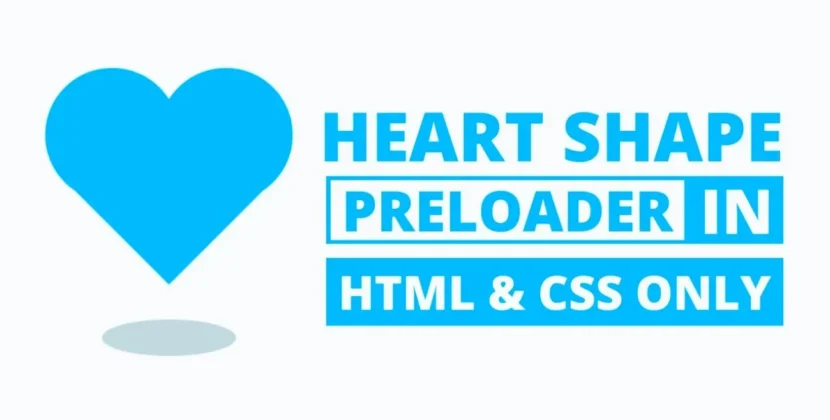
If you’ve ever filled out a form online, you’ve likely noticed the modern, sleek look of Input Label Animations—floating labels that animate when you click inside a text field. This small design feature not only enhances the look of your form but also improves user experience by making it clear which field is currently active.
In this guide, we’ll show you how to create Input Label Animations using HTML and CSS from scratch. We’ll cover everything from the basic structure of the form to the CSS styling that makes the label float when the input is focused. By the end of this article, you’ll have a fully functional, aesthetically pleasing form that can be easily integrated into any website.
Let’s dive right in!
What is Input Label Animation?
Input Label Animations, often called floating label animations, are a popular UI design pattern where the label, usually placed inside the input field, “floats” or moves above the input box when the user interacts with the field. This method is not only visually appealing but also more efficient in terms of space. The input label transitions from being inside the field to hovering above it, providing a clear distinction between inactive and active input fields.
Floating labels are commonly used in modern web forms, helping maintain a clean, minimalist design. Moreover, this animation improves usability by clearly indicating where the user is supposed to input data.
Benefits of Floating Label Animation
Why should you consider implementing input label animation in your forms? Here are a few benefits:
1. Enhanced User Experience
Floating labels offer a more interactive and dynamic experience for users, helping them navigate through forms with ease. It gives visual cues to the user about the input they are currently filling out, which is particularly helpful on forms with multiple fields.
2. Space-Saving Design
With floating labels, there’s no need to place static labels above input fields, saving precious screen real estate. This makes it perfect for mobile-responsive designs where space is limited.
3. Improved Readability
Since the label floats above the input field when the user begins typing, there’s no confusion about what information needs to be entered in each field. This design also makes the form appear cleaner and more intuitive.
4. Modern Aesthetic
Incorporating animations in web forms adds a polished, professional look to the design. It makes your website feel more up-to-date, which can enhance user trust and overall brand perception.
Creating the Basic HTML Structure
Before we get to the fun part of styling the animation, we need to set up a simple HTML structure. Follow these steps:
Step 1: Create an HTML File
First, create a file named index.html. This file will contain the basic HTML structure for your form.
<!DOCTYPE html><!---Coding By abhikesh | www.abhikesh.com---><html lang="en"><head><meta charset="UTF-8"><meta name="viewport" content="width=device-width, initial-scale=1.0"><meta http-equiv="X-UA-Compatible" content="ie=edge"><title>Input Label Animaton | CoderGirl</title><!---Custom CSS File---><link rel="stylesheet" href="style.css"></head><body><div class="input-field"><inputtype="text"requiredspellcheck="false"><label>Enter email</label></div></body></html>
In this structure, we have two input fields: one for the name and one for the email. Each field is wrapped in a div with the class input group for easier styling and alignment.
CSS Styling for Input Label Animation
Now that we have our HTML structure, let’s add some CSS to bring the animation to life. This will be done in a file named style.css.
/* Import Google font - Poppins */ @import url('https://fonts.googleapis.com/css2?family=Poppins:wght@200;300;400;500;600;700&display=swap'); *{ margin: 0; padding: 0; box-sizing: border-box; font-family: 'Poppins', sans-serif; } body{ min-height: 100vh; display: flex; align-items: center; justify-content: center; background-color: #060b23; } .input-field{ position: relative; } .input-field input{ width: 350px; height: 60px; border-radius: 6px; font-size: 18px; padding: 0 15px; border: 2px solid #fff; background: transparent; color: #fff; outline: none; } .input-field label{ position: absolute; top: 50%; left: 15px; transform: translateY(-50%); color: #fff; font-size: 19px; pointer-events: none; transition: 0.3s; } input:focus{ border: 2px solid #18ffff; } input:focus ~ label, input:valid ~ label{ top: 0; left: 15px; font-size: 16px; padding: 0 2px; background: #060b23; }
Step 2: Create a CSS File
Create a file named style.css and add the following CSS code:
Explanation of the CSS
- Form Styling: The form is centered both vertically and horizontally using Flexbox.
- Input Field Styling: Each input field has a basic border and padding, and when focused, the border color changes to blue (#007BFF).
- Label Animation: The label starts inside the input field, aligned vertically to the middle. When the user clicks inside the field or starts typing, the label moves to the top of the input box and shrinks in size.
Understanding the Animation Process
When a user interacts with the input field, CSS transitions are used to smoothly move the label from inside the input field to above it. This movement gives a clear indication of where the label goes as the user focuses on the input field, enhancing the overall user experience.
The transition is primarily controlled by the transform, top, and font-size properties of the label. Additionally, the color of the border changes when the input is focused, which provides a visual cue to users.
The key to this input label animation lies in the following lines of CSS:
input:focus ~ label, input:valid ~ label { top: 0; left: 15px; font-size: 16px; padding: 0 2px; background: #060b23; }
This code tells the browser to move the label upwards when the input field is focused or when valid input is detected. The label floats above the input, stays in this position while the user is typing, and returns to its original position if the input field is cleared. The border color also changes on focus, providing a responsive and elegant experience for the user.
This ensures a clean and dynamic interaction between the user and the form, making the label behavior intuitive and visually appealing.
Additional Customization Options
If you want to further enhance the look and feel of your form, you can try the following:
- Custom Fonts: Experiment with different Google Fonts to give your form a unique look.
- Theme Colors: Change the border color and label colors to match your website’s theme.
- Input Validation: Add some JavaScript to validate user input in real-time, providing feedback when a field is incorrectly filled.
Tips for Optimizing Input Label Animation
To ensure that your floating label animations look great and perform well across all devices, follow these best practices:
- Mobile Responsiveness: Always test your forms on different screen sizes to ensure the labels and input fields scale properly on mobile devices.
- Accessibility: Make sure that your forms are accessible by adding proper aria-label tags and ensuring that the animations are easy to understand for screen reader users.
- Performance: Use lightweight CSS animations to avoid impacting your website’s load time. Avoid using too many CSS animations in one page, as this can slow down the user experience.
Read Also
- Glassmorphism Login Form in HTML and CSS
Explore the stylish world of glassmorphism as you create a modern login form using HTML and CSS. This guide breaks down the design process step by step. - Toggle Button using HTML, CSS, and JavaScript
Discover how to enhance user interaction by creating a sleek toggle button with HTML, CSS, and JavaScript. This tutorial covers everything from structure to styling. - Responsive Cards in HTML and CSS
Learn how to design eye-catching responsive cards that adapt seamlessly to any device. This guide offers practical tips for achieving stunning layouts. - Build a Google Gemini Chatbot Using HTML, CSS, and JS
Dive into chatbot development by creating a Google Gemini chatbot with HTML, CSS, and JavaScript. This tutorial will help you understand the basics of interactive forms.
Conclusion
You’ve now learned how to create a sleek and user-friendly Input Label Animation using HTML and CSS. By integrating this feature into your forms, you’ll enhance both the functionality and aesthetic appeal of your website, making it more engaging for users.
Input label animations are a small but impactful UI/UX feature that can make your forms look modern and professional. Experiment with different styles, colors, and fonts to find the perfect fit for your website.
For further customization, feel free to download the source code below and start tweaking it to suit your needs!
Download Source Code
Click the button below to download the full source code for the Input Label Animation project: