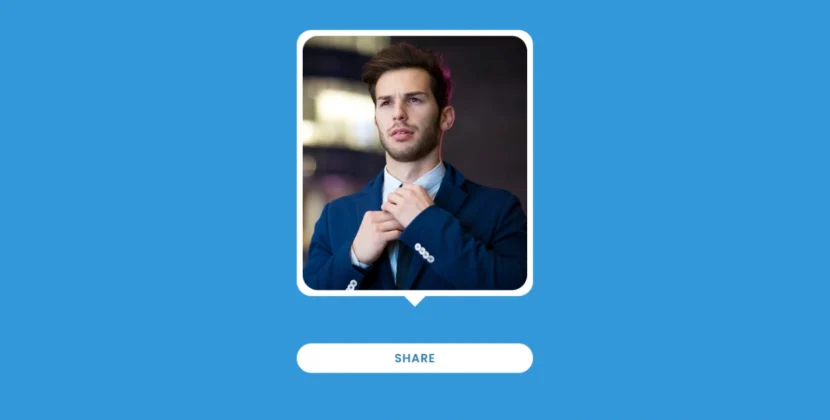
Hello friends, In this blog post, you will learn how to build a Working Contact Form using PHP. This form will use HTML, CSS, JavaScript, and PHP. I previously demonstrated how to send emails using PHP on Localhost; now, let’s use that knowledge to make an accessible PHP contact form!
Contact Forms are an integral website component, enabling visitors to communicate directly with its owner. A Contact Form may include fields for visitors to submit information like their name, email address, subject of message, etc. You can customize its fields according to your website requirements.
As can be seen from the image, this project [Contact form in PHP] features a contact page with dynamic status text that responds to the form’s status and tells users if their message was sent successfully or not. Furthermore, this form has been fully validated, so users cannot submit messages unless they enter valid message text and email address data; moreover, it comes complete with a video tutorial or demo for easy learning purposes.
Read through this blog to fully understand how this contact form was built using PHP. Unlike a regular contact form, which refreshes when the message has been sent or failed, my contact form utilizes JavaScript Ajax technology instead. It sends all data (name, email, phone, web address, and message) directly from Ajax back to PHP for processing.
First, I verified that the user had entered a valid email address and message, as this information is required by law. I then sent this data using PHP’s mail() function.
If the message could not be delivered because of an invalid email address, an empty message field, or network issues, I sent a notification detailing why. This information was then displayed using JavaScript on the contact form.
Check out this:
- Login/Registration Form in HTML, CSS and JavaScript
- Testimonial Slider in HTML CSS & Js | SwiperJS
- Cookie Consent Box for HTML CSS and JavaScript
- Glassmorphism Login Form in HTML and CSS
Create a functional contact form in PHP [Source Codes]
Create this project [Contact form in PHP]. First, four files, HTML, CSS, and JavaScript, will be created. Copy and paste the codes below after creating these files into each of them – after making all four, you may download its source code, which consists of both PHP and JavaScript code.
Create an HTML file named index.html, copy and paste the provided codes into it, and save it as an .html file.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Contact Form in PHP</title> <link rel="stylesheet" href="style.css"> <link rel="stylesheet" href="https://fonts.googleapis.com/icon?family=Material+Icons"> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.3/css/all.min.css"/> </head> <body> <div class="wrapper"> <header>Send us a Message</header> <form action="#"> <div class="dbl-field"> <div class="field"> <input type="text" name="name" placeholder="Enter your name"> <i class='fas fa-user'></i> </div> <div class="field"> <input type="text" name="email" placeholder="Enter your email"> <i class='fas fa-envelope'></i> </div> </div> <div class="dbl-field"> <div class="field"> <input type="text" name="phone" placeholder="Enter your phone"> <i class='fas fa-phone-alt'></i> </div> <div class="field"> <input type="text" name="website" placeholder="Enter your website"> <i class='fas fa-globe'></i> </div> </div> <div class="message"> <textarea placeholder="Write your message" name="message"></textarea> <i class="material-icons">message</i> </div> <div class="button-area"> <button type="submit">Send Message</button> <span></span> </div> </form> </div> <script src="script.js"></script> </body> </html>
Create a CSS Style Sheet named style.css and copy and paste all your codes. For best results, give this file the extension .css.
/* Import Google font - Poppins */ @import url('https://fonts.googleapis.com/css2?family=Poppins:wght@400;500;600&display=swap'); *{ margin: 0; padding: 0; box-sizing: border-box; font-family: "Poppins", sans-serif; } body{ display: flex; padding: 0 10px; min-height: 100vh; background: #0D6EFD; align-items: center; justify-content: center; } ::selection{ color: #fff; background: #0D6EFD; } .wrapper{ width: 715px; background: #fff; border-radius: 5px; box-shadow: 10px 10px 10px rgba(0,0,0,0.05); } .wrapper header{ font-size: 22px; font-weight: 600; padding: 20px 30px; border-bottom: 1px solid #ccc; } .wrapper form{ margin: 35px 30px; } .wrapper form.disabled{ pointer-events: none; opacity: 0.7; } form .dbl-field{ display: flex; margin-bottom: 25px; justify-content: space-between; } .dbl-field .field{ height: 50px; display: flex; position: relative; width: calc(100% / 2 - 13px); } .wrapper form i{ position: absolute; top: 50%; left: 18px; color: #ccc; font-size: 17px; pointer-events: none; transform: translateY(-50%); } form .field input, form .message textarea{ width: 100%; height: 100%; outline: none; padding: 0 18px 0 48px; font-size: 16px; border-radius: 5px; border: 1px solid #ccc; } .field input::placeholder, .message textarea::placeholder{ color: #ccc; } .field input:focus, .message textarea:focus{ padding-left: 47px; border: 2px solid #0D6EFD; } .field input:focus ~ i, .message textarea:focus ~ i{ color: #0D6EFD; } form .message{ position: relative; } form .message i{ top: 30px; font-size: 20px; } form .message textarea{ min-height: 130px; max-height: 230px; max-width: 100%; min-width: 100%; padding: 15px 20px 0 48px; } form .message textarea::-webkit-scrollbar{ width: 0px; } .message textarea:focus{ padding-top: 14px; } form .button-area{ margin: 25px 0; display: flex; align-items: center; } .button-area button{ color: #fff; border: none; outline: none; font-size: 18px; cursor: pointer; border-radius: 5px; padding: 13px 25px; background: #0D6EFD; transition: background 0.3s ease; } .button-area button:hover{ background: #025ce3; } .button-area span{ font-size: 17px; margin-left: 30px; display: none; } @media (max-width: 600px){ .wrapper header{ text-align: center; } .wrapper form{ margin: 35px 20px; } form .dbl-field{ flex-direction: column; margin-bottom: 0px; } form .dbl-field .field{ width: 100%; height: 45px; margin-bottom: 20px; } form .message textarea{ resize: none; } form .button-area{ margin-top: 20px; flex-direction: column; } .button-area button{ width: 100%; padding: 11px 0; font-size: 16px; } .button-area span{ margin: 20px 0 0; text-align: center; } }
Create a JavaScript file called script.js, copy-paste the codes, and save it as an actual.js file.
//Contact Form in PHP const form = document.querySelector("form"), statusTxt = form.querySelector(".button-area span"); form.onsubmit = (e)=>{ e.preventDefault(); statusTxt.style.color = "#0D6EFD"; statusTxt.style.display = "block"; statusTxt.innerText = "Sending your message..."; form.classList.add("disabled"); let xhr = new XMLHttpRequest(); xhr.open("POST", "message.php", true); xhr.onload = ()=>{ if(xhr.readyState == 4 && xhr.status == 200){ let response = xhr.response; if(response.indexOf("required") != -1 || response.indexOf("valid") != -1 || response.indexOf("failed") != -1){ statusTxt.style.color = "red"; }else{ form.reset(); setTimeout(()=>{ statusTxt.style.display = "none"; }, 3000); } statusTxt.innerText = response; form.classList.remove("disabled"); } } let formData = new FormData(form); xhr.send(formData); }
Create a PHP message.php file and copy and paste its codes. After doing this, please enter your email address as the $receiver variable to ensure messages reach their destination.
//Contact Form in PHP <?php $name = htmlspecialchars($_POST['name']); $email = htmlspecialchars($_POST['email']); $phone = htmlspecialchars($_POST['phone']); $website = htmlspecialchars($_POST['website']); $message = htmlspecialchars($_POST['message']); if(!empty($email) && !empty($message)){ if(filter_var($email, FILTER_VALIDATE_EMAIL)){ $receiver = "receiver_email_address"; //enter that email address where you want to receive all messages $subject = "From: $name <$email>"; $body = "Name: $name\nEmail: $email\nPhone: $phone\nWebsite: $website\n\nMessage:\n$message\n\nRegards,\n$name"; $sender = "From: $email"; if(mail($receiver, $subject, $body, $sender)){ echo "Your message has been sent"; }else{ echo "Sorry, failed to send your message!"; } }else{ echo "Enter a valid email address!"; } }else{ echo "Email and message field is required!"; } ?>
Your PHP Contact Form is complete! If there are any errors/problems, download the source code files; they’re free. However, it won’t function on localhost; upload it and the .zip files instead to make it work online.
Launch message.php on your computer to upload the file. In this file, $receiver can be set as the email address that should receive messages. When done, save and close out this file before zipping it up and uploading it to web hosting, where it will extract itself. We are ready to accept visitor messages using the PHP Contact Form!
Fantastic tutorial! Your step-by-step guide to building a functional contact form in PHP was incredibly helpful. The sections on form validation and email handling were particularly well-explained. This guide will be a great asset for anyone looking to create robust contact forms. Thanks for the clear and practical instructions!
Excellent guide! Your step-by-step approach to building a functional contact form in PHP was incredibly clear and practical. The explanations on handling form submissions and validation were especially helpful. This tutorial is a fantastic resource for anyone looking to improve their PHP skills and create effective contact forms. Thank you for sharing such valuable insights!
Great tutorial! Your guide on building a functional contact form in PHP was very thorough and easy to understand. The sections on form validation and email handling were particularly useful. This resource will definitely help me enhance my PHP skills and create effective contact forms. Thanks for the detailed and practical advice!
Great tutorial! Your explanation of how to build a functional contact form in PHP was very clear and practical. I found the sections on form validation and email handling especially useful. This guide will definitely help me with my PHP projects. Thanks for the detailed and easy-to-follow instructions!
Excellent tutorial! Your step-by-step approach to building a functional contact form in PHP was very clear and informative. The tips on validation and email handling were particularly useful. This guide is a fantastic resource for anyone looking to enhance their PHP skills. Thanks for the detailed and practical insights!
Wonderful tutorial! Your guide to building a functional contact form in PHP was both comprehensive and easy to follow. The explanations on validation and handling form submissions were especially helpful. This resource will be invaluable for my PHP projects. Thanks for sharing such detailed and practical insights!
Fantastic guide! Your instructions on building a functional contact form in PHP were clear and detailed. The sections on form validation and email handling were particularly useful. This tutorial is a great resource for enhancing PHP skills and building effective contact forms. Thanks for the well-explained and practical advice!
Fantastic tutorial! Your guide to building a functional contact form in PHP was incredibly thorough and easy to follow. The sections on validation and email handling were especially helpful. This resource will be invaluable for my web development projects. Thanks for the detailed and practical insights!
Great tutorial! The step-by-step approach to building a functional contact form in PHP was very informative and easy to understand. The sections on validation and email handling were particularly useful. This guide is perfect for anyone looking to enhance their PHP skills. Thanks for the comprehensive and practical advice!
Fantastic tutorial! Your detailed guide on building a functional contact form in PHP was very informative and easy to follow. The sections on form validation and handling email submissions were particularly useful. This will be a great addition to my PHP skill set. Thanks for sharing such a practical and well-explained resource!
Excellent tutorial! Your step-by-step guide to building a functional contact form in PHP was incredibly clear and thorough. I found the sections on form validation and email handling particularly useful. This will definitely help me with my PHP projects. Thanks for providing such a practical and insightful resource!
Fantastic tutorial! The step-by-step instructions for building a functional contact form in PHP were clear and very useful. I particularly appreciated the sections on form validation and email handling. This guide will be a great asset for my PHP development projects. Thanks for sharing such a practical and detailed resource!
Wonderful tutorial! The step-by-step instructions for building a functional contact form in PHP were clear and comprehensive. I found the sections on form validation and handling submissions especially useful. This guide will definitely enhance my PHP skills. Thanks for providing such a detailed and practical resource!”
Excellent tutorial! The step-by-step guide to building a functional contact form in PHP was incredibly helpful. I particularly appreciated the explanations on form validation and handling submissions. This guide will be a great addition to my development toolkit. Thanks for providing such a clear and practical resource!
This guide is fantastic! The step-by-step instructions for building a functional contact form in PHP were clear and thorough. I especially appreciated the sections on form validation and handling submissions. This will be extremely useful for my web projects. Thanks for providing such a detailed and practical tutorial!
This tutorial is incredibly useful! The detailed guide on building a functional contact form in PHP was clear and easy to follow. I especially appreciated the sections on handling form submissions and validations. This will be a great addition to my development toolkit. Thanks for the comprehensive guide!
This is a fantastic tutorial! The guide on building a functional contact form in PHP was very clear and comprehensive. I especially appreciated the sections on handling form submissions and validation. This will be incredibly useful for my projects. Thanks for the detailed instructions!
This tutorial is fantastic for anyone looking to build a contact form in PHP! The step-by-step instructions were clear and easy to follow. I especially appreciated the focus on form validation and handling submissions. This guide will definitely help improve the functionality of my website. Thanks for the great insights!
This is a fantastic guide for building a contact form in PHP! The instructions are clear and straightforward, making it easy to follow along. I especially appreciated the tips on handling form submissions and validation. This will definitely help in enhancing the functionality of my website. Thanks for sharing!
Master the essentials of creating a fully functional contact form using PHP. This guide will take you through the steps of form creation, validation, and email handling, helping you integrate a seamless contact feature into your website. Perfect for beginners and those looking to enhance their PHP skills!
Looking to create a fully functional contact form in PHP? This tutorial guides you through the step-by-step process of building a secure, reliable form for your website, complete with email integration, validation, and more. Perfect for beginners and experienced developers alike!
This tutorial was spot on! The way you explained the process of building a functional contact form in PHP made it so easy to understand. The form validation and email handling tips were especially useful. Thank you for breaking it down in such a simple and practical way!