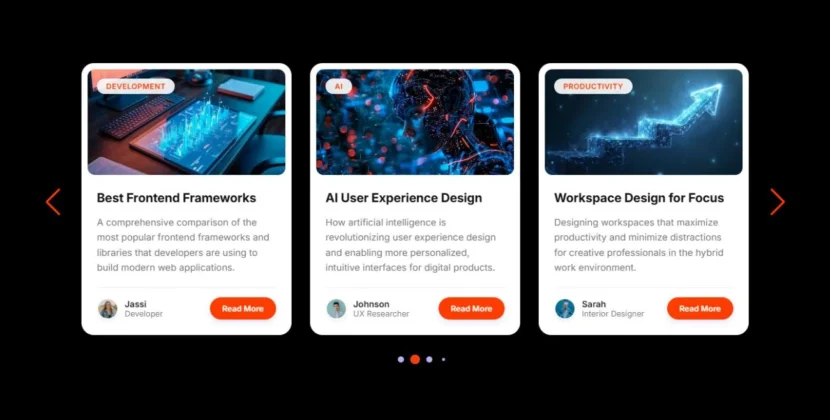
Want to create a cool and sleek vertical card sliding animation for your website with only HTML and CSS? Give a touch of elegance to your website without the clutter of JavaScript! Let’s dive into this comprehensive guide, where we’ll explore how to create a sleek and stylish vertical card slider with smooth animations that require no JavaScript at all. So, let’s experience these smooth animations and modern design, perfect for developers committed to creating fast, adaptable, and SEO-friendly sites.
Whether working on a personal project or building a portfolio, this tutorial will show you how to create a beautiful card slider that performs well across all devices and screens. The best part? It’s entirely built with HTML and CSS, making it easy to implement and maintain.
What is Vertical Card Sliding Animation?
A Vertical Card Sliding Animation is a smooth and modern way to display multiple pieces of content, such as user profiles, testimonials, or products. With this design, several cards slide vertically, one after the other, while maintaining a minimal, clean layout.
This guide will teach you how to build Vertical Card Sliding Animation using only HTML & CSS, focusing on performance and responsiveness. This design helps capture user attention and provides a dynamic display of essential content in a small space.
Why Choose HTML & CSS for Vertical Card Sliding Animation?
There are several reasons to create this animation using only HTML and CSS:
- Performance: A CSS-only solution is much lighter than JavaScript-based animations, meaning faster load times and better performance on mobile and desktop devices.
- SEO Benefits: Since this animation does not rely on JavaScript, it helps maintain clean, crawlable code, improving your website’s search engine optimization (SEO).
- Cross-Browser Compatibility: All modern browsers widely support Pure CSS animations, ensuring a seamless experience for your users.
- Ease of Maintenance: A simpler codebase with only HTML and CSS is more effortless to maintain and modify, making it more adaptable to future changes or enhancements.
Focusing on a Vertical Card Sliding Animation using only HTML and CSS will create a visually appealing design while keeping your website efficient and user-friendly.
Key Features of Vertical Card Sliding Animation
Here are the standout features you’ll learn to create in this tutorial:
- Vertical Sliding Animation: The cards slide up and down smoothly.
- Hover Effect: When you hover over a card, the animation pauses, allowing users to focus on the content.
- Fade-In Effect: The central card remains fully visible, while the surrounding cards fade subtlely, adding to the aesthetic.
- Responsive Design: The card slider will adjust to different screen sizes, ensuring it looks great on mobile, tablet, and desktop devices.
- Minimalist Layout: The design is clean and modern, making it perfect for any website that values simplicity and user interaction.
Step-by-Step Guide to Creating Vertical Card Sliding Animation Using Only HTML & CSS
Let’s break down how to build this Vertical Card Sliding Animation using only HTML & CSS step by step.
