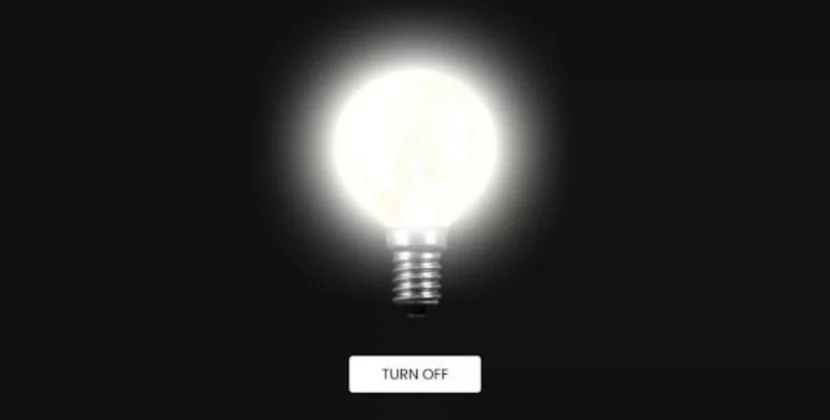
Creating a Random Password Generator App in HTML and CSS is a practical project for beginners and professionals looking to enhance their web development skills. Password security is vital in today’s digital landscape, and building such an app provides users with strong, secure, and randomly generated passwords. This blog will guide you through creating a sleek, user-friendly design using only HTML and CSS without relying on JavaScript.
What is a Random Password Generator?
A Random Password Generator App is a tool that generates secure passwords by combining a mix of characters, including:
- Uppercase and lowercase alphabets.
- Numbers.
- Special characters such as @, #, and !.
This functionality ensures the creation of complex passwords that are difficult to guess, enhancing online security for users.
Features of the Random Password Generator App
- User-Friendly Interface: Clean design with a simple layout for seamless navigation.
- Responsive Design: Fully functional across devices like desktops, tablets, and smartphones.
- Secure Password Generation: Creates passwords with alphanumeric and special characters.
- Copy Functionality: Easy-to-use copy button for pasting passwords elsewhere.
- Customizable Design: Easily tweak the app’s appearance to suit your brand or personal preference.
Benefits of Using a Random Password Generator
Using a Random Password Generator App provides several advantages:
1. Enhanced Security
Robust passwords help minimize the chances of hacking and cybersecurity threats. A random password generator ensures that passwords do not have predictable patterns.
2. Saves Time
Creating a secure password by hand can be time-consuming and difficult. This app generates passwords instantly, saving you Time and effort.
3. Convenience
With built-in features like the copy button, you can easily store or share your password without retyping it.
4. Password Complexity
The generated passwords include combinations that adhere to the best password security practices, making them highly reliable.
Steps to Create a Random Password Generator App using HTML and CSS
While this blog focuses on using HTML and CSS, a typical implementation of a random password generator also includes JavaScript for dynamic functionality. However, we will limit the scope to designing a functional and visually appealing layout.
Step 1: Designing the App Layout
The layout for the Random Password Generator App should include:
- Password Display Field: An input box to showcase the generated password.
- Copy Feature: A button that copies the generated password to your clipboard.
- Generate Button: A button to generate a random password.
Step 2: Adding Visual Appeal with CSS
CSS is essential for enhancing the app’s visual design and overall appeal. Key considerations include:
- Color Scheme: Use a neutral and professional palette, such as white backgrounds with blue and green accents.
- Responsive Design: Ensure the app adjusts seamlessly to different screen sizes.
- Hover Effects: Enhance the buttons with hover animations to improve interactivity.
Step 1: Designing the Layout with HTML
First, create the foundational structure of the app using HTML,CSS and JS. The layout should include:
- A password display field will be created to showcase the generated password.
- A button that allows users to quickly copy the generated password to their clipboard.
- Another button to create the password.
