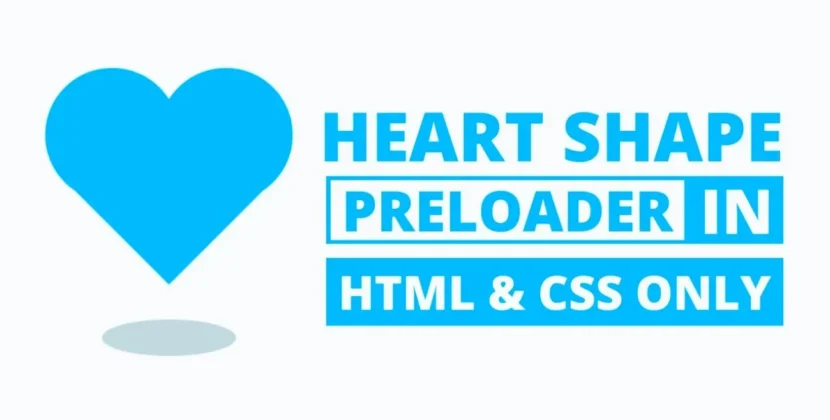
Are you looking for an elegant, modern, and transparent login form that you can quickly implement using HTML, CSS, and a little JavaScript? This step-by-step guide will walk you through creating a transparent login form design that’s lightweight, responsive, and visually stunning. It’s a great way to offer a sleek user experience while keeping your website fast and SEO-friendly.
What is a Transparent Login Form?
A transparent login form is a visually appealing input form where elements like the background or form fields have reduced opacity, giving a glass-like effect. This design is perfect for modern websites prioritizing minimalism and aesthetics, particularly for login pages, registration forms, and even portfolio or business websites. With just HTML, CSS, and minimal JavaScript for functionality, you can create a login form that stands out and adds a professional touch to your site.
Why Use Transparent Login Forms?
Transparent login forms offer more than just a great look—they also provide a user-friendly interface. This type of design is commonly used across many websites because:
- They focus on the user input fields while blending beautifully with the background.
- The design is lightweight, keeping your website’s performance optimized.
- It’s highly customizable, meaning you can easily change the background, text, and form details to fit your site’s theme.
Using only HTML and CSS, you can achieve a seamless login experience without relying on heavy frameworks, ensuring your website remains responsive and SEO-optimized.
Key Features of the Transparent Login Form
This transparent login form design includes the following:
- Transparent background that provides a sleek, glass-like appearance.
- Username and password fields with focus animations for a clean, professional look.
- Show/hide password toggle functionality using JavaScript for better user experience.
- Social media icons that can link to popular platforms like Facebook, Twitter, or LinkedIn.
- Fully responsive design that adapts to different screen sizes, ensuring mobile-friendly accessibility.
Step-by-Step Guide to Create a Transparent Login Form
Step 1: Building the HTML Structure
To start, create a basic HTML structure for the form. The form should contain input fields for the username, password, and a submit button. Here’s an example:
<!DOCTYPE html><html lang="en" dir="ltr"> <head>  <meta charset="utf-8">  <title>Transparent Login Form UI</title>  <link rel="stylesheet" href="style.css">  <script src="https://kit.fontawesome.com/a076d05399.js"></script> </head> <body>  <div class="bg-img">   <div class="content">    <header>Login Form</header>    <form action="#">     <div class="field">      <span class="fa fa-user"></span>      <input type="text" required placeholder="Email or Phone">     </div>     <div class="field space">      <span class="fa fa-lock"></span>      <input type="password" class="pass-key" required placeholder="Password">      <span class="show">SHOW</span>     </div>     <div class="pass">      <a href="#">Forgot Password?</a>     </div>     <div class="field">      <input type="submit" value="LOGIN">     </div>    </form>    <div class="login">Or login with</div>    <div class="links">     <div class="facebook">      <i class="fab fa-facebook-f"><span>Facebook</span></i>     </div>     <div class="instagram">      <i class="fab fa-instagram"><span>Instagram</span></i>     </div>    </div>    <div class="signup">Don't have account?     <a href="#">Signup Now</a>    </div>   </div>  </div> </body></html>
Step 2: Styling with CSS
Now, it’s time to make the form look stylish using CSS. You’ll use properties like opacity for the transparent effect and border-radius for smooth edges. Here’s how you can style it:
@import url('https://fonts.googleapis.com/css?family=Montserrat:400,500,600,700|Poppins:400,500&display=swap');*{Â margin: 0;Â padding: 0;Â box-sizing: border-box;Â user-select: none;}.bg-img{Â background: url('bg.jpg');Â height: 100vh;Â background-size: cover;Â background-position: center;}.bg-img:after{Â position: absolute;Â content: '';Â top: 0;Â left: 0;Â height: 100%;Â width: 100%;Â background: rgba(0,0,0,0.7);}.content{Â position: absolute;Â top: 50%;Â left: 50%;Â z-index: 999;Â text-align: center;Â padding: 60px 32px;Â width: 370px;Â transform: translate(-50%,-50%);Â background: rgba(255,255,255,0.04);Â box-shadow: -1px 4px 28px 0px rgba(0,0,0,0.75);}.content header{Â color: white;Â font-size: 33px;Â font-weight: 600;Â margin: 0 0 35px 0;Â font-family: 'Montserrat',sans-serif;}.field{Â position: relative;Â height: 45px;Â width: 100%;Â display: flex;Â background: rgba(255,255,255,0.94);}.field span{Â color: #222;Â width: 40px;Â line-height: 45px;}.field input{Â height: 100%;Â width: 100%;Â background: transparent;Â border: none;Â outline: none;Â color: #222;Â font-size: 16px;Â font-family: 'Poppins',sans-serif;}.space{Â margin-top: 16px;}.show{Â position: absolute;Â right: 13px;Â font-size: 13px;Â font-weight: 700;Â color: #222;Â display: none;Â cursor: pointer;Â font-family: 'Montserrat',sans-serif;}.pass-key:valid ~ .show{Â display: block;}.pass{Â text-align: left;Â margin: 10px 0;}.pass a{Â color: white;Â text-decoration: none;Â font-family: 'Poppins',sans-serif;}.pass:hover a{Â text-decoration: underline;}.field input[type="submit"]{Â background: #3498db;Â border: 1px solid #2691d9;Â color: white;Â font-size: 18px;Â letter-spacing: 1px;Â font-weight: 600;Â cursor: pointer;Â font-family: 'Montserrat',sans-serif;}.field input[type="submit"]:hover{Â background: #2691d9;}.login{Â color: white;Â margin: 20px 0;Â font-family: 'Poppins',sans-serif;}.links{Â display: flex;Â cursor: pointer;Â color: white;Â margin: 0 0 20px 0;}.facebook,.instagram{Â width: 100%;Â height: 45px;Â line-height: 45px;Â margin-left: 10px;}.facebook{Â margin-left: 0;Â background: #4267B2;Â border: 1px solid #3e61a8;}.instagram{Â background: #E1306C;Â border: 1px solid #df2060;}.facebook:hover{Â background: #3e61a8;}.instagram:hover{Â background: #df2060;}.links i{Â font-size: 17px;}i span{Â margin-left: 8px;Â font-weight: 500;Â letter-spacing: 1px;Â font-size: 16px;Â font-family: 'Poppins',sans-serif;}.signup{Â font-size: 15px;Â color: white;Â font-family: 'Poppins',sans-serif;}.signup a{Â color: #3498db;Â text-decoration: none;}.signup a:hover{Â text-decoration: underline;}
Step 3: Adding JavaScript for Toggle Password Visibility
You can add a show/hide password functionality to improve the form’s usability using JavaScript. This will allow users to toggle the visibility of their password, ensuring ease of use.
<script>Â const pass_field = document.querySelector('.pass-key');Â const showBtn = document.querySelector('.show');Â showBtn.addEventListener('click', function(){Â Â if(pass_field.type === "password"){Â Â Â pass_field.type = "text";Â Â Â showBtn.textContent = "HIDE";Â Â Â showBtn.style.color = "#3498db";Â Â }else{Â Â Â pass_field.type = "password";Â Â Â showBtn.textContent = "SHOW";Â Â Â showBtn.style.color = "#222";Â Â }Â });</script>
Step 4: Ensuring Responsiveness
Add media queries for different screen sizes to ensure the login form looks great on mobile devices. This will ensure the form adjusts its size and layout based on the device’s width.
@media (max-width: 600px) {.field input[type="submit"]{Â background: red;Â border: 1px solid #2691d9;Â color: white;Â font-size: 18px;Â letter-spacing: 1px;Â font-weight: 600;Â cursor: pointer;Â font-family: 'Montserrat',sans-serif;}}Want to Check Username Availability on Social Media?If you’re looking to check username availability on various social media platforms, visit NameChkr to find out!
Read Also
- Glassmorphism Login Form in HTML and CSS
Explore the stylish world of glassmorphism as you create a modern login form using HTML and CSS. This guide breaks down the design process step by step.- Toggle Button using HTML, CSS, and JavaScript
Discover how to enhance user interaction by creating a sleek toggle button with HTML, CSS, and JavaScript. This tutorial covers everything from structure to styling.- Responsive Cards in HTML and CSS
Learn how to design eye-catching responsive cards that adapt seamlessly to any device. This guide offers practical tips for achieving stunning layouts.- Build a Google Gemini Chatbot Using HTML, CSS, and JS
Dive into chatbot development by creating a Google Gemini chatbot with HTML, CSS, and JavaScript. This tutorial will help you understand the basics of interactive forms.Conclusion
By following this guide, you now have a beautifully designed Transparent Login Form using HTML, CSS, and JavaScript that’s functional and visually appealing. This login form can easily be customized and integrated into any website, providing a sleek and modern way to handle user authentication.
If you encounter any challenges or want to explore more customization options, feel free to download the complete source code by clicking the button below.