Step 1: Setting Up the HTML Structure
The first step is creating the basic HTML structure for your card slider. This will include profile pictures, names, job titles, and a button for interaction. Here’s the initial HTML:
<!DOCTYPE html><!-- Coding By abhikesh - abhikesh.com --><html lang="en"><head>Â <meta charset="UTF-8">Â <meta name="viewport" content="width=device-width, initial-scale=1.0">Â <title>Vertical Card Slider | abhikesh</title>Â <link rel="stylesheet" href="style.css"></head><body>Â <div class="wrapper">Â Â <div class="outer">Â Â Â <div class="card" style="--delay:-1;">Â Â Â Â <div class="content">Â Â Â Â Â <div class="img"><img src="images/img-1.jpg" alt=""></div>Â Â Â Â Â <div class="details">Â Â Â Â Â Â <span class="name">Rahul Kapoor</span>Â Â Â Â Â Â <p>Frontend Developer</p>Â Â Â Â Â </div>Â Â Â Â </div>Â Â Â Â <a href="#">Follow</a>Â Â Â </div>Â Â Â <div class="card" style="--delay:0;">Â Â Â Â <div class="content">Â Â Â Â Â <div class="img"><img src="images/img-2.jpg" alt=""></div>Â Â Â Â Â <div class="details">Â Â Â Â Â Â <span class="name">Romiyo Neil</span>Â Â Â Â Â Â <p>YouTuber & Blogger</p>Â Â Â Â Â </div>Â Â Â Â </div>Â Â Â Â <a href="#">Follow</a>Â Â Â </div>Â Â Â <div class="card" style="--delay:1;">Â Â Â Â <div class="content">Â Â Â Â Â <div class="img"><img src="images/img-3.jpg" alt=""></div>Â Â Â Â Â <div class="details">Â Â Â Â Â Â <span class="name">Jasmine Carter</span>Â Â Â Â Â Â <p>Freelancer & Vlogger</p>Â Â Â Â Â </div>Â Â Â Â </div>Â Â Â Â <a href="#">Follow</a>Â Â Â </div>Â Â Â <div class="card" style="--delay:2;">Â Â Â Â <div class="content">Â Â Â Â Â <div class="img"><img src="images/img-4.jpg" alt=""></div>Â Â Â Â Â <div class="details">Â Â Â Â Â Â <span class="name">Justin Chung</span>Â Â Â Â Â Â <p>Backend Developer</p>Â Â Â Â Â </div>Â Â Â Â </div>Â Â Â Â <a href="#">Follow</a>Â Â Â </div>Â Â Â <div class="card" style="--delay:2;">Â Â Â Â <div class="content">Â Â Â Â Â <div class="img"><img src="images/img-5.jpg" alt=""></div>Â Â Â Â Â <div class="details">Â Â Â Â Â Â <span class="name">Adrina Calvo</span>Â Â Â Â Â Â <p>Teacher & Advertiser</p>Â Â Â Â Â </div>Â Â Â Â </div>Â Â Â Â <a href="#">Follow</a>Â Â Â </div>Â Â </div>Â </div></body></html>
This simple HTML provides the foundation for your Vertical Card Sliding Animation using only HTML & CSS.
Step 2: Applying CSS Styling and Animation
Next, let’s style the card slider using CSS. We’ll use Flexbox for layout, and CSS animations will create the vertical sliding effect.
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@200;300;400;500;600;700&display=swap');*{Â margin: 0;Â padding: 0;Â box-sizing: border-box;Â font-family: "Poppins", sans-serif;}body{Â width: 100%;Â height: 100vh;Â display: flex;Â align-items: center;Â justify-content: center;Â background: linear-gradient(to bottom, #bea2e7 0%, #86b7e7 100%);}.wrapper .outer{Â display: flex;Â align-items: center;Â justify-content: center;}.wrapper .card{Â background: #fff;Â width: 430px;Â display: flex;Â align-items: center;Â padding: 20px;Â opacity: 0;Â pointer-events: none;Â position: absolute;Â justify-content: space-between;Â border-radius: 100px 20px 20px 100px;Â box-shadow: 0px 10px 15px rgba(0,0,0,0.1);Â animation: animate 15s linear infinite;Â animation-delay: calc(3s * var(--delay));}.outer:hover .card{Â animation-play-state: paused;}.wrapper .card:last-child{Â animation-delay: calc(-3s * var(--delay));}@keyframes animate {Â 0%{Â Â opacity: 0;Â Â transform: translateY(100%) scale(0.5);Â }Â 5%, 20%{Â Â opacity: 0.4;Â Â transform: translateY(100%) scale(0.7);Â }Â 25%, 40%{Â Â opacity: 1;Â Â pointer-events: auto;Â Â transform: translateY(0%) scale(1);Â }Â 45%, 60%{Â Â opacity: 0.4;Â Â transform: translateY(-100%) scale(0.7);Â }Â 65%, 100%{Â Â opacity: 0;Â Â transform: translateY(-100%) scale(0.5);Â }}.card .content{Â display: flex;Â align-items: center;}.wrapper .card .img{Â height: 90px;Â width: 90px;Â position: absolute;Â left: -5px;Â background: #fff;Â border-radius: 50%;Â padding: 5px;Â box-shadow: 0px 0px 5px rgba(0,0,0,0.2);}.card .img img{Â height: 100%;Â width: 100%;Â border-radius: 50%;Â object-fit: cover;}.card .details{Â margin-left: 80px;}.details span{Â font-weight: 600;Â font-size: 18px;}.card a{Â text-decoration: none;Â padding: 7px 18px;Â border-radius: 25px;Â color: #fff;Â background: linear-gradient(to bottom, #bea2e7 0%, #86b7e7 100%);Â transition: all 0.3s ease;}.card a:hover{Â transform: scale(0.94);}
This CSS will create the vertical sliding animation that brings the cards in and out of view.
Step 3: Making the Design Responsive with Media Queries
Use media queries for responsive design to ensure that your card slider looks perfect on all devices. This allows the slider to adapt to smaller screens.
@media (max-width: 600px) {Â .card a {Â Â text-decoration: none;Â Â padding: 7px 18px;Â Â border-radius: 25px;Â Â color: red;Â Â background: linear-gradient(to bottom, #bea2e7 0%, #86b7e7 100%);Â Â transition: all 0.3s ease;Â }Â .card a:hover {Â Â transform: scale(0.94);Â }}
Benefits of Using Pure HTML & CSS for Animations
Why should you opt for a solution using only HTML and CSS? Here are some key reasons:
- No JavaScript Dependency: You don’t need to load additional libraries, reducing page weight and load times.
- Improved SEO: Clean HTML and CSS are more search engine friendly than JavaScript-heavy solutions.
- Accessibility: CSS animations are inherently accessible and work across various devices and browsers.
- Faster Development Time: With fewer dependencies, the development process is more rapid, and debugging is simpler.
Utilizing only HTML and CSS for this vertical sliding card animation ensures your design is lightweight, high-performing, and easy to maintain.
Read Also
- Glassmorphism Login Form in HTML and CSS
Explore the stylish world of glassmorphism as you create a modern login form using HTML and CSS. This guide breaks down the design process step by step. - Toggle Button using HTML, CSS, and JavaScript
Discover how to enhance user interaction by creating a sleek toggle button with HTML, CSS, and JavaScript. This tutorial covers everything from structure to styling. - Responsive Cards in HTML and CSS
Learn how to design eye-catching responsive cards that adapt seamlessly to any device. This guide offers practical tips for achieving stunning layouts. - Build a Google Gemini Chatbot Using HTML, CSS, and JS
Dive into chatbot development by creating a Google Gemini chatbot with HTML, CSS, and JavaScript. This tutorial will help you understand the basics of interactive forms.
Conclusion
Creating a Vertical Card Sliding Animation using only HTML and CSS is an excellent way to add an engaging and interactive element to your website. This design is perfect for portfolios, business websites, and personal blogs. Avoiding JavaScript and relying solely on CSS animations enhances user experience and SEO performance while maintaining a simple and clean codebase.
Feel free to customize the design further to suit your project needs. If you encounter any difficulties or want to access the complete source code, don’t hesitate to download it using the link below.
Download the Source Code
Need the complete source code for this Vertical Card Sliding Animation using only HTML & CSS? Click the button below to get the full package and start building your interactive card slider today!