was always intimidated by JavaScript, but your explanations made it much more approachable. Now I can create robust forms with confidence!
Thank you for sharing this tutorial! I’ve been struggling with form validation for a while, but your guidance made it much simpler. Can’t wait to apply this knowledge to my website.
Excellent tutorial! Clear explanations and practical examples made it easy to follow along. Form validation used to be a headache, but now I feel confident implementing it in my projects.
This tutorial was a lifesaver! Finally, I understand how to implement form validation seamlessly across HTML, CSS, and JavaScript. Thanks for breaking it down step by step!
Fantastic guide! Your tutorial on creating a login/registration form using HTML, CSS, and JavaScript was both comprehensive and easy to understand. The detailed steps for form design, validation, and interactivity were particularly helpful. This resource is perfect for anyone looking to build functional and stylish web forms. Thanks for the excellent instructions and examples!
Awesome tutorial! Your step-by-step guide on creating a login/registration form with HTML, CSS, and JavaScript was incredibly useful. The explanations on integrating form validation and styling were spot-on. This will definitely be a great resource for enhancing my web development skills. Thanks for providing such clear and practical instructions!
Outstanding tutorial! Your guide on creating a login/registration form with HTML, CSS, and JavaScript was incredibly clear and informative. The attention to both design and functionality aspects is very much appreciated. This tutorial has provided me with a solid foundation for implementing secure and stylish forms on my own websites. Thanks for the valuable insights and practical examples!
Excellent guide! Your tutorial on creating a login/registration form using HTML, CSS, and JavaScript was thorough and easy to follow. The step-by-step approach and clear explanations of form functionality and design made it very practical. This will definitely help me build better web forms for my projects. Thanks for the great insights and detailed instructions!
Great tutorial! Your step-by-step guide to creating a login/registration form using HTML, CSS, and JavaScript was incredibly helpful. The explanations on form validation, styling, and interactivity were spot-on. This is exactly what I needed to enhance my web development skills. Thanks for providing such a comprehensive and easy-to-follow resource!
Fantastic tutorial! Your guide on building a login/registration form with HTML, CSS, and JavaScript was very well-explained and practical. I appreciated the detailed coverage of both the frontend and backend aspects, as well as the tips for enhancing form security and user experience. This will be incredibly useful for my projects. Thank you for the clear and insightful instructions!
Amazing tutorial! Your guide on creating a login/registration form using HTML, CSS, and JavaScript was both detailed and easy to understand. The focus on both functionality and design aspects made it very comprehensive. This will definitely be a great resource for building secure and attractive web forms. Thanks for sharing such valuable insights!
Great tutorial! Your step-by-step guide on creating a login/registration form using HTML, CSS, and JavaScript was incredibly clear and easy to follow. The sections on form validation and user interface design were especially helpful. This resource will be invaluable for anyone looking to build functional and visually appealing web forms. Thanks for the fantastic guide!
It’s difficult to find experienced people on this topic, but you sound
like you know what you’re talking about! Thanks
Excellent tutorial! Your detailed approach to building a login/registration form using HTML, CSS, and JavaScript was both informative and easy to follow. The insights on user authentication and responsive design were especially valuable. This guide will be a great asset for developing secure and interactive web forms. Thanks for the detailed instructions!
Fantastic tutorial! Your guide on creating a login/registration form with HTML, CSS, and JavaScript was extremely clear and detailed. The explanations on styling and validation were particularly helpful. This tutorial will definitely aid in building effective and user-friendly web forms. Thank you for providing such a comprehensive resource!
Amazing tutorial! Your step-by-step guide on building a login/registration form with HTML, CSS, and JavaScript was incredibly thorough and easy to follow. I found the sections on form validation and design to be particularly useful. This resource is perfect for anyone looking to create a sleek and functional web form. Thanks for sharing such valuable insights!
Excellent guide! Your tutorial on creating a login/registration form using HTML, CSS, and JavaScript was incredibly detailed and easy to understand. The tips on form validation and responsive design were especially helpful. This is a fantastic resource for anyone looking to build functional and stylish web forms. Thanks for the great content!
Wonderful tutorial! Your guide on building a login/registration form with HTML, CSS, and JavaScript was both detailed and easy to follow. The explanations on validation and responsive design were particularly useful. This will definitely help me in creating more dynamic and secure web forms. Thanks for sharing such a comprehensive resource!
Simply wish to say your article is as surprising. The clarity
to your put up is simply nice and i could suppose you are knowledgeable in this subject.
Well along with your permission allow me to clutch your feed to stay up to date with
impending post. Thanks one million and please continue the
rewarding work.
Fantastic guide! Your tutorial on creating a login/registration form using HTML, CSS, and JavaScript was incredibly insightful. The step-by-step instructions and tips on form validation and styling were very helpful. This is a great resource for anyone looking to build a robust and user-friendly login system. Thanks for the excellent work!
Great tutorial! Your detailed steps for creating a login/registration form with HTML, CSS, and JavaScript were incredibly helpful. I particularly appreciated the insights on form validation and user interface design. This guide will be a valuable reference for my future projects. Thanks for making it so easy to understand!
Outstanding tutorial! Your guide on building a login/registration form using HTML, CSS, and JavaScript was very thorough and easy to follow. The explanations on form validation and user experience improvements were particularly useful. This will be a great resource for my web development work. Thanks for the clear and practical insights!
Incredible guide! Your detailed walkthrough of creating a login/registration form with HTML, CSS, and JavaScript was both clear and comprehensive. The tips on form validation and responsive design were especially valuable. This tutorial will definitely help me in building a sleek and functional login system. Thanks for sharing such practical advice!
Whoa! This blog looks just like my old one!
It’s on a entirely different subject but it has pretty much
the same page layout and design. Excellent choice of colors!
Excellent tutorial! Your step-by-step guide on creating a login/registration form with HTML, CSS, and JavaScript was very informative. The insights on both frontend styling and form validation were particularly useful. This will be a great asset for my web development projects. Thanks for the detailed and practical instructions!
Fantastic tutorial! Your guide on creating a login/registration form using HTML, CSS, and JavaScript was extremely helpful. The explanations on JavaScript validation and CSS styling were clear and practical. This is a great resource for anyone looking to build robust and attractive web forms. Thanks for the thorough and easy-to-follow instructions!
Awesome guide! Your detailed explanation of creating a login/registration form with HTML, CSS, and JavaScript was incredibly useful. The insights on form validation and responsive design were particularly helpful. This tutorial is perfect for anyone looking to build a sleek and functional login system. Thanks for sharing such valuable information!
Awesome tutorial! Your step-by-step guide on creating a login/registration form using HTML, CSS, and JavaScript was incredibly helpful. The explanations on form validation and styling were very clear and practical. This will be a great resource for my upcoming projects. Thanks for the detailed and well-structured instructions!
Excellent guide! The instructions for creating a login/registration form using HTML, CSS, and JavaScript were clear and detailed. I particularly appreciated the tips on JavaScript validation and CSS styling. This tutorial is a fantastic resource for building functional and stylish forms. Thanks for the practical and well-explained content!”
Excellent tutorial! The step-by-step instructions for building a login/registration form with HTML, CSS, and JavaScript were very well presented. I found the JavaScript validation tips and CSS design ideas particularly useful. This guide will definitely help me in creating functional and visually appealing forms. Thanks for the clear and practical advice!
Great tutorial! Your guide on creating a login/registration form with HTML, CSS, and JavaScript was incredibly clear and comprehensive. The explanations on handling form validation with JavaScript and styling with CSS were especially helpful. This will be a valuable resource for my web development projects. Thanks for sharing such a well-structured guide!
Awesome tutorial! Your step-by-step guide to creating a login/registration form with HTML, CSS, and JavaScript was incredibly useful. The explanations on JavaScript validation and CSS styling were particularly insightful. This guide is perfect for anyone looking to build a robust and user-friendly form. Thanks for the detailed and practical advice!
Excellent guide! The tutorial on building a login/registration form using HTML, CSS, and JavaScript was thorough and easy to follow. The JavaScript validation and CSS styling tips were particularly useful. This will definitely help in developing functional and attractive web forms. Thanks for the detailed and practical advice!
Fantastic tutorial! Your step-by-step guide on creating a login/registration form with HTML, CSS, and JavaScript was incredibly helpful. The clear explanations of JavaScript validation and CSS design tips were especially useful. This is a great resource for anyone looking to enhance their web development skills. Thanks for the comprehensive and practical guide!
Wonderful tutorial! The instructions for creating a login/registration form using HTML, CSS, and JavaScript were very clear and easy to follow. I especially liked the detailed explanations on JavaScript validation and CSS styling. This guide is perfect for anyone looking to build a robust login system. Thanks for the excellent and practical advice!
Fantastic guide! The step-by-step instructions for building a login/registration form using HTML, CSS, and JavaScript were extremely helpful. I appreciated the clear explanations on JavaScript validation and the CSS styling tips. This tutorial is a great resource for anyone looking to improve their web development skills. Thanks for the insightful and practical guide!
Excellent tutorial! Your guide to creating a login/registration form with HTML, CSS, and JavaScript was very clear and detailed. The JavaScript validation and CSS styling tips were especially helpful. This will definitely be a great resource for my upcoming projects. Thanks for the comprehensive and easy-to-follow instructions!
Amazing guide! The step-by-step instructions for creating a login/registration form using HTML, CSS, and JavaScript were incredibly helpful. I especially liked the detailed explanations on JavaScript validation and CSS styling. This will definitely enhance my web development skills. Thanks for the comprehensive and practical tutorial!
Fantastic tutorial! The guide on building a login/registration form with HTML, CSS, and JavaScript was very detailed and easy to follow. I especially appreciated the practical tips on JavaScript validation and CSS styling. This will be a great asset for my web development projects. Thanks for the clear and useful instructions!
Fantastic tutorial! The comprehensive guide on creating a login/registration form with HTML, CSS, and JavaScript was incredibly helpful. The detailed explanations on JavaScript validation and CSS styling are just what I needed. This will be a great resource for my web development work. Thanks for the excellent instructions!
Great tutorial! The step-by-step instructions for creating a login/registration form using HTML, CSS, and JavaScript were very clear and practical. I found the JavaScript validation tips particularly useful. This guide will definitely help with my web development projects. Thanks for providing such a thorough and easy-to-follow resource!
Great tutorial! The guide on creating a login/registration form using HTML, CSS, and JavaScript was very well-structured and easy to follow. I found the JavaScript validation techniques especially helpful. This will be a valuable resource for my web development projects. Thanks for providing such clear and practical instructions!
Fantastic guide! The step-by-step instructions for creating a login/registration form with HTML, CSS, and JavaScript were incredibly clear and easy to follow. I especially appreciated the detailed explanations of JavaScript validation and CSS styling. This will be a valuable resource for my web development projects. Thanks for sharing such a practical and comprehensive tutorial!
Excellent tutorial! The guide on creating a login/registration form using HTML, CSS, and JavaScript was very detailed and easy to understand. I particularly appreciated the clear explanations on JavaScript validation and CSS design. This resource will be invaluable for my web development projects. Thank you for the thorough and practical instructions!
Great tutorial! Your step-by-step approach to building a login/registration form with HTML, CSS, and JavaScript was incredibly helpful. I found the explanations on JavaScript validation and CSS customization particularly useful. This guide will definitely improve my web development skills. Thanks for the clear and practical instructions!
Amazing tutorial! The detailed guide on creating a login/registration form using HTML, CSS, and JavaScript was very informative and easy to follow. The JavaScript validation and CSS styling tips were particularly helpful. This will be a fantastic resource for my web development projects. Thanks for sharing such a comprehensive and practical guide!
Fantastic tutorial! The step-by-step guide on creating a login/registration form with HTML, CSS, and JavaScript was very well-structured and easy to follow. I especially appreciated the tips on JavaScript validation and CSS styling. This will be a great resource for my web development projects. Thanks for the detailed instructions!
Fantastic tutorial! The instructions for creating a login/registration form with HTML, CSS, and JavaScript were very detailed and easy to follow. I found the JavaScript validation techniques particularly useful. This guide will definitely be a great resource for my upcoming projects. Thanks for the clear and practical advice!
Great guide! The tutorial on creating a login/registration form with HTML, CSS, and JavaScript was clear and easy to follow. I found the JavaScript validation examples particularly useful. This will definitely help me enhance my web development skills. Thanks for the detailed and practical instructions!
This tutorial is fantastic! The comprehensive guide on building a login/registration form with HTML, CSS, and JavaScript was easy to follow and very informative. I especially appreciated the sections on JavaScript validation and CSS design tips. This will be a valuable resource for my upcoming projects. Thanks for the clear and practical instructions!
Fantastic tutorial! The step-by-step guide to creating a login/registration form using HTML, CSS, and JavaScript was incredibly clear and practical. I especially appreciated the tips on JavaScript validation and CSS styling. This guide will be immensely helpful for my web development projects. Thanks for the detailed instructions!
This is an outstanding tutorial! The step-by-step approach to creating a login/registration form using HTML, CSS, and JavaScript was very thorough and easy to follow. I found the JavaScript validation examples particularly useful. This guide will be a valuable resource for my web development projects. Thanks for sharing such a practical and detailed tutorial!
This tutorial is incredibly helpful! The step-by-step approach to creating a login/registration form using HTML, CSS, and JavaScript was clear and well-structured. I particularly appreciated the JavaScript validation tips and the CSS styling details. This guide will definitely help me with my web development projects. Thanks for the excellent resource!
Fantastic tutorial! The clear instructions for building a login/registration form with HTML, CSS, and JavaScript were incredibly helpful. I especially liked the JavaScript validation and the detailed CSS styling tips. This guide will be a great resource for my web development projects. Thanks for making it so accessible and easy to follow!
This guide is excellent! The step-by-step process for creating a login/registration form using HTML, CSS, and JavaScript was very detailed and easy to understand. I particularly appreciated the tips on JavaScript validation and CSS styling. This will definitely enhance my web development skills. Thanks for sharing such a practical and insightful tutorial!
This tutorial is fantastic! The step-by-step guide on creating a login/registration form using HTML, CSS, and JavaScript was very clear and thorough. I found the JavaScript validation tips especially useful. This will be a great resource for my upcoming projects. Thanks for the detailed and practical guide!
Fantastic tutorial! The step-by-step approach to creating a login/registration form using HTML, CSS, and JavaScript was very well explained. I found the JavaScript validation techniques particularly useful. This guide will be a great help for my web development projects. Thanks for the clear and practical instructions!
This is a superb guide! The comprehensive instructions for building a login/registration form using HTML, CSS, and JavaScript were very clear and practical. I especially appreciated the JavaScript validation tips and CSS styling suggestions. This will be a valuable resource for my project. Thanks for the detailed tutorial!
This tutorial is fantastic! The step-by-step approach to building a login/registration form using HTML, CSS, and JavaScript was very easy to follow. The detailed explanations and practical tips were incredibly useful. I’m looking forward to using these techniques on my own site. Thanks for the thorough guide!
This is an excellent tutorial! The guide to building a login/registration form with HTML, CSS, and JavaScript was very clear and comprehensive. I especially appreciated the tips on JavaScript validation and CSS styling. This will be incredibly useful for my next project. Thanks for sharing such a practical resource!
This guide is incredibly helpful! The step-by-step instructions for creating a login/registration form using HTML, CSS, and JavaScript were very clear and practical. I especially appreciated the JavaScript validation and styling tips. This will definitely improve my website’s functionality. Thanks for the excellent tutorial!
This tutorial is fantastic! The detailed steps for creating a login/registration form using HTML, CSS, and JavaScript were very clear and easy to follow. I especially liked the section on JavaScript validation—it made the process much smoother. Thanks for sharing such a practical and comprehensive guide!
This guide is incredibly useful! The step-by-step instructions for building a login/registration form were clear and detailed. I especially appreciated the JavaScript validation examples and CSS styling tips. This will be a great addition to my website. Thanks for the excellent tutorial!
This tutorial is incredibly helpful! The detailed guide on creating a login/registration form using HTML, CSS, and JavaScript was easy to follow and well-explained. I especially found the JavaScript validation tips useful. Thanks for providing such a comprehensive and practical resource!
This tutorial is incredibly useful! The step-by-step process for creating a login/registration form was well-explained and easy to follow. I especially appreciated the detailed explanations for the JavaScript functionality and CSS styling. This will definitely help me improve my website. Thanks for sharing!
This guide is fantastic! The detailed explanation of creating a login/registration form using HTML, CSS, and JavaScript was incredibly helpful. The step-by-step approach made it easy to follow, and the JavaScript validation tips were especially useful. Thanks for providing such a clear and practical tutorial!
This tutorial is incredibly helpful! The detailed breakdown of building a login/registration form using HTML, CSS, and JavaScript was very clear. I especially liked the section on JavaScript validation—it really helps in ensuring data accuracy. Thanks for providing such a comprehensive and easy-to-follow guide!
Fantastic guide! The step-by-step approach to creating a login/registration form was very straightforward and easy to follow. I appreciated the detailed explanations for HTML, CSS, and JavaScript. This will definitely help in building a functional and stylish form for my projects. Thanks for sharing!
This is a fantastic tutorial! The step-by-step guide to creating a login/registration form was incredibly helpful. The integration of HTML, CSS, and JavaScript was explained clearly, making it easy to follow. I’m excited to use these techniques in my own projects. Thank you for the detailed instructions!
This is an amazing guide! Building a login/registration form can be tricky, but your clear instructions and code examples made it so much easier. I particularly appreciated the tips on integrating JavaScript validation. This will definitely help me enhance my website. Thanks for the detailed walkthrough!
This guide is incredibly useful! The detailed explanation of setting up a login/registration form using HTML, CSS, and JavaScript was very clear and practical. I especially appreciated the JavaScript validation examples—great for ensuring a smooth user experience. Thanks for providing such a comprehensive tutorial!
Fantastic guide! The step-by-step instructions for building a login/registration form were very easy to follow. I found the HTML structure and CSS styling tips particularly helpful. The JavaScript validation examples were spot-on. Thanks for providing such a comprehensive tutorial!
This tutorial is incredibly useful! The detailed breakdown of creating a login/registration form using HTML, CSS, and JavaScript was very clear. I especially liked the section on JavaScript validation—it’s a crucial part of the process. Thanks for sharing this easy-to-follow guide!
This tutorial is fantastic! The step-by-step approach made it really easy to build the login/registration form. The CSS styling tips are great for making the form look polished. The JavaScript validation examples were also very useful. Thanks for sharing such a detailed guide!
This is an excellent guide for creating a login/registration form! The clear explanations and code examples are very helpful. I particularly appreciated the JavaScript validation tips—very useful for ensuring a smooth user experience. Looking forward to more tutorials like this!
Discover how to build a website with robust login and registration functionality using HTML, CSS, and JavaScript. This guide covers creating and styling forms, implementing client-side validation, and ensuring a responsive design. Perfect for developers looking to enhance their front-end skills and add essential user authentication features to their websites.
Learn how to develop a fully functional website with both login and registration forms using HTML, CSS, and JavaScript. This tutorial walks you through building secure, responsive forms with real-time validation, ensuring a smooth user experience. Perfect for anyone looking to add authentication features to their website!
Such a comprehensive guide! The way you’ve detailed every step to design a stunning login and registration form is impressive. It’s perfect for anyone wanting to create a sleek user interface. Thanks for providing so much clarity and inspiration!