Here’s an example of the HTML code:
<!DOCTYPE html><!-- Created By abhikesh.com --><html lang="en" dir="ltr"><head><meta charset="utf-8"><title>Random Password Generator | abhikesh kumar</title><link rel="stylesheet" href="style.css"><link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.3/css/all.min.css" /></head><body><div class="container"><div class="text">Random Password Generator <br>in HTML CSS & JavaScript</div><div class="input-data"><div class="display"><input type="text"><span class="far fa-copy" onclick="copy()"></span><span class="fas fa-copy" onclick="copy()"></span></div><button>Generate Password</button></div></div><script>const display = document.querySelector("input"),button = document.querySelector("button"),copyBtn = document.querySelector("span.far"),copyActive = document.querySelector("span.fas");let chars = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789!@#$%^&*()_+~`|}{[]:;?><,./-=";button.onclick = () => {let i,randomPassword = "";copyBtn.style.display = "block";copyActive.style.display = "none";for (i = 0; i < 16; i++) {randomPassword = randomPassword + chars.charAt(Math.floor(Math.random() * chars.length));}display.value = randomPassword;}function copy() {copyBtn.style.display = "none";copyActive.style.display = "block";display.select();document.execCommand("copy");}</script></body></html>
Step 2: Styling the App with CSS
Once the HTML is ready, enhance the app’s appearance using CSS. Your CSS should include:
- A professional color scheme (e.g., light background with accent colors).
- Responsiveness for all device sizes.
- Hover effects to improve interactivity.
Here’s an example of the CSS code:
<style>@import url('https://fonts.googleapis.com/css?family=Poppins:400,500,600,700&display=swap');* {margin: 0;padding: 0;box-sizing: border-box;font-family: 'Poppins', sans-serif;}html,body {display: grid;height: 100%;place-items: center;background: #000;text-align: center;}.container {width: 450px;background: #ff014f;border: 1px solid #444;border-radius: 5px;padding: 20px 25px;}.container .text {color: #fff;font-weight: 600;font-size: 26px;line-height: 35px;}.container .input-data {margin: 20px 5px 15px 5px;}.input-data .display {height: 45px;width: 100%;display: flex;position: relative;}.input-data .display input {height: 100%;width: 100%;outline: none;color: #eee;border: 1px solid #333;background: #222;padding: 10px;font-size: 17px;pointer-events: none;user-select: none;}.input-data .display span {position: absolute;right: 15px;top: 50%;transform: translateY(-50%);font-size: 25px;color: #fff;z-index: 999;display: none;cursor: pointer;}.input-data button {display: block;height: 45px;width: 100%;margin-top: 15px;border: 1px solid #444;outline: none;background: #1b1b1b;color: #fff;font-size: 17px;font-weight: 500;text-transform: uppercase;cursor: pointer;transition: all 0.3s ease;}.input-data button:hover {background: #222;}</style>
SEO Tips for Optimizing Your Password Generator App
When creating your Random Password Generator App in HTML and CSS, it’s essential to make the content SEO-friendly to attract organic traffic. Here are some tips:
- Focus Keyword Usage:
- Start the blog with the focus keyword: Random Password Generator App in HTML and CSS.
- Use it naturally throughout the content, including subheadings and image alt text.
- Meta Description:
- Image Optimization:
- Use descriptive filenames like random-password-generator-html-css.png.
- Add alt tags such as: “Random Password Generator App designed with HTML and CSS for secure passwords.”
- Readability:
Write a concise, keyword-rich meta description. For example:
Discover how to build a stylish Random Password Generator App using HTML and CSS. Improve your web development abilities through this detailed guide. Perfect for secure password generation.”
Break down the content into short paragraphs and use subheadings to improve user experience.
Read Also
- Glassmorphism Login Form in HTML and CSS
Explore the stylish world of glassmorphism as you create a modern login form using HTML and CSS. This guide breaks down the design process step by step. - Toggle Button using HTML, CSS, and JavaScript
Discover how to enhance user interaction by creating a sleek toggle button with HTML, CSS, and JavaScript. This tutorial covers everything from structure to styling. - Responsive Cards in HTML and CSS
Learn how to design eye-catching responsive cards that adapt seamlessly to any device. This guide offers practical tips for achieving stunning layouts. - Build a Google Gemini Chatbot Using HTML, CSS, and JS
Dive into chatbot development by creating a Google Gemini chatbot with HTML, CSS, and JavaScript. This tutorial will help you understand the basics of interactive forms.
Conclusion
A Random Password Generator App in HTML and CSS is a valuable addition to any web developer’s portfolio. It showcases design skills and the ability to create practical tools. By following this guide, you’ll build an appealing app and optimize your content for search engines, driving organic traffic to your site.
For the complete source code, click the button below: