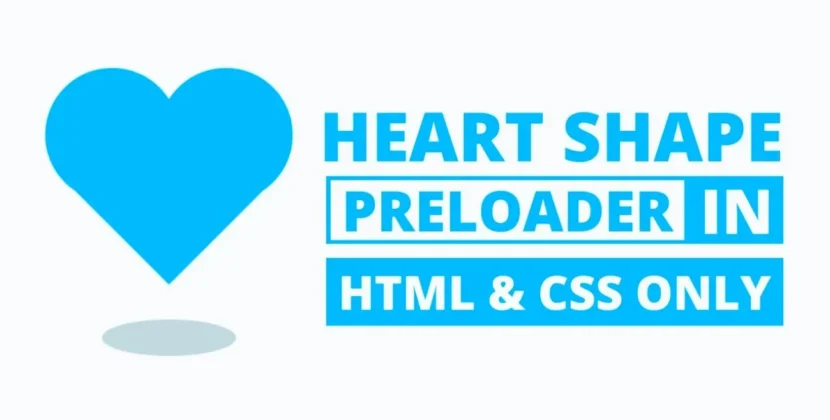
The sleek and familiar design of Netflix’s login page is one of the most well-known on the web. Have you ever thought to recreate this eye-catching interface, then you’re in the right place. In this guide, I’ll show you how to build a Netflix-inspired login page using HTML and CSS. Whether you’re a beginner or an intermediate developer, this project will help you improve your front-end skills while mimickingthe modern, user-friendly design of Netflix.
Why Build a Netflix-inspired Login Page?
For developers, recreating real-world projects is a fantastic way to practice. The Netflix-inspired login page is more than just an ordinary sign-in form—it has a clean, responsive design that works seamlessly across devices. By building this, you’ll learn how to position elements, style forms, and make a page responsive, all while mimicking a global platform’s aesthetic.
Let’s jump into the step-by-step process of building a Netflix-inspired login page.
Steps to Create a Netflix-inspired Login Page in HTML and CSS
1. Create Your Project Folder: Create a folder for your project. Inside this folder, create two files:
- index.html (for HTML structure)
- style.css (for styling)
You can also create an “Images” folder to add the Netflix logo or background images to the project.
2. Build the HTML Structure: In the index.html file, you’ll use semantic HTML to create the layout. Add the necessary elements such as a navigation bar, heading, form, input fields for username and password, and a “Sign In” button. Here’s an example of how your HTML might look:
<!DOCTYPE html><!-- Coding By Abhikesh - www.abhikesh.com --><html lang="en"><head>  <meta charset="UTF-8">  <meta name="viewport" content="width=device-width, initial-scale=1.0">  <title>Netflix Login Page | Abhikesh Kumar</title>  <link rel="stylesheet" href="style.css"></head><body>  <nav>    <a href="#"><img src="images/logo.svg" alt="logo"></a>  </nav>  <div class="form-wrapper">    <h2>Sign In</h2>    <form action="#">      <div class="form-control">        <input type="text" required>        <label>Email or phone number</label>      </div>      <div class="form-control">        <input type="password" required>        <label>Password</label>      </div>      <button type="submit">Sign In</button>      <divclass="form-help">        <div class="remember-me">          <input type="checkbox" id="remember-me">          <label for="remember-me">Remember me</label>        </div>        <a href="#">Need help?</a>      </div>    </form>    <p>New to Netflix? <a href="#">Sign up now</a></p>    <small>      This page is protected by Google reCAPTCHA to ensure you're not a bot.      <a href="#">Learn more.</a>    </small>  </div></body></html>
3. Style the Page with CSS: In your style.css file, style the elements to match Netflix’s minimalist look. You’ll focus on fonts, spacing, and responsiveness to ensure the page looks good on all devices. Here’s some basic CSS for the login page:
@import url("https://fonts.googleapis.com/css2?family=Roboto:wght@400;500;600;700&display=swap");* {Â Â margin: 0;Â Â padding: 0;Â Â box-sizing: border-box;Â Â font-family: 'Roboto', sans-serif;}body {Â Â background: #000;}body::before {Â Â content: "";Â Â position: absolute;Â Â left: 0;Â Â top: 0;Â Â opacity: 0.5;Â Â width: 100%;Â Â height: 100%;Â Â background: url("images/hero-img.jpg");Â Â background-position: center;}nav {Â Â position: fixed;Â Â padding: 25px 60px;Â Â z-index: 1;}nav a img {Â Â width: 167px;}.form-wrapper {Â Â position: absolute;Â Â left: 50%;Â Â top: 50%;Â Â border-radius: 4px;Â Â padding: 70px;Â Â width: 450px;Â Â transform: translate(-50%, -50%);Â Â background: rgba(0, 0, 0, .75);}.form-wrapper h2 {Â Â color: #fff;Â Â font-size: 2rem;}.form-wrapper form {Â Â margin: 25px 0 65px;}form .form-control {Â Â height: 50px;Â Â position: relative;Â Â margin-bottom: 16px;}.form-control input {Â Â height: 100%;Â Â width: 100%;Â Â background: #333;Â Â border: none;Â Â outline: none;Â Â border-radius: 4px;Â Â color: #fff;Â Â font-size: 1rem;Â Â padding: 0 20px;}.form-control input:is(:focus, :valid) {Â Â background: #444;Â Â padding: 16px 20px 0;}.form-control label {Â Â position: absolute;Â Â left: 20px;Â Â top: 50%;Â Â transform: translateY(-50%);Â Â font-size: 1rem;Â Â pointer-events: none;Â Â color: #8c8c8c;Â Â transition: all 0.1s ease;}.form-control input:is(:focus, :valid)~label {Â Â font-size: 0.75rem;Â Â transform: translateY(-130%);}form button {Â Â width: 100%;Â Â padding: 16px 0;Â Â font-size: 1rem;Â Â background: #e50914;Â Â color: #fff;Â Â font-weight: 500;Â Â border-radius: 4px;Â Â border: none;Â Â outline: none;Â Â margin: 25px 0 10px;Â Â cursor: pointer;Â Â transition: 0.1s ease;}form button:hover {Â Â background: #c40812;}.form-wrapper a {Â Â text-decoration: none;}.form-wrapper a:hover {Â Â text-decoration: underline;}.form-wrapper :where(label, p, small, a) {Â Â color: #b3b3b3;}form .form-help {Â Â display: flex;Â Â justify-content: space-between;}form .remember-me {Â Â display: flex;}form .remember-me input {Â Â margin-right: 5px;Â Â accent-color: #b3b3b3;}form .form-help :where(label, a) {Â Â font-size: 0.9rem;}.form-wrapper p a {Â Â color: #fff;}.form-wrapper small {Â Â display: block;Â Â margin-top: 15px;Â Â color: #b3b3b3;}.form-wrapper small a {Â Â color: #0071eb;}@media (max-width: 740px) {Â Â body::before {Â Â Â Â display: none;Â Â }Â Â nav, .form-wrapper {Â Â Â Â padding: 20px;Â Â }Â Â nav a img {Â Â Â Â width: 140px;Â Â }Â Â .form-wrapper {Â Â Â Â width: 100%;Â Â Â Â top: 43%;Â Â }Â Â .form-wrapper form {Â Â Â Â margin: 25px 0 40px;Â Â }}
Key Learning Points
- Form Design: You’ll understand how to create a clean and user-friendly login form, focusing on accessibility and responsiveness.
- CSS Flexbox: You’ll use Flexbox to center the form on the screen, making it responsive and ensuring it looks good on all devices.
- Styling and Design: You’ll match the Netflix aesthetic, learning how to incorporate background images, colors, and minimalistic design elements that enhance the user experience.
Read Also
- Glassmorphism Login Form in HTML and CSS
Explore the stylish world of glassmorphism as you create a modern login form using HTML and CSS. This guide breaks down the design process step by step. - Toggle Button using HTML, CSS, and JavaScript
Discover how to enhance user interaction by creating a sleek toggle button with HTML, CSS, and JavaScript. This tutorial covers everything from structure to styling. - Responsive Cards in HTML and CSS
Learn how to design eye-catching responsive cards that adapt seamlessly to any device. This guide offers practical tips for achieving stunning layouts. - Build a Google Gemini Chatbot Using HTML, CSS, and JS
Dive into chatbot development by creating a Google Gemini chatbot with HTML, CSS, and JavaScript. This tutorial will help you understand the basics of interactive forms.
Conclusion and Final Words
Building a Netflix-inspired login page using only HTML and CSS is a great project for both beginners and seasoned developers. It allows you to explore crucial aspects of web development like form creation, styling, and making responsive designs. Projects like this help hone your front-end development skills, improve your understanding of CSS, and build your portfolio.
Once you’ve mastered this, you can take it a step further by adding additional features like floating labels, password toggle options, or even basic JavaScript form validation to make your project more dynamic.
If you encounter any issues while building your Netflix login page, don’t worry! You can download the source code for this project by clicking the “Download” button below.
Download Source Code
Ready to get started? Click the button below to download the source code for the Netflix login page and start your project today.
Well-commented code and step-by-step explanation. Made implementation very easy!
I’ve followed your blog for a while—this is one of your best tutorials yet.