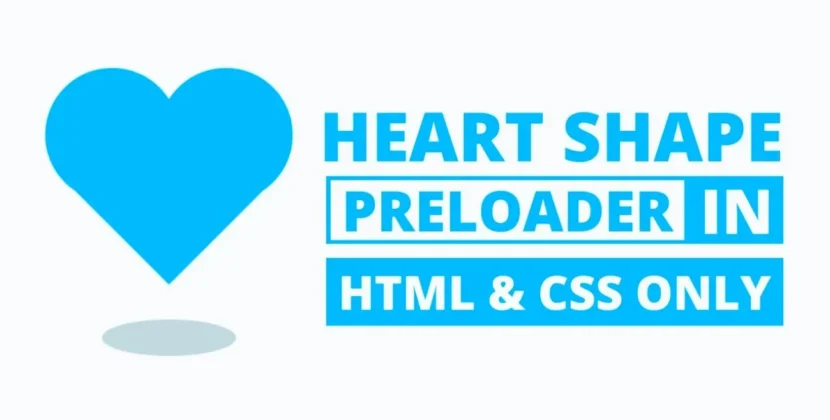
Creating a Basic Login Form is a fundamental skill for any aspiring web developer. If you’ve ever browsed various websites, you’ve likely encountered numerous basic login forms. Have you ever wondered how to design a basic login form using just HTML and CSS? The great news is that it’s entirely possible to build an elegant and functional basic login form using only these two powerful languages.
In this guide, we’ll walk you through the entire process of building a Basic Login Form using HTML and CSS. We’ll begin by establishing the HTML structure, followed by applying CSS styles to enhance its visual appeal. Additionally, we’ll incorporate modern features like Google and Apple login buttons to give it a contemporary touch. Let’s dive right in!
Steps to Build a Basic Login Form Using HTML and CSS
To create your simple login form using only HTML and CSS, follow these straightforward steps:
Step 1: Set Up Your Project
First, create a new folder to house your project files. You can name it anything you like; for example, login-form. Within this folder, create the following files:
- index.html: This file will act as the primary HTML document for your project.
- style.css: This file will contain all your CSS styling code.
- Images Folder: Create an Images folder where you will place logos for Google and Apple, which will be used in the form.
Step 2: Build the HTML Structure
Now, let’s start coding! Open your index.html file and add the necessary HTML markup. This code will lay out the basic structure of your login form using essential HTML elements like <form>, <div>, <label>, and <button>.
<!DOCTYPE html><!-- Source Codes By abhikesh - www.abhikesh.com --><html lang="en"><head>Â <meta charset="UTF-8" />Â <meta name="viewport" content="width=device-width, initial-scale=1.0" />Â <title>Login Form in HTML and CSS | Abhikesh Kumar</title>Â <link rel="stylesheet" href="style.css" /></head><body>Â <div class="login_form">Â Â <!-- Login form container -->Â Â <form action="#">Â Â Â <h3>Log in with</h3>Â Â Â <div class="login_option">Â Â Â Â <!-- Google button -->Â Â Â Â <div class="option">Â Â Â Â Â <a href="#">Â Â Â Â Â Â <img src="logos/google.png" alt="Google" />Â Â Â Â Â Â <span>Google</span>Â Â Â Â Â </a>Â Â Â Â </div>Â Â Â Â <!-- Apple button -->Â Â Â Â <div class="option">Â Â Â Â Â <a href="#">Â Â Â Â Â Â <img src="logos/apple.png" alt="Apple" />Â Â Â Â Â Â <span>Apple</span>Â Â Â Â Â </a>Â Â Â Â </div>Â Â Â </div>Â Â Â <!-- Login option separator -->Â Â Â <p class="separator">Â Â Â Â <span>or</span>Â Â Â </p>Â Â Â <!-- Email input box -->Â Â Â <div class="input_box">Â Â Â Â <label for="email">Email</label>Â Â Â Â <input type="email" id="email" placeholder="Enter email address" required />Â Â Â </div>Â Â Â <!-- Paswwrod input box -->Â Â Â <div class="input_box">Â Â Â Â <div class="password_title">Â Â Â Â Â <label for="password">Password</label>Â Â Â Â Â <a href="#">Forgot Password?</a>Â Â Â Â </div>Â Â Â Â <input type="password" id="password" placeholder="Enter your password" required />Â Â Â </div>Â Â Â Â <!-- Login button -->Â Â Â <button type="submit">Log In</button>Â Â Â <p class="sign_up">Don't have an account? <a href="#">Sign up</a></p>Â Â </form>Â </div></body></html>
Step 3: Style Your Form Using CSS
Next, open your style.css file and add the appropriate CSS code to style your login form. This code will help your form look modern and visually appealing. You can customize it further by experimenting with different colors, fonts, and backgrounds to match your style.
/* Google Fonts Link */@import url('https://fonts.googleapis.com/css2?family=Montserrat:ital,wght@0,100..900;1,100..900&display=swap');/* Resetting default styling and setting font-family */* {Â Â margin: 0;Â Â padding: 0;Â Â box-sizing: border-box;Â Â font-family: "Montserrat", sans-serif;}body {Â Â width: 100%;Â Â min-height: 100vh;Â Â padding: 0 10px;Â Â display: flex;Â Â background: #ff3c00;Â Â justify-content: center;Â Â align-items: center;}/* Login form styling */.login_form {Â Â width: 100%;Â Â max-width: 435px;Â Â background: #fff;Â Â border-radius: 6px;Â Â padding: 41px 30px;Â Â box-shadow: 0 10px 20px rgba(0, 0, 0, 0.15);}.login_form h3 {Â Â font-size: 20px;Â Â text-align: center;}/* Google & Apple button styling */.login_form .login_option {Â Â display: flex;Â Â width: 100%;Â Â justify-content: space-between;Â Â align-items: center;}.login_form .login_option .option {Â Â width: calc(100% / 2 - 12px);}.login_form .login_option .option a {Â Â height: 56px;Â Â display: flex;Â Â justify-content: center;Â Â align-items: center;Â Â gap: 12px;Â Â background: #F8F8FB;Â Â border: 1px solid #DADAF2;Â Â border-radius: 5px;Â Â margin: 34px 0 24px 0;Â Â text-decoration: none;Â Â color: #171645;Â Â font-weight: 500;Â Â transition: 0.2s ease;}.login_form .login_option .option a:hover {Â Â background: #ededf5;Â Â border-color: #ff3c00;}.login_form .login_option .option a img {Â Â max-width: 25px;}.login_form p {Â Â text-align: center;Â Â font-weight: 500;}.login_form .separator {Â Â position: relative;Â Â margin-bottom: 24px;}/* Login option separator styling */.login_form .separator span {Â Â background: #fff;Â Â z-index: 1;Â Â padding: 0 10px;Â Â position: relative;}.login_form .separator::after {Â Â content: '';Â Â position: absolute;Â Â width: 100%;Â Â top: 50%;Â Â left: 0;Â Â height: 1px;Â Â background: #C2C2C2;Â Â display: block;}form .input_box label {Â Â display: block;Â Â font-weight: 500;Â Â margin-bottom: 8px;}/* Input field styling */form .input_box input {Â Â width: 100%;Â Â height: 57px;Â Â border: 1px solid #DADAF2;Â Â border-radius: 5px;Â Â outline: none;Â Â background: #F8F8FB;Â Â font-size: 17px;Â Â padding: 0px 20px;Â Â margin-bottom: 25px;Â Â transition: 0.2s ease;}form .input_box input:focus {Â Â border-color: #ff3c00;}form .input_box .password_title {Â Â display: flex;Â Â justify-content: space-between;Â Â text-align: center;}form .input_box {Â Â position: relative;}a {Â Â text-decoration: none;Â Â color: #ff3c00;Â Â font-weight: 500;}a:hover {Â Â text-decoration: underline;}/* Login button styling */form button {Â Â width: 100%;Â Â height: 56px;Â Â border-radius: 5px;Â Â border: none;Â Â outline: none;Â Â background: #ff3c00;Â Â color: #fff;Â Â font-size: 18px;Â Â font-weight: 500;Â Â text-transform: uppercase;Â Â cursor: pointer;Â Â margin-bottom: 28px;Â Â transition: 0.3s ease;}form button:hover {Â Â background: #ff3c00;}
Step 4: Test Your Login Form
Once you have added the HTML and CSS code, it’s time to see your work in action! Open index.html in your favorite web browser to view your project. If everything is set up correctly, you should see your stylish login form ready to use.
Read Also
- Glassmorphism Login Form in HTML and CSS
Explore the stylish world of glassmorphism as you create a modern login form using HTML and CSS. This guide breaks down the design process step by step. - Toggle Button using HTML, CSS, and JavaScript
Discover how to enhance user interaction by creating a sleek toggle button with HTML, CSS, and JavaScript. This tutorial covers everything from structure to styling. - Responsive Cards in HTML and CSS
Learn how to design eye-catching responsive cards that adapt seamlessly to any device. This guide offers practical tips for achieving stunning layouts. - Build a Google Gemini Chatbot Using HTML, CSS, and JS
Dive into chatbot development by creating a Google Gemini chatbot with HTML, CSS, and JavaScript. This tutorial will help you understand the basics of interactive forms.
Conclusion
Creating a Basic Login Form is a fantastic way for beginners to grasp the fundamentals of HTML and CSS while gaining practical experience in designing and styling web forms. By following the steps outlined in this guide, you’ve successfully built your Basic Login Form from scratch.
To further enhance your web development skills, consider exploring other attractive login and registration forms available online. Many of these examples utilize advanced features that can deepen your understanding of user interactions and enhance your projects.
If you encounter any challenges while building your Basic Login Form or wish to save time, feel free to download the source code for this project using the button below.
Feel free to download the complete source code for this project and start building!